在本文中,我们将了解OpenCV 库的distanceTransform() 函数。要使用此函数,您需要在 Python environment 中包含 OpenCV library installed 。
distanceTransform() 函数是什么?
OpenCV distanceTransform() 函数是一个图像处理函数,用于计算图像中每个非零像素与最近的零像素之间的欧几里德距离。它计算用于分割目的的距离图。此方法返回灰度图片,其中每个像素值表示距所提供图像中最近的非零像素的距离。
distanceTransform()函数的语法
用法: cv2.distanceTransform(src, distanceType, maskSize[, dst[, labels[, distanceMask]]]) -> dst
参数:
该函数接受以下参数:
- src:输入图片,应该是单通道的8位或32位浮点图像。
- distanceType:用于计算距离的距离类型。可以使用以下常量之一:掩码 cv2.DIST L1、掩码 cv2.DIST L2、掩码 cv2.DIST C 或掩码 cv2.DIST L12。
- maskSize:距离变换掩模的大小。可以使用以下常量之一:cv2.DIST MASK 3、cv2.DIST MASK 5、cv2.DIST MASK PRECISE 和 cv2.DIST MASK PRECISE。
示例 1
我们将在代码中使用下图。
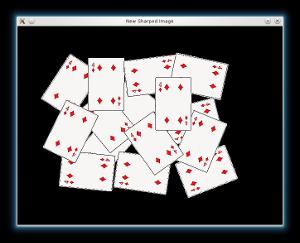
然后,您可以导入 cv2 模块并调用 distanceTransform() 函数,将其传递给适当的参数。此函数用于在加载图像并对其进行阈值处理后对其进行转换以生成二进制图像。 5×5 像素的邻域和 L2(欧几里得)距离用于确定距离变换。接下来,使用OpenCV对距离变换图像进行归一化和显示。
Python3
import cv2
import numpy as np
# Load the input image and make it grayscale.
image = cv2.imread('cards.jpeg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Create a binary image by throttling the image.
ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
#Determine the distance transform.
dist = cv2.distanceTransform(thresh, cv2.DIST_L2, 5)
# Make the distance transform normal.
dist_output = cv2.normalize(dist, None, 0, 1.0, cv2.NORM_MINMAX)
# Display the distance transform
cv2.imshow('Distance Transform', dist_output)
cv2.waitKey(0)
输出:
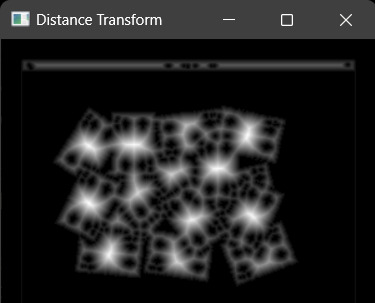
示例 2
我们将在代码中使用下图。
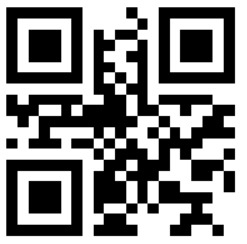
在这种情况下,照片会加载、设置阈值并传递到远处。利用 3×3 邻域和距离测量 DIST LABEL PIXEL,transform() 函数。此外,OpenCV 用于标准化和显示输出。
Python3
import cv2
import numpy as np
# Load appropriate image
img = cv2.imread('randomqr.png', 0)
# Threshold the image to convert a binary image
ret, thresh = cv2.threshold(img, 127,
255, cv2.THRESH_BINARY)
# Perform the distance transform
dist = cv2.distanceTransform(thresh,
cv2.DIST_LABEL_PIXEL, 3)
# Standardize the distance transform
normalized = cv2.normalize(dist, None, 0,
255, cv2.NORM_MINMAX, cv2.CV_8U)
# Display the distance transform image
cv2.imshow('Distance Transform', normalized)
cv2.waitKey(0)
cv2.destroyAllWindows()
输出:
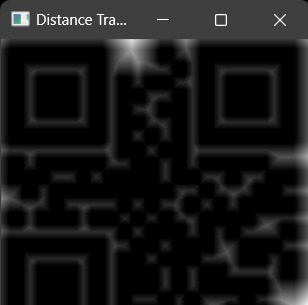
相关用法
- Python OpenCV destroyAllWindows()用法及代码示例
- Python OpenCV drawKeypoints()用法及代码示例
- Python OpenCV drawMatchesKnn()用法及代码示例
- Python OpenCV destroyWindow()用法及代码示例
- Python OpenCV cv2.circle()用法及代码示例
- Python OpenCV cv2.blur()用法及代码示例
- Python OpenCV cv2.ellipse()用法及代码示例
- Python OpenCV cv2.cvtColor()用法及代码示例
- Python OpenCV cv2.copyMakeBorder()用法及代码示例
- Python OpenCV cv2.imread()用法及代码示例
- Python OpenCV cv2.imshow()用法及代码示例
- Python OpenCV cv2.imwrite()用法及代码示例
- Python OpenCV cv2.putText()用法及代码示例
- Python OpenCV cv2.rectangle()用法及代码示例
- Python OpenCV cv2.arrowedLine()用法及代码示例
- Python OpenCV cv2.erode()用法及代码示例
- Python OpenCV cv2.line()用法及代码示例
- Python OpenCV cv2.flip()用法及代码示例
- Python OpenCV cv2.transpose()用法及代码示例
- Python OpenCV cv2.rotate()用法及代码示例
- Python OpenCV cv2.polylines()用法及代码示例
- Python OpenCV Canny()用法及代码示例
- Python OpenCV Filter2D()用法及代码示例
- Python OpenCV getgaussiankernel()用法及代码示例
- Python OpenCV getRotationMatrix2D()用法及代码示例
注:本文由纯净天空筛选整理自nomaanrz17大神的英文原创作品 Python OpenCV – distanceTransform() Function。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。