Python OpenCV imencode() 函数将图像格式转换(编码)为流数据并将其存储在内存缓存中。它主要用于压缩图像数据格式,以便于网络传输。
基本示例imencode()函数
示例 1:
我们首先导入必要的库,即 OpenCV 和 NumPy 。导入库后,我们使用imread() 函数加载图像,并使用图像路径作为参数。加载图像后,我们开始使用 imencode() 方法对其进行编码,传递要编码的图像的扩展名以及加载的图像作为参数。
结果将根据格式而有所不同。如果您注意到,我们只保存imencode()方法的第一个索引的数据,因为它产生两个输出:零索引处的操作是否成功,以及第一个索引处的编码图像。现在我们将编码图像转换为 NumPy 数组,以便我们可以使用它。最后,我们将这个NumPy数组转换为字节,以便可以轻松传输。
使用的图像:
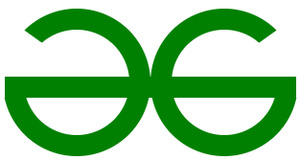
gfg.png
代码:
Python3
# This code demonstrates encoding of image.
import numpy as np
import cv2 as cv
# Passing path of image as parameter
img = cv.imread('/content/gfg.png')
# If the extension of our image was
# '.jpg' then we have passed it as
# argument instead of '.png'.
img_encode = cv.imencode('.png', img)[1]
# Converting the image into numpy array
data_encode = np.array(img_encode)
# Converting the array to bytes.
byte_encode = data_encode.tobytes()
print(byte_encode)
输出:(由于输出较长,这里只显示一部分)
b’\x89PNG\r\n\x1a\n\x00\x00\x00\rIHDR\x00\x00\x01,\x00\x00\x00\xa0\x08\x02\x00\x00\x009\x1a\xc65\x00\ …………
示例2:
使用的图像:
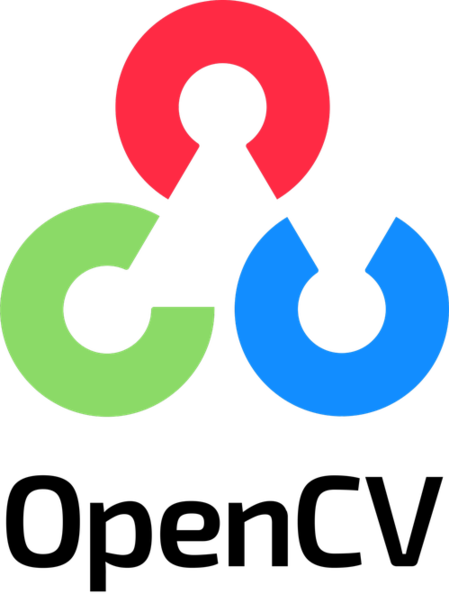
openCV.png
代码:
Python3
import numpy as np
import cv2 as cv
img = cv.imread('/content/OpenCV.png')
img_encode = cv.imencode('.jpg', img)[1]
data_encode = np.array(img_encode)
byte_encode = data_encode.tobytes()
print(byte_encode)
输出:
b’\xff\xd8\xff\xe0\x00\x10JFIF\x00\x01\x01\x00\x00\x01\x00\x01\x00\x00\xff\xdb\x00C\x00\x02\……..
相关用法
- Python OpenCV imdecode()用法及代码示例
- Python OpenCV cv2.circle()用法及代码示例
- Python OpenCV cv2.blur()用法及代码示例
- Python OpenCV cv2.ellipse()用法及代码示例
- Python OpenCV cv2.cvtColor()用法及代码示例
- Python OpenCV cv2.copyMakeBorder()用法及代码示例
- Python OpenCV cv2.imread()用法及代码示例
- Python OpenCV cv2.imshow()用法及代码示例
- Python OpenCV cv2.imwrite()用法及代码示例
- Python OpenCV cv2.putText()用法及代码示例
- Python OpenCV cv2.rectangle()用法及代码示例
- Python OpenCV cv2.arrowedLine()用法及代码示例
- Python OpenCV cv2.erode()用法及代码示例
- Python OpenCV cv2.line()用法及代码示例
- Python OpenCV cv2.flip()用法及代码示例
- Python OpenCV cv2.transpose()用法及代码示例
- Python OpenCV cv2.rotate()用法及代码示例
- Python OpenCV cv2.polylines()用法及代码示例
- Python OpenCV Canny()用法及代码示例
- Python OpenCV destroyAllWindows()用法及代码示例
- Python OpenCV Filter2D()用法及代码示例
- Python OpenCV getgaussiankernel()用法及代码示例
- Python OpenCV getRotationMatrix2D()用法及代码示例
- Python OpenCV getTrackbarPos()用法及代码示例
- Python OpenCV haveImageReader()用法及代码示例
注:本文由纯净天空筛选整理自sp3768546大神的英文原创作品 Python OpenCV – imencode() Function。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。