Mongoose API 的 Mongoose SchemaType.prototype.default() 方法用于 SchemaType 对象。它允许我们设置模式中字段的默认值。使用此方法,我们可以以文字值或函数的形式向模式中的任何路径提供默认值。我们提供的值将根据我们提供给特定字段或路径的类型进行转换。让我们通过一个例子来理解default()方法。
用法:
schemaTypeObject.path( <path> ).default( <function/value>);
Parameters: 此方法接受一个参数,如下所述:
- function/value:它用于指定字段的默认值。
返回值:该方法返回字段设置的默认值,并为SchemaType的字段或路径设置默认值。
设置 Node.js Mongoose 模块:
步骤 1:使用以下命令创建 Node.js 应用程序:
npm init
步骤 2:创建 NodeJS 应用程序后,使用以下命令安装所需的模块:
npm install mongoose
项目结构: 项目结构将如下所示:

示例 1:下面的示例说明了 Mongoose SchemaType 的函数default()方法。在此示例中,我们将 StudentSchema 定义为具有三个属性或字段 name、age 和 rollNumber。我们在 rollNumber 路径上使用 default() 方法来返回默认值作为随机整数。最后,我们可以注意到,在创建 Student 模型的新对象时,我们没有为 rollNumber 字段提供任何值,并且在数据库中默认值,即随机整数值反映在 rollNumber 字段中。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
const URI = "mongodb://localhost:27017/geeksforgeeks"
const connectionObject = mongoose.createConnection(URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
const studentSchema = new mongoose.Schema({
name: { type: String },
age: { type: Number },
rollNumber: { type: Number },
});
studentSchema.path('rollNumber')
.default(Math.ceil(Math.random() * 100))
const Student = connectionObject
.model('Student', studentSchema);
Student.create({ name: 'Student1', age: 20 })
.then(newDocument => {
console.log(newDocument);
})
运行程序的步骤:要运行应用程序,请从项目的根目录执行以下命令:
node app.js
输出:
注意:rollNumber 字段的值可能与您不同,因为我使用的是 random() 方法,该方法在每次执行时都会返回新的整数值。
{ name: 'Student1', age: 20, rollNumber: 9, _id: new ObjectId("63a40a1065e8951038a391b1"), __v: 0 }
使用 Robo3T GUI 工具的数据库的 GUI 表示。

示例 2:在此示例中,我们使用箭头函数返回 rollNumber 字段的默认值。除此之外,我们还为年龄字段设置了默认值。最后,我们只是在创建学生模型的新文档对象时提供名称字段的值,并且我们得到了预期的结果,默认值正在填充到数据库中。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
const URI = "mongodb://localhost:27017/geeksforgeeks"
const connectionObject = mongoose.createConnection(URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
const studentSchema = new mongoose.Schema({
name: { type: String },
age: { type: Number, default: 18 },
rollNumber: { type: Number },
});
studentSchema.path('rollNumber').default(() => {
return 100 + Math.ceil(Math.random() * 100)
})
const Student = connectionObject
.model('Student', studentSchema);
const newStudent = new Student({ name: 'Student2' });
newStudent.save().then(document => {
console.log(document);
})
运行程序的步骤:要运行应用程序,请从项目的根目录执行以下命令:
node app.js
输出:
{ name: 'Student2', age: 18, _id: new ObjectId("63a40c193c8fe434f7effe5a"), rollNumber: 176, __v: 0 }
使用 Robo3T GUI 工具的数据库的 GUI 表示。
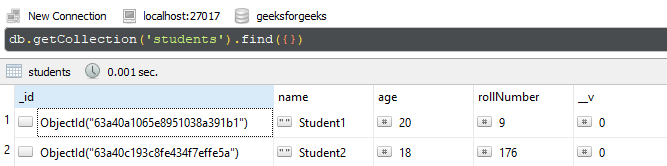
参考: https://mongoosejs.com/docs/api/schematype.html#schematype_SchemaType-default
相关用法
- Mongoose SchemaType.prototype.ref()用法及代码示例
- Mongoose SchemaType.prototype.immutable()用法及代码示例
- Mongoose SchemaType.prototype.unique()用法及代码示例
- Mongoose SchemaType.prototype.validate()用法及代码示例
- Mongoose SchemaType.prototype.get()用法及代码示例
- Mongoose SchemaType.prototype.text()用法及代码示例
- Mongoose SchemaType.prototype.set()用法及代码示例
- Mongoose SchemaType.prototype.required()用法及代码示例
- Mongoose SchemaType.prototype.select()用法及代码示例
- Mongoose SchemaType.prototype.transform()用法及代码示例
- Mongoose SchemaType.prototype.index()用法及代码示例
- Mongoose Schema Connection.prototype.asPromise()用法及代码示例
- Mongoose Schema Connection.prototype.dropCollection()用法及代码示例
- Mongoose Schema.prototype.virtual()用法及代码示例
- Mongoose Schema Connection.prototype.set()用法及代码示例
- Mongoose Schema Connection.prototype.dropDatabase()用法及代码示例
- Mongoose Schema.prototype.plugin()用法及代码示例
- Mongoose Schema Connection.prototype.close()用法及代码示例
- Mongoose Schema.prototype.static()用法及代码示例
- Mongoose Schema Connection.prototype.useDb()用法及代码示例
- Mongoose Schema.prototype.pre()用法及代码示例
- Mongoose countDocuments()用法及代码示例
- Mongoose deleteMany()用法及代码示例
- Mongoose deleteOne()用法及代码示例
- Mongoose estimatedDocumentCount()用法及代码示例
注:本文由纯净天空筛选整理自sakshio0hoj大神的英文原创作品 Mongoose SchemaType.prototype.default() API。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。