Flann 代表近似最近鄰的快速庫。它實際上用於係統根據數據集選擇最佳算法和最佳參數。 FlannBasedMatcher 還用於匹配或搜索一幅圖像與另一幅圖像的特征。該函數在 OpenCV 庫中可用。它用最近鄰居接近並且通常跑得比BruteForceMatcher對於各種數據集。它也適用於大型數據集。這裏,特征說明符搜索最接近的數值以進行特征匹配,並且其鄰居的距離計算很容易且快速完成。對於FlannBasedMatcher,我們需要傳遞index_params 字典和搜索參數。
該庫使用一組技術來實現其速度,包括近似k-d樹和隨機kd-trees。這些數據結構用於組織數據,使得與其他傳統方法相比可以更快地搜索最近鄰居。一般來說,FLANN 的工作原理是首先使用支持的索引方法之一構建數據集的索引。索引建立後,用戶可以通過使用新點查詢索引來執行nearest-neighbor搜索。該索引返回距查詢點最近的鄰居及其距離。隨機k-d樹和基於優先級的K-Means都是可在FLANN中使用的高效近似最近鄰搜索算法,算法的選擇取決於應用程序的具體要求。
例子:
在此示例中,使用 cv2.imread() 函數加載sub-image 和主圖像。然後使用 SIFT 檢測器檢測關鍵點並使用 sift.detectAndCompute() 函數計算查詢和訓練圖像中的說明符。然後使用 cv2.FlannBasedMatcher() 類創建 FLANN 對象。 knnMatch() 函數用於執行 k-nearest 鄰居搜索以查找兩個最近的鄰居。然後使用距離比測試過濾匹配。然後使用 cv2.drawMatches() 函數繪製良好的匹配,並使用 plt.imshow() 函數顯示結果。
This is a basic example of how to use FLANN and SIFT together in OpenCV for object detection. You can also experiment with different parameters, such as the number of nearest neighbors to search for, the algorithm used in FLANN, and the threshold value used in the distance ratio test to get the best results for specific use case. for more precision you need to use different parameters according to need.
輸入:
main_image:
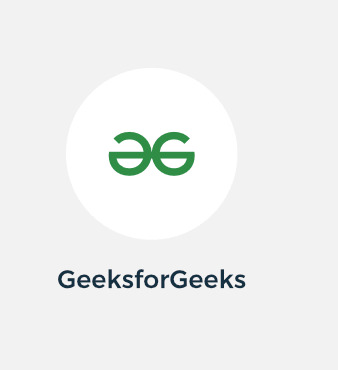
主圖
sub_image:
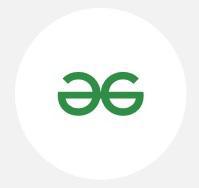
sub_image
代碼說明
- Flanned_Matcher(main_image,sub_image)。
- 找到index_params並搜索參數。
- 傳遞給 FlannBasedMatcher(index_params,search_params) 函數。
- 匹配所有相似的特征。
- 匹配所有特征後,將其存儲在另一個變量中。
- 返回存儲的圖像。
Python3
import cv2 as cv
import matplotlib.pyplot as plt
#Function returns the feature matched image
def Flanned_Matcher(main_image,sub_image):
# Initiating the SIFT detector
sift = cv.SIFT_create()
#Find the keypoints and descriptors with SIFT.
key_point1, descr1 = sift.detectAndCompute(main_image,None)
key_point2, descr2 = sift.detectAndCompute(sub_image,None)
# FLANN parameters.
FLANN_INDEX_KDTREE = 0
index_params = dict(algorithm = FLANN_INDEX_KDTREE, trees = 5)
search_params = dict(checks=50)
# FLANN based matcher with implementation of k nearest neighbour.
flann = cv.FlannBasedMatcher(index_params,search_params)
matches = flann.knnMatch(descr1,descr2,k=2)
# selecting only good matches.
matchesMask = [[0,0] for i in range(len(matches))]
# ratio test.
for i,(m,n) in enumerate(matches):
if( m.distance < 0.1*n.distance):
matchesMask[i]=[1,0]
draw_params = dict(matchColor = (0,255,0),
singlePointColor = (255,0,0),
matchesMask = matchesMask,flags = 0)
# drawing nearest neighbours
img = cv.drawMatchesKnn(main_image,
key_point1,
sub_image,
key_point2,
matches,
None,
**draw_params)
return img
# reading two input images
main_image = cv.imread('geeks-full.png')
sub_image = cv.imread('geeks-half.jpg')
#Passing two input images
output=Flanned_Matcher(main_image,sub_image)
# Save the image
cv2.imwrite('Match.jpg', output)
輸出:
True
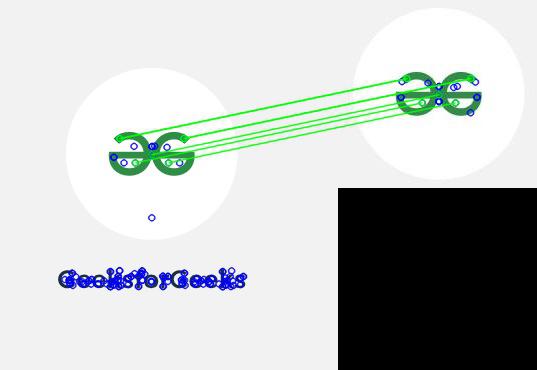
FlannBasedMatcher 輸出
輸出為 True 表示匹配的輸出圖像以名稱 Match.jpg 保存在本地文件夾中
示例 2:
代碼說明
- cv2.imread()是讀取兩個圖像的圖像文件。
- 使用 cv2.SIFT_create() 初始化 SIFT 檢測器
- 使用 SIFT 檢測器檢測兩幅圖像中的關鍵點和說明符。
- 找到 index_params 並搜索 KNN 算法的參數。
- 使用 cv2.FlannBasedMatcher(index_params,search_params) 函數初始化FlannBasedMatcher。
- 匹配兩個說明符之間 FlannBasedMatcher 相似的所有特征。
- 匹配所有特征後,將其存儲在另一個變量中,並僅在距離小於 0.5 時過濾良好匹配(這裏我們根據要求進行更改)
- 使用 cv2.drawMatchesKnn() 繪製兩個圖像之間的匹配
- 將匹配的圖像保存到本地文件夾。
Python3
import numpy as np
import cv2
# load the images
image1 = cv2.imread('Bhagavad-Gita.jpg')
image2 = cv2.imread('Geeta.jpg')
#img1 = cv2.cvtColor(img1, cv2.COLOR_BGR2GRAY)
#img2 = cv2.cvtColor(img2, cv2.COLOR_BGR2GRAY)
# Initiate SIFT detector
sift = cv2.SIFT_create()
# find the keypoints and descriptors with SIFT
keypoint1, descriptors1 = sift.detectAndCompute(image1, None)
keypoint2, descriptors2 = sift.detectAndCompute(image2, None)
# finding nearest match with KNN algorithm
index_params = dict(algorithm=0, trees=20)
search_params = dict(checks=150) # or pass empty dictionary
# Initialize the FlannBasedMatcher
flann = cv2.FlannBasedMatcher(index_params, search_params)
Matches = flann.knnMatch(descriptors1, descriptors2, k=2)
# Need to draw only good matches, so create a mask
good_matches = [[0, 0] for i in range(len(Matches))]
# Ratio Test
for i, (m, n) in enumerate(Matches):
if m.distance < 0.5*n.distance:
good_matches[i] = [1, 0]
# Draw the matches using drawMatchesKnn()
Matched = cv2.drawMatchesKnn(image1,
keypoint1,
image2,
keypoint2,
Matches,
outImg=None,
matchColor=(0, 155, 0),
singlePointColor=(0, 255, 255),
matchesMask=good_matches,
flags=0
)
# Displaying the image
cv2.imwrite('Match.jpg', Matched)
輸出:
True
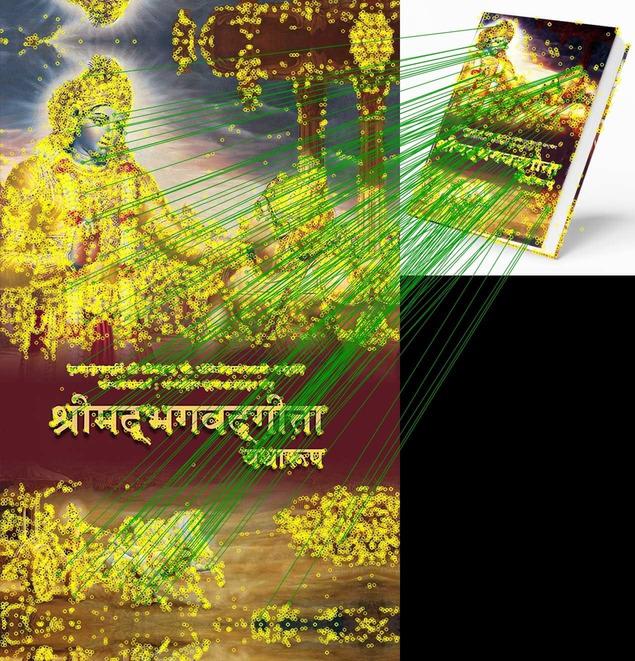
FlannBasedMatcher 輸出圖像
輸出為 True 表示匹配的輸出圖像以名稱 Match.jpg 保存在本地文件夾中
應用:
- 模式識別。
- 選擇最佳算法和參數。
- 特征匹配。
- 數據壓縮。
- 聚類分析。
- 數據庫管理係統。
- 計算幾何。
相關用法
- Python OpenCV Filter2D()用法及代碼示例
- Python OpenCV cv2.circle()用法及代碼示例
- Python OpenCV cv2.blur()用法及代碼示例
- Python OpenCV cv2.ellipse()用法及代碼示例
- Python OpenCV cv2.cvtColor()用法及代碼示例
- Python OpenCV cv2.copyMakeBorder()用法及代碼示例
- Python OpenCV cv2.imread()用法及代碼示例
- Python OpenCV cv2.imshow()用法及代碼示例
- Python OpenCV cv2.imwrite()用法及代碼示例
- Python OpenCV cv2.putText()用法及代碼示例
- Python OpenCV cv2.rectangle()用法及代碼示例
- Python OpenCV cv2.arrowedLine()用法及代碼示例
- Python OpenCV cv2.erode()用法及代碼示例
- Python OpenCV cv2.line()用法及代碼示例
- Python OpenCV cv2.flip()用法及代碼示例
- Python OpenCV cv2.transpose()用法及代碼示例
- Python OpenCV cv2.rotate()用法及代碼示例
- Python OpenCV cv2.polylines()用法及代碼示例
- Python OpenCV Canny()用法及代碼示例
- Python OpenCV destroyAllWindows()用法及代碼示例
- Python OpenCV getgaussiankernel()用法及代碼示例
- Python OpenCV getRotationMatrix2D()用法及代碼示例
- Python OpenCV getTrackbarPos()用法及代碼示例
- Python OpenCV haveImageReader()用法及代碼示例
- Python OpenCV imdecode()用法及代碼示例
注:本文由純淨天空篩選整理自dhanushrajsrmq47大神的英文原創作品 Python OpenCV – FlannBasedMatcher() Function。非經特殊聲明,原始代碼版權歸原作者所有,本譯文未經允許或授權,請勿轉載或複製。