Mongoose SchemaType.prototype.index() 是一种用于在 Mongoose 模式中的字段上创建索引的方法。索引是一种数据结构,允许高效查询数据库中的数据。它可以在集合中创建一个或多个字段,以加快使用这些字段作为搜索条件的查询。在字段上创建索引时,数据库会创建一个单独的数据结构,用于存储该字段的值以及对包含每个值的文档的引用。
用法:
SchemaType.prototype.index( options )
Parameters: 该方法仅采用一个参数:
- options (optional):指定索引选项的对象。此参数可以采用对象、布尔值、字符串或数字作为参数。
返回类型:它返回一个 SchemaType 对象作为响应。
创建节点应用程序并安装 Mongoose:
1. 您可以使用此命令安装此软件包。
npm install mongoose
2.安装mongoose模块后,您可以在命令提示符中使用命令检查您的mongoose版本。
npm version mongoose
3. 之后,您可以创建一个文件夹并添加一个文件,例如index.js,要运行该文件,您需要运行以下命令。
node index.js
项目结构: 项目结构将如下所示:
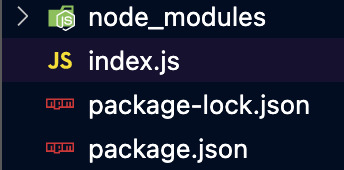
示例 1:在此示例中,我们为具有姓名、电子邮件、年龄和地址字段的用户创建 Mongoose 模式。然后,我们使用 SchemaType.prototype.index() 方法在模式中的不同字段上创建各种类型的索引。定义架构并创建索引后,我们连接到 MongoDB 数据库,创建一个新的用户文档,并将其保存到数据库。最后,我们记录新创建的用户名。
Index.js
Javascript
const mongoose = require("mongoose");
const { Schema } = mongoose;
mongoose.set("strictQuery", true);
const userSchema = new Schema({
name: {
type: String,
required: true,
},
email: {
type: String,
required: true,
},
age: Number,
address: {
street: String,
city: String,
state: String,
zip: String,
},
});
// Creating indexes on the email and age fields
userSchema.index({ email: 1 }, { unique: true });
userSchema.index({ age: 1 });
// Creating a compound index on the address fields
userSchema.index({ "address.city": 1, "address.state": 1 });
// Creating a text index on the name and address fields
userSchema.index({
name: "text",
"address.street": "text",
"address.city": "text",
"address.state": "text",
});
// Creating a 2dsphere index on the address field for
// geo-spatial queries
userSchema.index({ "address.coordinates": "2dsphere" });
// Creating a hashed index on the name field for
//efficient sharding
userSchema.index({ name: "hashed" });
// Creating a partial index on the age field for documents
// where the age field is less than or equal to 30
userSchema.index(
{ age: 1 },
{ partialFilterExpression: { age: { $lte: 30 } } }
);
const User = mongoose.model("User", userSchema);
// Connecting to a MongoDB database and creating a new user
mongoose
.connect("mongodb://localhost:27017/geeksforgeeks",
{ useNewUrlParser: true })
.then(() => {
console.log("Connected to MongoDB");
const newUser = new User({
name: "John Doe",
email: "johndoe@example.com",
age: 25,
address: {
street: "123 Main St",
city: "Anytown",
state: "CA",
zip: "12345",
},
});
return newUser.save();
})
.then((user) => {
console.log(`Created new user: ${user.name}`);
mongoose.connection.close();
})
.catch((err) => console.error(err));
运行代码的步骤:
1.确认是否安装了mongoose模块。
npm install mongoose
2. 使用以下命令运行代码:
node index.js
输出:

示例 2:在此示例中,我们定义了一个 movieSchema,其中包含一个名为 actor 的子文档数组字段。然后,我们使用 index() 方法在 actors.name 字段上创建唯一索引。该索引将强制 actor 子文档数组中 actors.name 字段的唯一性。
然后,我们使用重复的演员名称创建一部新电影,并尝试将其保存到数据库中。由于actors.name字段是唯一的,MongoDB将抛出重复键错误,并且保存操作将失败。
Index.js
Javascript
const mongoose = require("mongoose");
const { Schema } = mongoose;
mongoose.set("strictQuery", true);
const movieSchema = new Schema({
title: {
type: String,
required: true,
},
actors: [
{
name: {
type: String,
required: true,
},
character: {
type: String,
required: true,
},
},
],
});
// Creating a unique index on the 'actors.name' field within
// the 'actors' subdocument array
movieSchema.index({ "actors.name": 1 }, { unique: true });
const Movie = mongoose.model("Movie", movieSchema);
// Connecting to a MongoDB database and creating a new user
mongoose
.connect("mongodb://localhost:27017/geeksforgeeks",
{ useNewUrlParser: true })
.then(() => {
console.log("Connected to MongoDB");
const newMovie = new Movie({
title: "The Avengers",
actors: [
{ name: "Robert Downey Jr.", character: "Iron Man" },
{ name: "Chris Evans", character: "Captain America" },
{ name: "Robert Downey Jr.", character: "Tony Stark" },
// Duplicate actor name
],
});
return newMovie.save();
})
.then((movie) => {
console.log(`Created new movie: ${movie.title}`);
mongoose.connection.close();
})
.catch((err) => console.error(err));
运行代码的步骤: 运行以下命令:
node index.js
输出:
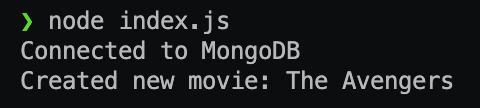
参考:https://mongoosejs.com/docs/api/schematype.html#schematype_SchemaType-index
相关用法
- Mongoose SchemaType.prototype.immutable()用法及代码示例
- Mongoose SchemaType.prototype.ref()用法及代码示例
- Mongoose SchemaType.prototype.default()用法及代码示例
- Mongoose SchemaType.prototype.unique()用法及代码示例
- Mongoose SchemaType.prototype.validate()用法及代码示例
- Mongoose SchemaType.prototype.get()用法及代码示例
- Mongoose SchemaType.prototype.text()用法及代码示例
- Mongoose SchemaType.prototype.set()用法及代码示例
- Mongoose SchemaType.prototype.required()用法及代码示例
- Mongoose SchemaType.prototype.select()用法及代码示例
- Mongoose SchemaType.prototype.transform()用法及代码示例
- Mongoose Schema Connection.prototype.asPromise()用法及代码示例
- Mongoose Schema Connection.prototype.dropCollection()用法及代码示例
- Mongoose Schema.prototype.virtual()用法及代码示例
- Mongoose Schema Connection.prototype.set()用法及代码示例
- Mongoose Schema Connection.prototype.dropDatabase()用法及代码示例
- Mongoose Schema.prototype.plugin()用法及代码示例
- Mongoose Schema Connection.prototype.close()用法及代码示例
- Mongoose Schema.prototype.static()用法及代码示例
- Mongoose Schema Connection.prototype.useDb()用法及代码示例
- Mongoose Schema.prototype.pre()用法及代码示例
- Mongoose countDocuments()用法及代码示例
- Mongoose deleteMany()用法及代码示例
- Mongoose deleteOne()用法及代码示例
- Mongoose estimatedDocumentCount()用法及代码示例
注:本文由纯净天空筛选整理自ianurag大神的英文原创作品 Mongoose SchemaType.prototype.index() API。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。