函数malloc()在 C++ 中用于分配请求的字节大小,并返回指向已分配内存的第一个字节的指针。 Amalloc()在C++中是一个在运行时分配内存的函数,因此,malloc()是一种动态内存分配技术。如果失败则返回空指针。
用法:
pointer_name = (cast-type*) malloc(size);
这里,size是一个无符号整数值(转换为size_t),它表示以字节为单位的内存块
malloc()在 C++ 中 分配 size 字节的内存块,返回指向块开头的指针。新分配的内存块的内容未初始化,保留不确定的值。 Malloc 函数存在于 <cstdlib> 头文件中。
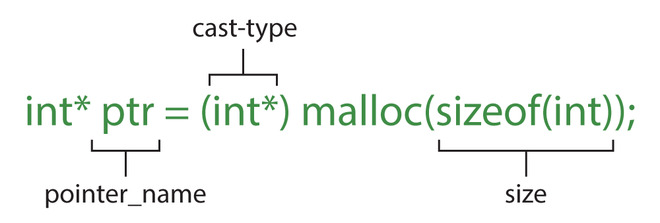
malloc() 的语法
malloc() 的返回类型
如果大小为零,则返回值取决于特定的库实现(它可能是也可能不是空指针),但返回的指针不应被取消引用。
- 空指针用于函数分配的未初始化、已初始化的内存块
- 空指针如果分配失败
内存块的工作和分配使用malloc()
示例 1:
C++
// C++ program to demonstrate working of malloc()
// cstdlib is used to use malloc function
#include <cstdlib>
#include <iostream>
using namespace std;
int main()
{
// size_t is an integer data type which can assign
// greater than or equal to 0 integer values
size_t s = 0; // s is SIZE
// malloc declaration/initialization
int* ptr = (int*)malloc(s);
// return condition if the memory block is not
// initialized
if (ptr == NULL) {
cout << "Null pointer has been returned";
}
// condition printing the message if the memory is
// initialized
else {
cout << "Memory has been allocated at address "
<< ptr << endl;
}
free(ptr);
return 0;
}
输出
Memory has been allocated at address 0x8cae70
free() 函数 在C++中用于动态地de-allocate memory 。
示例 2:
C++
// C++ program to demonstrate working of malloc()
#include <iostream>
#include<stdlib.h>
using namespace std;
int main()
{
// variable declaration
int var_len = 10;
// pointer variable declaration
int *ptr;
// allocating memory to the pointer variable using malloc()
ptr = (int*) malloc(sizeof(int)*var_len);
for(int i=0;i<var_len;i++)
{
cout << "Enter a number : " << endl;
cin >> *(ptr+i);
}
cout << "Entered elements are : " << endl;
for(int i=0;i<var_len;i++)
{
cout << *(ptr+i) << endl;
}
free(ptr);
return 0;
}
输出:
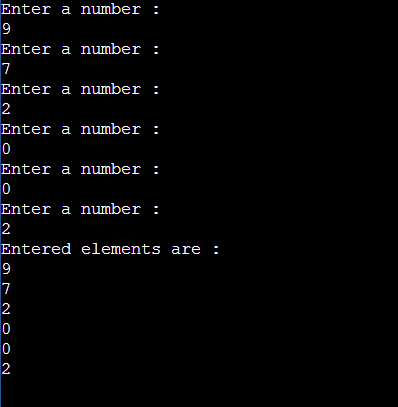
malloc()初始化的输出
Malloc应该用在什么地方?
1. 动态内存分配
动态内存分配帮助我们根据用户的需求分配一块内存。它返回一个指向该内存开头的指针,可以像数组一样对待它。
2.堆内存
malloc() 在堆上分配内存位置,并在堆栈上返回一个指针,指向正在分配的数组类型内存的起始地址,而静态数组大小对程序在任一时刻可以处理的数据量设置了硬性上限时间,无需重新编译。
3. 更长的使用寿命
使用 malloc 创建的变量或数组将存在终生,直到被清除为止。这对于 linked lists 、 binary heap 等各种数据结构非常重要。
新和malloc()之间的区别
新的 | 分配内存 |
---|---|
新是一个操作符 | malloc() 是函数 |
新调用构造函数 | malloc()不调用构造函数 |
新返回确切的数据类型 | malloc()返回空白* |
新的永远不会返回NULL(失败时会抛出) | malloc() 返回 NULL |
new 不处理内存的重新分配 | 内存的重新分配可以通过malloc来处理 |
新的分配内存并调用构造函数 | 仅分配内存分配内存 |
相关用法
- C++ malloc()用法及代码示例
- C++ map at()用法及代码示例
- C++ map begin()用法及代码示例
- C++ map cbegin()用法及代码示例
- C++ map cend()用法及代码示例
- C++ map clear()用法及代码示例
- C++ map crbegin()用法及代码示例
- C++ map crend()用法及代码示例
- C++ map empty()用法及代码示例
- C++ map end()用法及代码示例
- C++ map size()用法及代码示例
- C++ map swap()用法及代码示例
- C++ map::max_size()用法及代码示例
- C++ map count()用法及代码示例
- C++ map emplace()用法及代码示例
- C++ map emplace_hint()用法及代码示例
- C++ map equal_range()用法及代码示例
- C++ map erase()用法及代码示例
- C++ map find()用法及代码示例
- C++ map insert()用法及代码示例
- C++ map key_comp()用法及代码示例
- C++ map lower_bound()用法及代码示例
- C++ map max_size()用法及代码示例
- C++ map rbegin()用法及代码示例
- C++ map upper_bound()用法及代码示例
注:本文由纯净天空筛选整理自harsh_shokeen大神的英文原创作品 C++ malloc()。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。