在本文中,我们将看到 Pygame 和 Pyglet 游戏库之间的区别。
什么是 Pyglet?
皮格莱特 是 Python 的跨平台窗口和媒体库,旨在开发游戏和其他视觉效果丰富的应用程序。它支持窗口、UI 事件处理、游戏手柄、OpenGL 图形、图像和视频加载以及声音和音乐播放。 Pyglet 可在 Windows、OS X 和 Linux 上运行。 Pyglet 完全用纯 Python 编写,并使用标准库的类型模块与系统库交互。您可以修改代码库或做出贡献,而无需编译或了解其他语言。虽然 Pyglet 是纯 Python,但它提供了出色的批处理和 GPU 渲染性能。您可以使用 thing 模块轻松绘制数千个精灵或动画。
特征:
- 没有外部依赖项或安装要求,Pyglet 除了 Python 之外不需要任何东西。
- Pyglet 支持多种 platform-native 窗口和 multi-monitor 全屏游戏设置。
- 下载几乎任何格式的图像、声音、音乐和视频。
- Pyglet 是在 BSD 开源许可证下发布的,允许您将其用于商业和其他开源项目,几乎没有任何限制。
安装
pip install pyglet
什么是 Pygame?
pygame 是一组用于创建视频游戏的跨平台 Python 模块。它由设计用于 Python 编程语言的计算机图像和声音库组成。 Pygame 适合构建可以封装在独立可执行文件中的客户端应用程序。 Pygame 是专为视频游戏设计而创建的跨平台 Python 软件。它还包括可用于增强design-time游戏玩法的图形、图像和声音,游戏开发包括数学、逻辑、物理、人工智能等等,它可以非常有趣。它包含各种可处理图像和声音并可以创建游戏图形的库。它简化了整个游戏设计过程,使初学者的游戏开发变得更加容易。
特征:
- 易于编写,它没有数十万行代码来处理您无论如何都不会使用的东西。核心保持简单,GUI 库和效果等额外的东西是在 Pygame 之外单独开发的。
- 所有函数的使用并不需要使用 GUI。如果您只想处理图像、获取操纵杆输入或播放声音,则可以从命令行使用 Pygame。
- 对报告的错误快速响应, 如今,错误报告非常罕见,因为其中许多已经得到修复。
- 对代码的更多控制,这使您在使用其他库和不同类型的程序时拥有更多控制。
安装
pip install pygame
Pyglet 和 Pygame 之间的差异表:
Pyglet |
Pygame |
---|---|
Pyglet is much faster as compared to the Pygame |
Pygame is slower as compared with Pyglet |
Pyglet uses OpenGL |
Pygame uses SDL libraries and does not require OpenGL. |
It is rich with GUI elements. |
GUI using Pygame is not compatible. |
3D projects are supported in Pyglet |
Only 2D projects are Supported. |
Pyglet support Music, video, and image in all format |
Pygame 支持几种格式作为组件 |
No external installation requirements. |
Few module installations are required |
示例 1:使用 Pyglet 库制作一个简单的绿色圆圈
在此示例中,我们从 Pyglet 库导入形状并创建一个窗口来呈现我们的形状。之后,我们使用 Pyglet 模块制作一个绿色圆圈。
Python3
# pip install pyglet
import pyglet
# importing shapes from pyglet library
from pyglet import shapes
# Canvas
window = pyglet.window.Window(960, 540)
batch = pyglet.graphics.Batch()
# Making Green Circle
circle = shapes.Circle(700, 150, 100,
color=(50, 225, 30),
batch=batch)
@window.event
def on_draw():
window.clear()
batch.draw()
pyglet.app.run()
输出:
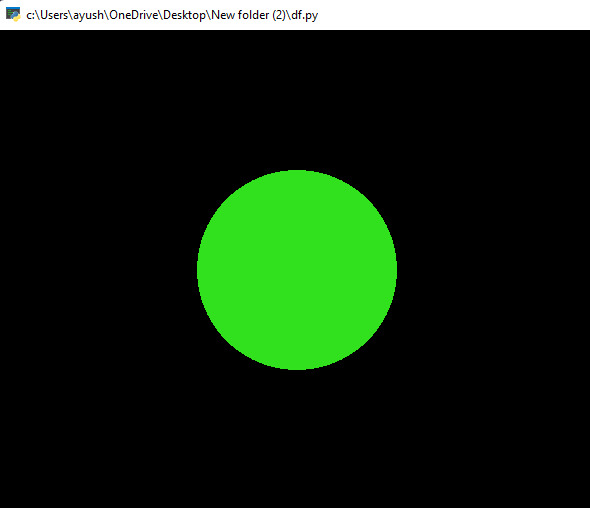
示例 2:使用 Pygame 库制作绿色矩形
在这个例子中,我们从 Pygame 库导入我们的库并创建一个 600*700 的窗口来呈现我们的形状。之后,我们使用 Pygame 模块的方法 pygame.draw.rect() 制作一个绿色矩形。
Python3
# pip install pygame
import pygame
pygame.init()
# Canvas size
screen = pygame.display.set_mode([600, 700])
# Program will run until the user quits
# the program
running = True
while running:
# If User clicked the close button
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# Settingup the background color
screen.fill((0, 0, 0))
# Making the green circle
pygame.draw.rect(screen, (0, 255, 0),
[100, 100, 400, 100], 2)
# Flip the display
pygame.display.flip()
# Quit
pygame.quit()
输出:
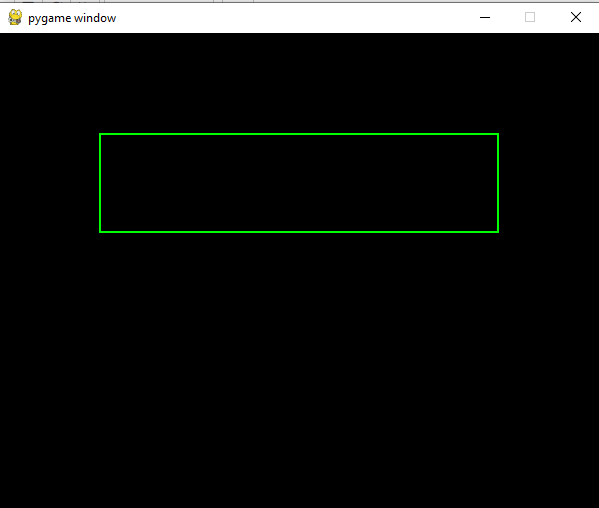
相关用法
- Python Pygorithm用法及代码示例
- Python PyTorch acos()用法及代码示例
- Python PyTorch asin()用法及代码示例
- Python PyTorch atan()用法及代码示例
- Python PyTorch cos()用法及代码示例
- Python PyTorch cosh()用法及代码示例
- Python PyTorch sin()用法及代码示例
- Python PyTorch sinh()用法及代码示例
- Python PyTorch tan()用法及代码示例
- Python PyTorch tanh()用法及代码示例
- Python PyTorch from_numpy()用法及代码示例
- Python Pytorch randn()用法及代码示例
- Python Pytorch permute()用法及代码示例
- Python PyTorch div()用法及代码示例
- Python PyTorch clamp()用法及代码示例
- Python PyTorch ceil()用法及代码示例
- Python PyTorch add()用法及代码示例
- Python PyTorch abs()用法及代码示例
- Python PyTorch exp()用法及代码示例
- Python PyTorch numel()用法及代码示例
- Python PyTorch is_storage()用法及代码示例
- Python PyTorch is_tensor()用法及代码示例
- Python PyTorch trunc()用法及代码示例
- Python PyTorch frac()用法及代码示例
- Python PyTorch log()用法及代码示例
注:本文由纯净天空筛选整理自ayushcoding100大神的英文原创作品 Differences Between Pyglet and Pygame in Python。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。