jQuery 中的 each() 方法指定了一個為每個匹配元素運行的函數。它是 JQuery 中廣泛使用的遍曆方法之一。使用這個方法,我們可以遍曆 jQuery 對象的 DOM 元素,並且可以為每個匹配的元素執行一個函數。
each() 接受一個參數 function(index,element),它是一個為每個選定元素執行的回調函數。此函數進一步可選地需要兩個參數,即索引和元素。因此,我們必須將回調函數傳遞給 each() 方法。
我們還可以從回調函數中返回 false 以提前停止循環。
用法
$(selector).each(function(index, element))
參數值
each() 方法中使用的參數值定義如下。
function(index,element):它是一個強製性參數。它是為每個選定元素執行的回調函數。它有兩個參數值,定義如下。
- index:它是一個整數值,用於指定選擇器的索引位置。
- element:它是當前元素。我們可以使用這個關鍵字來引用當前匹配的元素。
讓我們看一些插圖來清楚地理解 each() 方法。
示例 1
在此示例中,將在單擊按鈕時觸發 each() 方法。我們將此方法應用於 li 元素。因此,此方法將迭代每個 li 元素。該函數針對每個選定的 li 執行,並使用警告框顯示相應 li 元素的文本。
在這裏,我們沒有使用回調函數的參數值。
<!DOCTYPE html>
<html>
<head>
<title> jQuery each() method </title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
</head>
<body>
<h2> Welcome to the javaTpoint.com </h2>
<ul>
<li> First element </li>
<li> Second element </li>
<li> Third element </li>
<li> Fourth element </li>
</ul>
<p>
Click the following button to see the list of <b> li </b> elements.
</p>
<button onclick = fun()> Click me </button>
<script>
function fun(){
$(document).ready(function(){
$("li").each(function(){
alert($(this).text())
});
});
}
</script>
</body>
</html>
輸出
執行上述代碼後,輸出將是 -
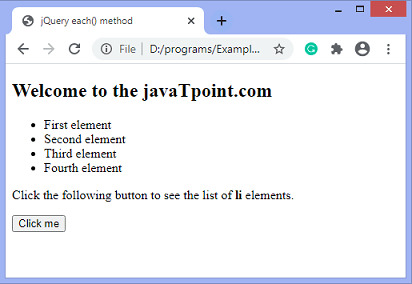
單擊該按鈕後,將顯示如下警告。
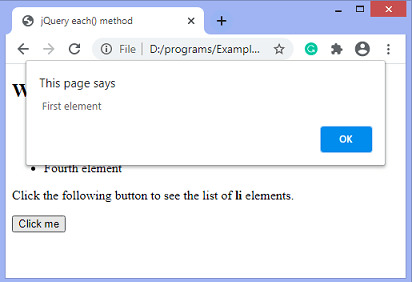
同樣,由於四個 li 元素,將顯示四個警告框。
例2
在這個例子中,我們使用回調函數的參數值 index 和 element。
我們在 li 元素上應用 each() 方法。因此,該方法將從索引 0 開始迭代 li 元素。它將在每個選定的 li 元素上執行並更改相應元素的背景顏色。
一旦函數返回false,迭代就會停止。這裏有六個 li 元素,當函數到達 id = "i4" 的元素時停止。雖然是第四個元素,但是索引從0開始,所以元素的位置是3。
<!DOCTYPE html>
<html>
<head>
<title> jQuery each() method </title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<style>
body{
text-align:center;
}
ul{
list-style-type:none;
float:left;
}
li {
width:40px;
height:40px;
margin:5px;
padding:5px;
font-size:20px;
float:left;
border:2px solid blue;
}
button{
font-size:20px;
}
</style>
</head>
<body>
<h2> Welcome to the javaTpoint.com </h2>
<ul>
<li> 1 </li>
<li> 2 </li>
<li> 3 </li>
<li id = "i4"> Stop </li>
<li> 5 </li>
<li> 6 </li>
</ul>
<button onclick = "fun()"> Click me </button>
<p></p>
<script>
function fun() {
$(document).ready(function(){
$("li").each(function(index, element) {
$(element).css("background", "lightgreen");
if ($(this).is("#i4")) {
$("p").text("Index begins with 0. So, the function stopped at position:" + index ).css("fontSize", "20px");
return false;
}
});
});
}
</script>
</body>
</html>
輸出
執行上述代碼後,單擊給定的按鈕,輸出將是 -

相關用法
- JQuery event.isDefaultPrevented()用法及代碼示例
- JQuery event.which用法及代碼示例
- JQuery error()用法及代碼示例
- JQuery event.currentTarget用法及代碼示例
- JQuery event.isPropagationStopped()用法及代碼示例
- JQuery event.stopImmediatePropagation()用法及代碼示例
- JQuery event.relatedTarget用法及代碼示例
- JQuery event.preventDefault()用法及代碼示例
- JQuery event.type用法及代碼示例
- JQuery extend()用法及代碼示例
- JQuery event.pageX用法及代碼示例
- JQuery event.pageY用法及代碼示例
- JQuery event.namespace用法及代碼示例
- JQuery event.delegateTarget用法及代碼示例
- JQuery eq()用法及代碼示例
- JQuery event.isImmediatePropagationStopped()用法及代碼示例
- JQuery event.stopPropagation()用法及代碼示例
- JQuery end()用法及代碼示例
- JQuery event.target用法及代碼示例
- JQuery event.data用法及代碼示例
注:本文由純淨天空篩選整理自 jQuery each() method。非經特殊聲明,原始代碼版權歸原作者所有,本譯文未經允許或授權,請勿轉載或複製。