本节将讨论 C 编程语言中字符串头的 strchr() 函数。 strchr() 函数用于在原始字符串中查找指定字符的第一次出现。换句话说,strchr() 函数检查原始字符串是否包含定义的字符。如果在字符串中找到该字符,则返回一个指针值;否则,它返回一个空指针。在C语言中使用strchr()函数时,需要在程序中导入<string.h>头文件。
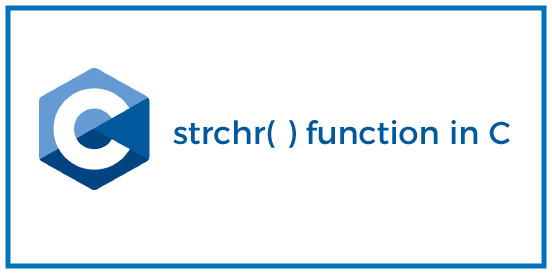
用法
char *strchr (const char *str, int c);
在上面的语法中,一个 strchr() 函数有两个参数:str 和 ch。
str:str 表示要在其中搜索字符的原始字符串。
ch:ch 是字符类型变量,表示在字符串 str 中搜索的字符。
返回值:它返回一个指针值,其中包含给定字符串中第一次出现的字符。
演示 strchr() 函数使用的程序
让我们考虑一个例子来检查给定字符串中字符的出现。
程序1.c
#include <stdio.h>
#include <string.h>
int main ()
{
const char str[] = "Use strchr() function in C.";
const char ch = 's'; // it is searched in str[] array
char *ptr; // declare character pointer ptr
printf (" Original string is:%s \n", str);
// use strchr() function and pass str in which ch is to be searched
ptr = strchr( str, ch);
printf (" The first occurrence of the '%c' in '%s' string is:'%s' ", ch, str, ptr);
return 0;
}
输出
Original string is:Use strchr() function in C. The first occurrence of the 's' in 'Use strchr() function in C.' string is:'e strchr() function in C.'
在上面的程序中,我们给strchr()函数传递了一个str参数来搜索字符's',当找到一个字符时,返回一个指针ptr值。 ptr 变量包含来自原始字符串的指定字符的值,直到 ch 变量在字符串中没有获得空字符。
使用 strchr() 函数和 if-else 语句搜索字符的程序
让我们考虑使用 strchr() 函数和 if-else 语句在 C 编程语言的给定字符串中获取第一个字符的出现的示例。
程序1.c
#include <stdio.h>
#include <string.h> // use string.h header file
int main ()
{
const char *s = "javatpoint"; // initialization of the constant char pointer
char ch; // declare a ch variable
printf (" Original string:\"%s\" \n ", s); // print the string
// take a character from the user
printf ("Please enter a character you want to search in the string:");
scanf (" %c", &ch;);
// it checks whether the specified character exists in the string
if ( strchr (s, ch) != NULL )
{
printf (" \n '%c' is found in \"%s\" ", ch, s);
}
// if the character is not found, the below statement is executed
else
printf (" \n '%c' is not found in \"%s\" ", ch, s);
return 0;
}
输出
Original string:"javatpoint" Please enter a character you want to search in the string:p 'p' is found in "javatpoint"
2nd执行:
Original string:"javatpoint" Please enter a character you want to search in the string:b 'b' is not found in "javatpoint"
在上面的程序中,我们传递了一个字符串 "javatpoint" 来搜索指定的字符。这里我们将 'p' 字符作为用户的输入并在字符串中进行搜索。之后,if 语句使用 strchr() 函数检查字符是否出现,如果存在则打印指定的字符。否则,表示在字符串中找不到该字符。
获取给定字符串中每个字符出现的程序
让我们考虑一个示例,使用 strchr() 函数和 C 编程语言的 while 循环打印给定字符串中每个字符的出现次数。
程序3.c
#include <stdio.h>
#include <string.h>
int main ()
{
// initialize a string
char str[] = " Welcome to the JavaTpoint site";
char *ptr; // declare a pointer variable
int i = 1; // declare and initialize i
// use strchr() function to check the occurrence of the character
ptr = strchr (str, 'e' );
// use while loop to checks ptr until it becomes null
while (ptr != NULL)
{
printf (" Given character 'e' found at position %d \n", (ptr - str + 1));
printf (" Occurrence of the character 'e':%d \n", i);
printf (" The occurrence of the character 'e' in the string \"%s\" is \"%s\" \n \n", str, ptr);
// use strchr() function to update the position of the string
ptr = strchr (ptr + 1, 'e');
i++;
}
return 0;
}
输出
Given character 'e' found at position 3 Occurrence of the character 'e':1 The occurrence of the character 'e' in the string " Welcome to the JavaTpoint site" is "elcome to the JavaTpoint site" Given character 'e' found at position 8 Occurrence of the character 'e':2 The occurrence of the character 'e' in the string " Welcome to the JavaTpoint site" is "e to the JavaTpoint site" Given character 'e' found at position 15 Occurrence of the character 'e':3 The occurrence of the character 'e' in the string " Welcome to the JavaTpoint site" is "e JavaTpoint site" Given character 'e' found at position 31 Occurrence of the character 'e':4 The occurrence of the character 'e' in the string " Welcome to the JavaTpoint site" is "e"
相关用法
- C语言 strcspn()用法及代码示例
- C语言 strcmpi()用法及代码示例
- C语言 strupr()用法及代码示例
- C语言 strlen()用法及代码示例
- C语言 strspn()用法及代码示例
- C语言 strrev()用法及代码示例
- C语言 strtok()、strtok_r()用法及代码示例
- C语言 strpbrk()用法及代码示例
- C语言 strnset()用法及代码示例
- C语言 strlwr()用法及代码示例
- C语言 setlinestyle()用法及代码示例
- C语言 showbits()用法及代码示例
- C语言 sprintf()用法及代码示例
- C语言 snprintf()用法及代码示例
- C语言 scanf()和gets()的区别用法及代码示例
- C语言 sector()用法及代码示例
- C语言 setviewport()用法及代码示例
- C语言 setfillstyle() and floodfill()用法及代码示例
- C语言 fread()用法及代码示例
- C语言 feof()用法及代码示例
注:本文由纯净天空筛选整理自 strchr() function in C。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。