Mongoose API 的 Mongoose Schema API Connection.prototype.asPromise() 方法用于 Connection 对象。如果与 MongoDB 的连接成功连接,它允许我们将结果作为 Promise 获取,这意味着 Promise 得到解决,否则 Promise 被拒绝。让我们通过一个例子来理解asPromise()方法。
用法:
connection.asPromise();
Parameters: 该方法不接受任何参数。
返回值:此方法返回一个可以使用 then 和 catch 块或使用异步函数处理的 Promise。
设置 Node.js Mongoose 模块:
步骤 1:使用以下命令创建 Node.js 应用程序:
npm init
步骤 2:创建 NodeJS 应用程序后,使用以下命令安装所需的模块:
npm install mongoose
项目结构: 项目结构将如下所示:
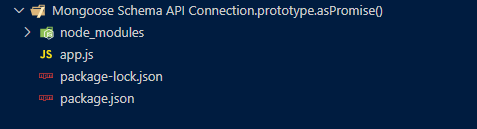
示例 1:下面的示例说明了 Mongoose Connection 的基本函数asPromise()方法,使用 then 和 catch 块。在此示例中,我们显示由以下方法解析的所有属性asPromise()方法,并访问结果对象上的数据库名称。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
const URI = "mongodb://localhost:27017/geeksforgeeks"
const connectionObject = mongoose.createConnection(URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
}).asPromise();
connectionObject.then(result => {
console.log(Object.keys(result));
console.log(result.name)
}).catch(err => console.log(err))
运行程序的步骤:要运行应用程序,请从项目的根目录执行以下命令:
node app.js
输出:
[ 'base', 'collections', 'models', 'config', 'replica', 'options', 'otherDbs', 'relatedDbs', 'states', '_readyState', '_closeCalled', '_hasOpened', 'plugins', 'id', '_queue', '_listening', '_connectionString', '_connectionOptions', 'client', '$initialConnection', 'db', 'host', 'port', 'name' ] geeksforgeeks
示例 2:下面的示例说明了 Mongoose Connection 的基本函数asPromise()方法。在此示例中,我们使用异步函数处理 Promise。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
const URI = "mongodb://localhost:27017/geeksforgeeks"
const asPromiseExample2 = async () => {
const connectionObject =
await mongoose.createConnection(URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
}).asPromise();
console.log(Object.keys(connectionObject));
console.log(connectionObject.port)
}
asPromiseExample2();
运行程序的步骤:要运行应用程序,请从项目的根目录执行以下命令:
node app.js
输出:
[ 'base', 'collections', 'models', 'config', 'replica', 'options', 'otherDbs', 'relatedDbs', 'states', '_readyState', '_closeCalled', '_hasOpened', 'plugins', 'id', '_queue', '_listening', '_connectionString', '_connectionOptions', 'client', '$initialConnection', 'db', 'host', 'port', 'name' ] 27017
参考:https://mongoosejs.com/docs/api/connection.html#connection_Connection-asPromise
相关用法
- Mongoose Schema Connection.prototype.dropCollection()用法及代码示例
- Mongoose Schema Connection.prototype.set()用法及代码示例
- Mongoose Schema Connection.prototype.dropDatabase()用法及代码示例
- Mongoose Schema Connection.prototype.close()用法及代码示例
- Mongoose Schema Connection.prototype.useDb()用法及代码示例
- Mongoose SchemaType.prototype.ref()用法及代码示例
- Mongoose SchemaType.prototype.default()用法及代码示例
- Mongoose SchemaType.prototype.immutable()用法及代码示例
- Mongoose SchemaType.prototype.unique()用法及代码示例
- Mongoose SchemaType.prototype.validate()用法及代码示例
- Mongoose Schema.prototype.virtual()用法及代码示例
- Mongoose SchemaType.prototype.get()用法及代码示例
- Mongoose SchemaType.prototype.text()用法及代码示例
- Mongoose SchemaType.prototype.set()用法及代码示例
- Mongoose SchemaType.prototype.required()用法及代码示例
- Mongoose SchemaType.prototype.select()用法及代码示例
- Mongoose Schema.prototype.plugin()用法及代码示例
- Mongoose SchemaType.prototype.transform()用法及代码示例
- Mongoose SchemaType.prototype.index()用法及代码示例
- Mongoose Schema.prototype.static()用法及代码示例
- Mongoose Schema.prototype.pre()用法及代码示例
- Mongoose countDocuments()用法及代码示例
- Mongoose deleteMany()用法及代码示例
- Mongoose deleteOne()用法及代码示例
- Mongoose estimatedDocumentCount()用法及代码示例
注:本文由纯净天空筛选整理自sakshio0hoj大神的英文原创作品 Mongoose Schema Connection.prototype.asPromise() API。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。