Mongoose Document API 模型。Mongoose API 的populate() 方法用於文檔模型。它允許用另一個集合中的文檔替換集合中的字段。我們可以提供集合名稱作為字段的值,作為填充集合及其字段的引用。讓我們通過一個例子來理解populate()方法。
用法:
Model.populate( doc, options, callback );
參數:該方法接受三個參數,如下所述:
- doc:它用於指定文檔名稱。它可以是單個文檔或文檔數組。
- options:它用於配置各種屬性。
- callback:它用於指定操作完成後將執行的回調函數。
返回值:此方法返回 Promise,可以使用回調函數處理該 Promise。
設置 Node.js 應用程序:
步驟 1:使用以下命令創建 Node.js 應用程序:
npm init
步驟 2:創建 NodeJS 應用程序後,使用以下命令安裝所需的模塊:
npm install mongoose
項目結構: 項目結構將如下所示:
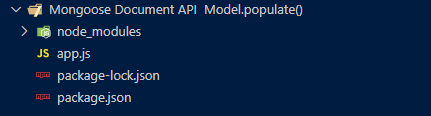
數據庫結構:數據庫結構如下所示,集合中存在以下文檔。
- 顧客集合有以下文件:
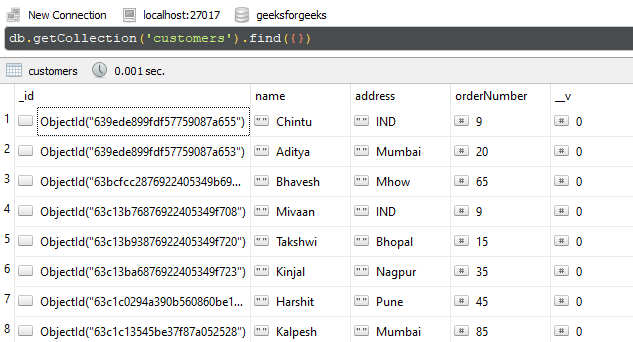
- 產品集合有以下文件:
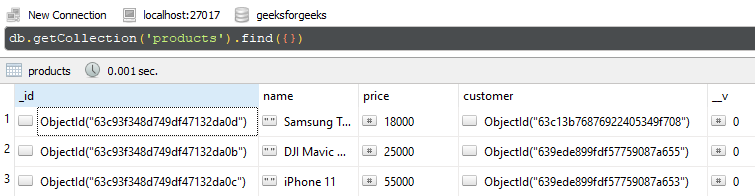
示例 1:在這個例子中,我們使用 mongoose 建立了一個數據庫連接,並定義了兩個模型客戶模式, 和產品架構。最後,我們正在訪問populate()方法超過產品模型並提供顧客模型作為指向的參考並填充兩個集合中的參考文檔。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
const URI = "mongodb://localhost:27017/geeksforgeeks";
let connectionObject = mongoose.createConnection(URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
let customerSchema = new mongoose.Schema({
name: String,
address: String,
orderNumber: Number,
})
let Customer =
connectionObject.model("Customer", customerSchema);
let productSchema = new mongoose.Schema({
name: String,
price: Number,
customer: {
type: mongoose.ObjectId,
ref: 'Customer'
},
})
let Product =
connectionObject.model("Product", productSchema);
Product.find().populate("customer").then(res => {
console.log(res);
});
運行程序的步驟:要運行應用程序,請從項目的根目錄執行以下命令:
node app.js
輸出:
[ { _id: new ObjectId("63c93f348d749df47132da0d"), name: 'Samsung TV 32', price: 18000, customer: { _id: new ObjectId("63c13b76876922405349f708"), name: 'Mivaan', address: 'IND', orderNumber: 9, __v: 0 }, __v: 0 }, { _id: new ObjectId("63c93f348d749df47132da0b"), name: 'DJI Mavic Mini 2', price: 25000, customer: { _id: new ObjectId("639ede899fdf57759087a655"), name: 'Chintu', address: 'IND', orderNumber: 9, __v: 0 }, __v: 0 }, { _id: new ObjectId("63c93f348d749df47132da0c"), name: 'iPhone 11', price: 55000, customer: { _id: new ObjectId("639ede899fdf57759087a653"), name: 'Aditya', address: 'Mumbai', orderNumber: 20, __v: 0 }, __v: 0 } ]
示例 2:在此示例中,我們正在訪問populate()方法上的顧客模型。作為參考,我們提供了被視為對另一個集合的引用的路徑名。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
const URI = "mongodb://localhost:27017/geeksforgeeks";
let connectionObject = mongoose.createConnection(URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
let customerSchema = new mongoose.Schema({
name: String,
address: String,
orderNumber: Number,
})
let Customer =
connectionObject.model("Customer", customerSchema);
let productSchema = new mongoose.Schema({
name: String,
price: Number,
customer: {
type: mongoose.ObjectId,
ref: 'Customer'
},
})
let Product =
connectionObject.model("Product", productSchema);
let products;
Product.find({ name: "Samsung TV 32" }).then(res => {
products = res;
Customer.populate(products, { path: "customer" }).then(res => {
console.log(res);
})
});
運行程序的步驟:要運行應用程序,請從項目的根目錄執行以下命令:
node app.js
輸出:
[ { _id: new ObjectId("63c93f348d749df47132da0d"), name: 'Samsung TV 32', price: 18000, customer: { _id: new ObjectId("63c13b76876922405349f708"), name: 'Mivaan', address: 'IND', orderNumber: 9, __v: 0 }, __v: 0 } ]
參考: https://mongoosejs.com/docs/api/model.html#model_Model-populate
相關用法
- Mongoose Document Model.prototype.model()用法及代碼示例
- Mongoose Document Model.prototype.deleteOne()用法及代碼示例
- Mongoose Document Model.prototype.remove()用法及代碼示例
- Mongoose Document Model.prototype.save()用法及代碼示例
- Mongoose Document Model.create()用法及代碼示例
- Mongoose Document Model.deleteOne()用法及代碼示例
- Mongoose Document Model.updateOne()用法及代碼示例
- Mongoose Document Model.countDocuments()用法及代碼示例
- Mongoose Document Model.replaceOne()用法及代碼示例
- Mongoose Document Model.inspect()用法及代碼示例
- Mongoose Document Model.listIndexes()用法及代碼示例
- Mongoose Document Model.estimatedDocumentCount()用法及代碼示例
- Mongoose Document Model.updateMany()用法及代碼示例
- Mongoose Document Model.where()用法及代碼示例
- Mongoose Document Model.init()用法及代碼示例
- Mongoose Document Model.remove()用法及代碼示例
- Mongoose Document Model.deleteMany()用法及代碼示例
- Mongoose Document Model.exists()用法及代碼示例
- Mongoose Document Model.insertMany()用法及代碼示例
- Mongoose Document Model.count()用法及代碼示例
- Mongoose Document Model.bulkWrite()用法及代碼示例
- Mongoose Document prototype.getChanges()用法及代碼示例
- Mongoose Document prototype.unmarkModified()用法及代碼示例
- Mongoose Document prototype.replaceOne()用法及代碼示例
- Mongoose Document prototype.get()用法及代碼示例
注:本文由純淨天空篩選整理自kartikmukati大神的英文原創作品 Mongoose Document Model.populate() API。非經特殊聲明,原始代碼版權歸原作者所有,本譯文未經允許或授權,請勿轉載或複製。