Mongoose Document API 模型。Mongoose API 的populate() 方法用于文档模型。它允许用另一个集合中的文档替换集合中的字段。我们可以提供集合名称作为字段的值,作为填充集合及其字段的引用。让我们通过一个例子来理解populate()方法。
用法:
Model.populate( doc, options, callback );
参数:该方法接受三个参数,如下所述:
- doc:它用于指定文档名称。它可以是单个文档或文档数组。
- options:它用于配置各种属性。
- callback:它用于指定操作完成后将执行的回调函数。
返回值:此方法返回 Promise,可以使用回调函数处理该 Promise。
设置 Node.js 应用程序:
步骤 1:使用以下命令创建 Node.js 应用程序:
npm init
步骤 2:创建 NodeJS 应用程序后,使用以下命令安装所需的模块:
npm install mongoose
项目结构: 项目结构将如下所示:
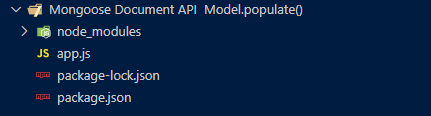
数据库结构:数据库结构如下所示,集合中存在以下文档。
- 顾客集合有以下文件:
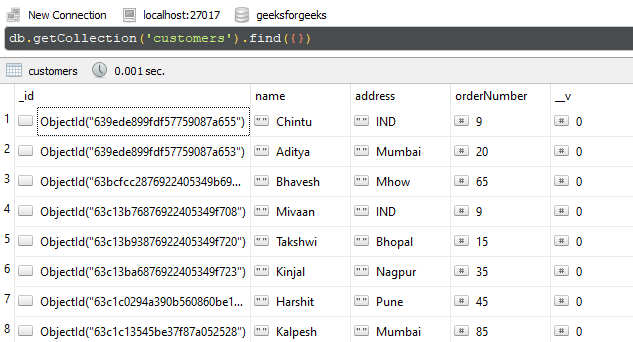
- 产品集合有以下文件:
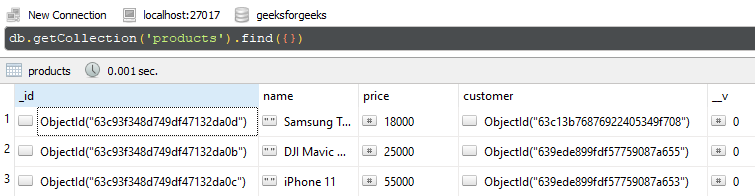
示例 1:在这个例子中,我们使用 mongoose 建立了一个数据库连接,并定义了两个模型客户模式, 和产品架构。最后,我们正在访问populate()方法超过产品模型并提供顾客模型作为指向的参考并填充两个集合中的参考文档。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
const URI = "mongodb://localhost:27017/geeksforgeeks";
let connectionObject = mongoose.createConnection(URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
let customerSchema = new mongoose.Schema({
name: String,
address: String,
orderNumber: Number,
})
let Customer =
connectionObject.model("Customer", customerSchema);
let productSchema = new mongoose.Schema({
name: String,
price: Number,
customer: {
type: mongoose.ObjectId,
ref: 'Customer'
},
})
let Product =
connectionObject.model("Product", productSchema);
Product.find().populate("customer").then(res => {
console.log(res);
});
运行程序的步骤:要运行应用程序,请从项目的根目录执行以下命令:
node app.js
输出:
[ { _id: new ObjectId("63c93f348d749df47132da0d"), name: 'Samsung TV 32', price: 18000, customer: { _id: new ObjectId("63c13b76876922405349f708"), name: 'Mivaan', address: 'IND', orderNumber: 9, __v: 0 }, __v: 0 }, { _id: new ObjectId("63c93f348d749df47132da0b"), name: 'DJI Mavic Mini 2', price: 25000, customer: { _id: new ObjectId("639ede899fdf57759087a655"), name: 'Chintu', address: 'IND', orderNumber: 9, __v: 0 }, __v: 0 }, { _id: new ObjectId("63c93f348d749df47132da0c"), name: 'iPhone 11', price: 55000, customer: { _id: new ObjectId("639ede899fdf57759087a653"), name: 'Aditya', address: 'Mumbai', orderNumber: 20, __v: 0 }, __v: 0 } ]
示例 2:在此示例中,我们正在访问populate()方法上的顾客模型。作为参考,我们提供了被视为对另一个集合的引用的路径名。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
const URI = "mongodb://localhost:27017/geeksforgeeks";
let connectionObject = mongoose.createConnection(URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
let customerSchema = new mongoose.Schema({
name: String,
address: String,
orderNumber: Number,
})
let Customer =
connectionObject.model("Customer", customerSchema);
let productSchema = new mongoose.Schema({
name: String,
price: Number,
customer: {
type: mongoose.ObjectId,
ref: 'Customer'
},
})
let Product =
connectionObject.model("Product", productSchema);
let products;
Product.find({ name: "Samsung TV 32" }).then(res => {
products = res;
Customer.populate(products, { path: "customer" }).then(res => {
console.log(res);
})
});
运行程序的步骤:要运行应用程序,请从项目的根目录执行以下命令:
node app.js
输出:
[ { _id: new ObjectId("63c93f348d749df47132da0d"), name: 'Samsung TV 32', price: 18000, customer: { _id: new ObjectId("63c13b76876922405349f708"), name: 'Mivaan', address: 'IND', orderNumber: 9, __v: 0 }, __v: 0 } ]
参考: https://mongoosejs.com/docs/api/model.html#model_Model-populate
相关用法
- Mongoose Document Model.prototype.model()用法及代码示例
- Mongoose Document Model.prototype.deleteOne()用法及代码示例
- Mongoose Document Model.prototype.remove()用法及代码示例
- Mongoose Document Model.prototype.save()用法及代码示例
- Mongoose Document Model.create()用法及代码示例
- Mongoose Document Model.deleteOne()用法及代码示例
- Mongoose Document Model.updateOne()用法及代码示例
- Mongoose Document Model.countDocuments()用法及代码示例
- Mongoose Document Model.replaceOne()用法及代码示例
- Mongoose Document Model.inspect()用法及代码示例
- Mongoose Document Model.listIndexes()用法及代码示例
- Mongoose Document Model.estimatedDocumentCount()用法及代码示例
- Mongoose Document Model.updateMany()用法及代码示例
- Mongoose Document Model.where()用法及代码示例
- Mongoose Document Model.init()用法及代码示例
- Mongoose Document Model.remove()用法及代码示例
- Mongoose Document Model.deleteMany()用法及代码示例
- Mongoose Document Model.exists()用法及代码示例
- Mongoose Document Model.insertMany()用法及代码示例
- Mongoose Document Model.count()用法及代码示例
- Mongoose Document Model.bulkWrite()用法及代码示例
- Mongoose Document prototype.getChanges()用法及代码示例
- Mongoose Document prototype.unmarkModified()用法及代码示例
- Mongoose Document prototype.replaceOne()用法及代码示例
- Mongoose Document prototype.get()用法及代码示例
注:本文由纯净天空筛选整理自kartikmukati大神的英文原创作品 Mongoose Document Model.populate() API。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。