在本文中,我們將看到 Pygame 和 Pyglet 遊戲庫之間的區別。
什麽是 Pyglet?
皮格萊特 是 Python 的跨平台窗口和媒體庫,旨在開發遊戲和其他視覺效果豐富的應用程序。它支持窗口、UI 事件處理、遊戲手柄、OpenGL 圖形、圖像和視頻加載以及聲音和音樂播放。 Pyglet 可在 Windows、OS X 和 Linux 上運行。 Pyglet 完全用純 Python 編寫,並使用標準庫的類型模塊與係統庫交互。您可以修改代碼庫或做出貢獻,而無需編譯或了解其他語言。雖然 Pyglet 是純 Python,但它提供了出色的批處理和 GPU 渲染性能。您可以使用 thing 模塊輕鬆繪製數千個精靈或動畫。
特征:
- 沒有外部依賴項或安裝要求,Pyglet 除了 Python 之外不需要任何東西。
- Pyglet 支持多種 platform-native 窗口和 multi-monitor 全屏遊戲設置。
- 下載幾乎任何格式的圖像、聲音、音樂和視頻。
- Pyglet 是在 BSD 開源許可證下發布的,允許您將其用於商業和其他開源項目,幾乎沒有任何限製。
安裝
pip install pyglet
什麽是 Pygame?
pygame 是一組用於創建視頻遊戲的跨平台 Python 模塊。它由設計用於 Python 編程語言的計算機圖像和聲音庫組成。 Pygame 適合構建可以封裝在獨立可執行文件中的客戶端應用程序。 Pygame 是專為視頻遊戲設計而創建的跨平台 Python 軟件。它還包括可用於增強design-time遊戲玩法的圖形、圖像和聲音,遊戲開發包括數學、邏輯、物理、人工智能等等,它可以非常有趣。它包含各種可處理圖像和聲音並可以創建遊戲圖形的庫。它簡化了整個遊戲設計過程,使初學者的遊戲開發變得更加容易。
特征:
- 易於編寫,它沒有數十萬行代碼來處理您無論如何都不會使用的東西。核心保持簡單,GUI 庫和效果等額外的東西是在 Pygame 之外單獨開發的。
- 所有函數的使用並不需要使用 GUI。如果您隻想處理圖像、獲取操縱杆輸入或播放聲音,則可以從命令行使用 Pygame。
- 對報告的錯誤快速響應, 如今,錯誤報告非常罕見,因為其中許多已經得到修複。
- 對代碼的更多控製,這使您在使用其他庫和不同類型的程序時擁有更多控製。
安裝
pip install pygame
Pyglet 和 Pygame 之間的差異表:
Pyglet |
Pygame |
---|---|
Pyglet is much faster as compared to the Pygame |
Pygame is slower as compared with Pyglet |
Pyglet uses OpenGL |
Pygame uses SDL libraries and does not require OpenGL. |
It is rich with GUI elements. |
GUI using Pygame is not compatible. |
3D projects are supported in Pyglet |
Only 2D projects are Supported. |
Pyglet support Music, video, and image in all format |
Pygame 支持幾種格式作為組件 |
No external installation requirements. |
Few module installations are required |
示例 1:使用 Pyglet 庫製作一個簡單的綠色圓圈
在此示例中,我們從 Pyglet 庫導入形狀並創建一個窗口來呈現我們的形狀。之後,我們使用 Pyglet 模塊製作一個綠色圓圈。
Python3
# pip install pyglet
import pyglet
# importing shapes from pyglet library
from pyglet import shapes
# Canvas
window = pyglet.window.Window(960, 540)
batch = pyglet.graphics.Batch()
# Making Green Circle
circle = shapes.Circle(700, 150, 100,
color=(50, 225, 30),
batch=batch)
@window.event
def on_draw():
window.clear()
batch.draw()
pyglet.app.run()
輸出:
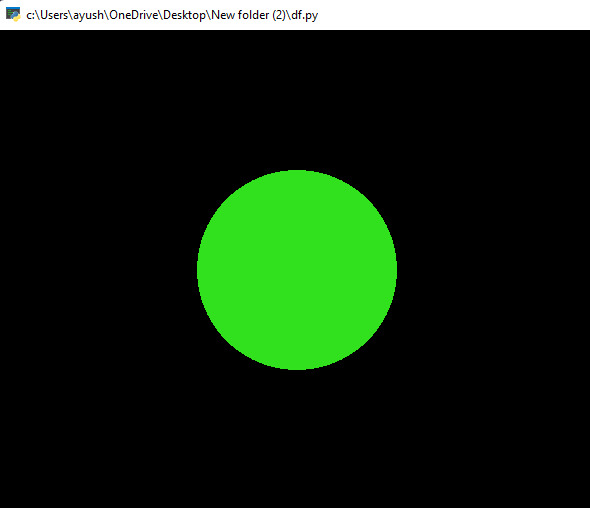
示例 2:使用 Pygame 庫製作綠色矩形
在這個例子中,我們從 Pygame 庫導入我們的庫並創建一個 600*700 的窗口來呈現我們的形狀。之後,我們使用 Pygame 模塊的方法 pygame.draw.rect() 製作一個綠色矩形。
Python3
# pip install pygame
import pygame
pygame.init()
# Canvas size
screen = pygame.display.set_mode([600, 700])
# Program will run until the user quits
# the program
running = True
while running:
# If User clicked the close button
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# Settingup the background color
screen.fill((0, 0, 0))
# Making the green circle
pygame.draw.rect(screen, (0, 255, 0),
[100, 100, 400, 100], 2)
# Flip the display
pygame.display.flip()
# Quit
pygame.quit()
輸出:
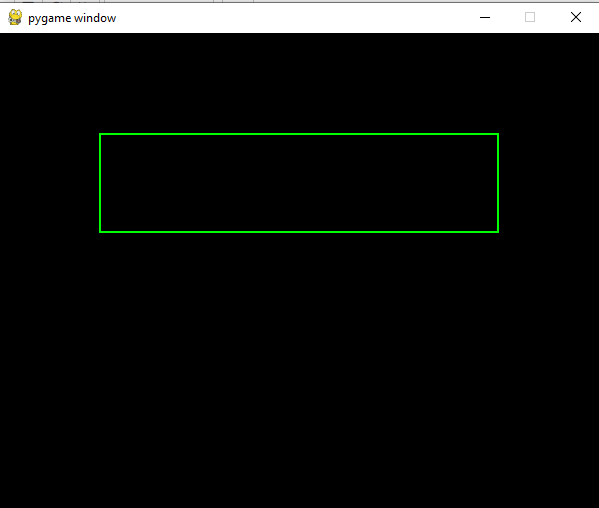
相關用法
- Python Pygorithm用法及代碼示例
- Python PyTorch acos()用法及代碼示例
- Python PyTorch asin()用法及代碼示例
- Python PyTorch atan()用法及代碼示例
- Python PyTorch cos()用法及代碼示例
- Python PyTorch cosh()用法及代碼示例
- Python PyTorch sin()用法及代碼示例
- Python PyTorch sinh()用法及代碼示例
- Python PyTorch tan()用法及代碼示例
- Python PyTorch tanh()用法及代碼示例
- Python PyTorch from_numpy()用法及代碼示例
- Python Pytorch randn()用法及代碼示例
- Python Pytorch permute()用法及代碼示例
- Python PyTorch div()用法及代碼示例
- Python PyTorch clamp()用法及代碼示例
- Python PyTorch ceil()用法及代碼示例
- Python PyTorch add()用法及代碼示例
- Python PyTorch abs()用法及代碼示例
- Python PyTorch exp()用法及代碼示例
- Python PyTorch numel()用法及代碼示例
- Python PyTorch is_storage()用法及代碼示例
- Python PyTorch is_tensor()用法及代碼示例
- Python PyTorch trunc()用法及代碼示例
- Python PyTorch frac()用法及代碼示例
- Python PyTorch log()用法及代碼示例
注:本文由純淨天空篩選整理自ayushcoding100大神的英文原創作品 Differences Between Pyglet and Pygame in Python。非經特殊聲明,原始代碼版權歸原作者所有,本譯文未經允許或授權,請勿轉載或複製。