PySpark DataFrame 的 join(~)
方法使用给定的连接方法连接两个 DataFrames。
参数
1. other
| DataFrame
要连接的另一个 PySpark DataFrame。
2. on
| string
或 list
或 Column
| optional
要执行连接的列。
3. how
| string
| optional
默认情况下,how="inner"
。有关实现的联接类型,请参阅下面的示例。
返回值
PySpark 数据帧 (pyspark.sql.dataframe.DataFrame
)。
例子
执行内连接、左连接和右连接
考虑以下PySpark DataFrame:
df1 = spark.createDataFrame([["Alex", 20], ["Bob", 24], ["Cathy", 22]], ["name", "age"])
df1.show()
+-----+---+
| name|age|
+-----+---+
| Alex| 20|
| Bob| 24|
|Cathy| 22|
+-----+---+
另一个PySpark DataFrame:
df2 = spark.createDataFrame([["Alex", 250], ["Bob", 200], ["Doge", 100]], ["name", "salary"])
df2.show()
+----+------+
|name|salary|
+----+------+
|Alex| 250|
| Bob| 200|
|Doge| 100|
+----+------+
内部联接
对于内部联接,在源 DataFrame 中具有匹配值的所有行都将出现在生成的 DataFrame 中:

df1.join(df2, on="name", how="inner").show() # how="cross" also works
+----+---+------+
|name|age|salary|
+----+---+------+
|Alex| 20| 250|
| Bob| 24| 200|
+----+---+------+
左连接和left-outer连接
对于左连接(或left-outer连接),左DataFrame中的所有行和右DataFrame中的匹配行将出现在生成的DataFrame中:
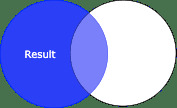
df1.join(df2, on="name", how="left").show() # how="left_outer" works
+-----+---+------+
| name|age|salary|
+-----+---+------+
| Alex| 20| 250|
| Bob| 24| 200|
|Cathy| 22| null|
+-----+---+------+
右连接和right-outer连接
对于右 (right-outer) 连接,右 DataFrame 中的所有行和左 DataFrame 中的匹配行将出现在生成的 DataFrame 中:
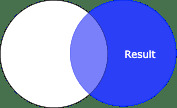
df1.join(df2, on="name", how="right").show() # how="right_outer" also works
+----+----+------+
|name| age|salary|
+----+----+------+
|Alex| 20| 250|
| Bob| 24| 200|
|Doge|null| 100|
+----+----+------+
执行外连接
考虑与之前相同的 PySpark DataFrame:
df1 = spark.createDataFrame([["Alex", 20], ["Bob", 24], ["Cathy", 22]], ["name", "age"])
df1.show()
+-----+---+
| name|age|
+-----+---+
| Alex| 20|
| Bob| 24|
|Cathy| 22|
+-----+---+
这是另一个PySpark DataFrame:
df2 = spark.createDataFrame([["Alex", 250], ["Bob", 200], ["Doge", 100]], ["name", "salary"])
df2.show()
+----+------+
|name|salary|
+----+------+
|Alex| 250|
| Bob| 200|
|Doge| 100|
+----+------+
对于外连接,左和右 DataFrames 都会出现:

df1.join(df2, on="name", how="outer").show() # how="full" or "fullouter" also works
+-----+----+------+
| name| age|salary|
+-----+----+------+
| Alex| 20| 250|
| Bob| 24| 200|
|Cathy| 22| null|
| Doge|null| 100|
+-----+----+------+
执行 left-anti 和 left-semi 连接
考虑与之前相同的 PySpark DataFrame:
df1 = spark.createDataFrame([["Alex", 20], ["Bob", 24], ["Cathy", 22]], ["name", "age"])
df1.show()
+-----+---+
| name|age|
+-----+---+
| Alex| 20|
| Bob| 24|
|Cathy| 22|
+-----+---+
这是另一个 DataFrame :
df2 = spark.createDataFrame([["Alex", 250], ["Bob", 200], ["Doge", 100]], ["name", "salary"])
df2.show()
+----+------+
|name|salary|
+----+------+
|Alex| 250|
| Bob| 200|
|Doge| 100|
+----+------+
左反连接
对于左反连接,左侧 DataFrame 中不存在于右侧 DataFrame 中的所有行都将出现在生成的 DataFrame 中:
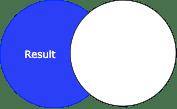
df1.join(df2, on="name", how="left_anti").show() # how="leftanti" also works
+-----+---+
| name|age|
+-----+---+
|Cathy| 22|
+-----+---+
左semi-join
左 semi-join 与 left-anti 连接相反,即左 DataFrame 中存在于右 DataFrame 中的所有行都将出现在生成的 DataFrame 中:
df1.join(df2, on="name", how="left_semi").show() # how="leftsemi" also works
+----+---+
|name|age|
+----+---+
|Alex| 20|
| Bob| 24|
+----+---+
对不同的列名执行连接
到目前为止,我们已经使用on
参数指定了连接键。现在让我们考虑连接键具有不同标签的情况。假设一个DataFrame如下:
df1 = spark.createDataFrame([["Alex", 20], ["Bob", 24], ["Cathy", 22]], ["name", "age"])
df1.show()
+-----+---+
| name|age|
+-----+---+
| Alex| 20|
| Bob| 24|
|Cathy| 22|
+-----+---+
假设另一个DataFrame如下:
df2 = spark.createDataFrame([["Alex", 250], ["Bob", 200], ["Doge", 100]], ["NAME", "salary"])
df2.show()
+----+------+
|NAME|salary|
+----+------+
|Alex| 250|
| Bob| 200|
|Doge| 100|
+----+------+
我们可以使用 df1
的 name
和 df2
的 NAME
加入,如下所示:
cond = [df1["name"] == df2["NAME"]]
df1.join(df2, on=cond, how="inner").show()
+----+---+----+------+
|name|age|NAME|salary|
+----+---+----+------+
|Alex| 20|Alex| 250|
| Bob| 24| Bob| 200|
+----+---+----+------+
在这里,我们可以提供多个连接键,因为 on
接受一个列表。
相关用法
- Python Pandas DataFrame join方法用法及代码示例
- Python Pandas DataFrame empty属性用法及代码示例
- Python Pandas DataFrame pop方法用法及代码示例
- Python Pandas DataFrame nsmallest方法用法及代码示例
- Python Pandas DataFrame sample方法用法及代码示例
- Python Pandas DataFrame items方法用法及代码示例
- Python Pandas DataFrame max方法用法及代码示例
- Python Pandas DataFrame swaplevel方法用法及代码示例
- Python Pandas DataFrame agg方法用法及代码示例
- Python Pandas DataFrame copy方法用法及代码示例
- Python Pandas DataFrame pow方法用法及代码示例
- Python Pandas DataFrame insert方法用法及代码示例
- Python Pandas DataFrame lt方法用法及代码示例
- Python Pandas DataFrame all方法用法及代码示例
- Python Pandas DataFrame unstack方法用法及代码示例
- Python Pandas DataFrame mean方法用法及代码示例
- Python PySpark DataFrame filter方法用法及代码示例
- Python Pandas DataFrame tz_convert方法用法及代码示例
- Python Pandas DataFrame isin方法用法及代码示例
- Python PySpark DataFrame collect方法用法及代码示例
- Python PySpark DataFrame intersect方法用法及代码示例
- Python PySpark DataFrame dtypes属性用法及代码示例
- Python Pandas DataFrame rank方法用法及代码示例
- Python Pandas DataFrame tail方法用法及代码示例
- Python Pandas DataFrame transform方法用法及代码示例
注:本文由纯净天空筛选整理自Isshin Inada大神的英文原创作品 PySpark DataFrame | join method。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。