jQuery parent() and parents() 方法返回 DOM 祖先的元素。它在 DOM 中向上遍历以查找祖先。
在本文中,我们将了解parent()和parents()方法之间的区别。
parent() 方法:parent() 方法仅遍历 DOM 之前的一层,并使用 jQuery 返回所选元素的直接父元素或最近的第一个祖先元素。
用法:
$('selector').parent();
示例1:
HTML
<!DOCTYPE html>
(ancestor-6 )
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<!-- Including jQuery -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"
integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4="
crossorigin="anonymous">
</script>
<style>
h1 {
color: #006600;
}
body {
text-align: center;
}
div {
text-align: center;
padding: 5px;
border: 2px solid black;
border-radius: 5px;
margin: 5px;
}
p{
border: 2px solid black;
margin: 2px;
padding: 5px;
background-color: white;
}
/* The class that turns the div's
background colour to red */
.bg-blue {
background-color: blue;
}
</style>
</head>
<body>
<h1>Geeks For Geeks(ancestor-5)</h1>
<div>
DIV-1(ancestor-4)
<div>
DIV-2(ancestor-3)
<div>
DIV-3(ancestor-2)
<div>
DIV-4 Direct parent of p(ancestor-1)
<p id= 'btn'>
This is geeks for geeks(Click
Me to find direct parent)
</p>
</div>
</div>
</div>
</div>
<script>
$('p').click(function(){
$('p').parent().addClass('bg-blue');
});
</script>
</body>
</html>
输出:
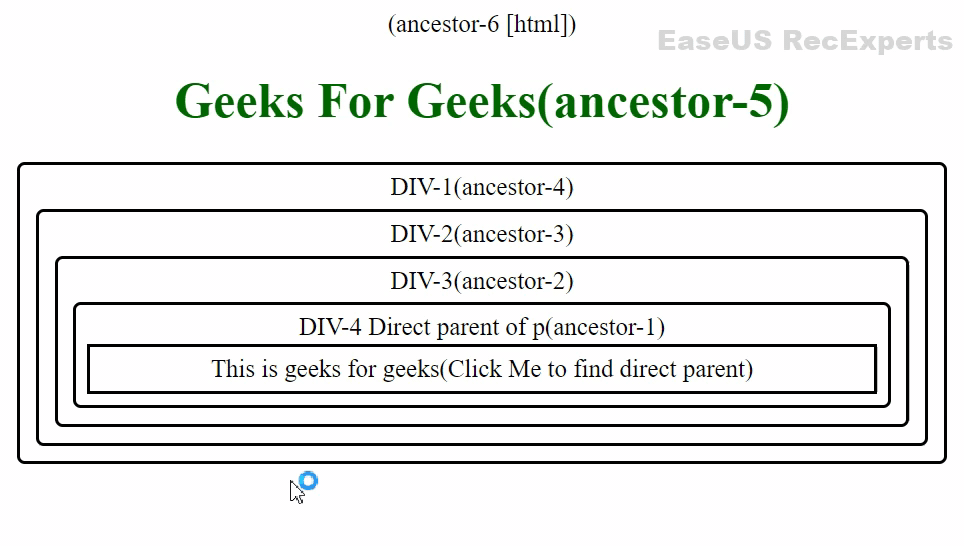
直系父母
如果我们观察到这一点,类‘bg-blue‘ 添加到的直接父级 p那是DIV-4并将背景颜色更改为蓝色 p有白色背景颜色,它保持白色。
示例 2:以下代码还以绿色显示子元素的父元素。
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Geeks for Geeks</title>
<!-- Including jQuery -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"
letegrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4="
crossorigin="anonymous"></script>
<style>
h2 {
color: #006600;
}
button {
color: white;
background-color: #006600;
width: 100px;
height: 30px;
}
#sublist2 {
color: red;
}
</style>
</head>
<body>
<h2>GeeksforGeeks</h2>
<div>
<ul id="list1">
<li>
GrandParent
<ol id="sublist1">
<li>one</li>
<li>two</li>
<li>three</li>
</ol>
</li>
<li>
Parent
<ol>
<li>three</li>
<li>four</li>
<li>five</li>
<ol id="sublist2">
<li> Child</li>
<li>six</li>
<li>seven</li>
<li>eight</li>
</ol>
</ol>
</li>
</ul>
</div>
<script>
$('ol#sublist2').parent().css('color', 'green');
</script>
</body>
</html>
输出:绿色有序列表是子元素的父元素。
parents() 方法:parents() 方法遍历 DOM 中高于所选元素的所有级别元素,并使用 jQuery 返回所选元素的祖先元素。
用法:
$('selector').parents();
示例 1:
HTML
<!DOCTYPE html>
(ancestor-6 )
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<!-- Including jQuery -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"
integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4="
crossorigin="anonymous">
</script>
<style>
h1 {
color: #006600;
}
body {
text-align: center;
}
div {
text-align: center;
padding: 5px;
border: 2px solid black;
border-radius: 5px;
margin: 5px;
}
p{
border: 2px solid black;
margin: 2px;
padding: 5px;
background-color: white;
}
/* The class that turns the div's background colour to red */
.bg-blue {
background-color: blue;
}
</style>
</head>
<body>
<h1>Geeks For Geeks(ancestor-5)</h1>
<div>
DIV-1(ancestor-4)
<div>
DIV-2(ancestor-3)
<div>
DIV-3(ancestor-2)
<div>
DIV-4 Direct parent of p(ancestor-1)
<p id= 'btn'>
This is geeks for geeks(Click Me to find all
ancestors of p tag)
</p>
</div>
</div>
</div>
</div>
<script>
$('p').click(function()
{
$('p').parents().addClass('bg-blue');
});
</script>
</body>
</html>
输出:
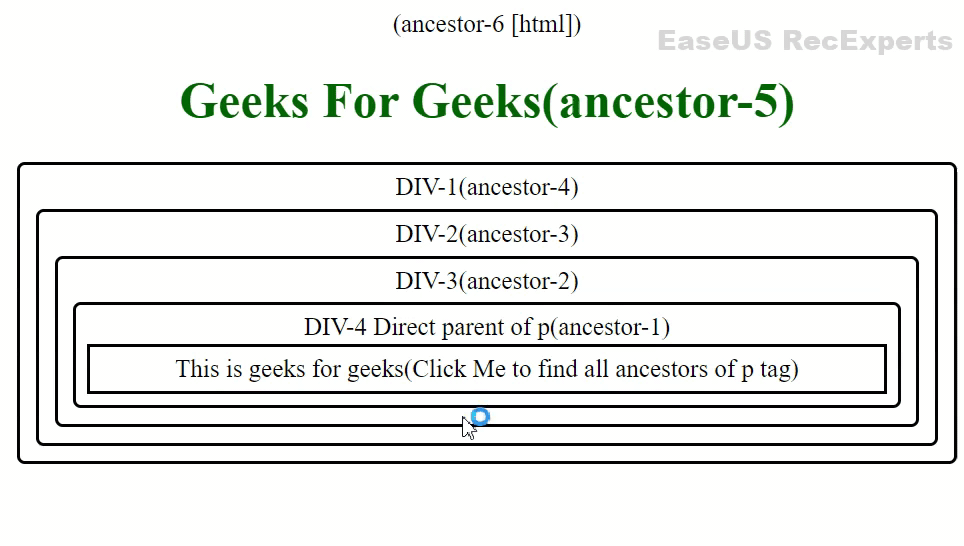
所有的祖先
如果我们观察到这一点,类‘bg-blue‘被添加到所有的祖先中p那是DIV-4、DIV-3、DIV-2、DIV-1、h1、HTML标记并将背景颜色更改为蓝色,因为p有白色背景颜色,它保持白色。
示例 2:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<!-- Including jQuery -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"
integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4="
crossorigin="anonymous">
</script>
<style>
h2 {
color: #006600;
}
button {
color: white;
background-color: #006600;
width: 100px;
height: 30px;
}
#sublist2 {
color: red;
}
</style>
</head>
<body>
<h2>GeeksforGeeks</h2>
<div>
<ul id="list1">
<li>
GrandParent
<ol id="sublist1">
<li>one</li>
<li>two</li>
<li>three</li>
</ol>
</li>
<li>
Parent
<ol>
<li>three</li>
<li>four</li>
<li>five</li>
<ol id="sublist2">
<li> Child</li>
<li>six</li>
<li>seven</li>
<li>eight</li>
</ol>
</ol>
</li>
</ul>
</div>
<script>
$('ol#sublist2').parents().css('color', 'green');
</script>
</body>
</html>
输出:所有green-colored 有序列表都是子元素的父元素。
parent() 和 parents() 方法之间的区别:
parent()方法 | parents()方法 |
它只向上遍历一层 在所选元素的 DOM 中。 |
它遍历 DOM 中的所有级别 所选元素直到根,即 HTML 标记。 |
它只返回一个元素 直接父母。 |
它返回所有祖先元素 选定的元素 |
相关用法
- jQuery param()用法及代码示例
- jQuery prop()和attr()的区别用法及代码示例
- jQuery position()和offset()的区别用法及代码示例
- jQuery event.preventDefault()用法及代码示例
- jQuery :button用法及代码示例
- jQuery :checkbox用法及代码示例
- jQuery :checked用法及代码示例
- jQuery :contains()用法及代码示例
- jQuery :disabled用法及代码示例
- jQuery :empty用法及代码示例
- jQuery :enabled用法及代码示例
- jQuery :even用法及代码示例
- jQuery :file用法及代码示例
- jQuery :first-child用法及代码示例
- jQuery :first-of-type用法及代码示例
- jQuery :first用法及代码示例
- jQuery :focus用法及代码示例
- jQuery :gt()用法及代码示例
- jQuery :header用法及代码示例
- jQuery :hidden用法及代码示例
- jQuery :image用法及代码示例
- jQuery :input用法及代码示例
- jQuery :lang()用法及代码示例
- jQuery :last-child用法及代码示例
- jQuery :last-of-type用法及代码示例
注:本文由纯净天空筛选整理自lokeshpotta20大神的英文原创作品 What is the difference between parent() and parents() methods in jQuery ?。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。