在本文中,我們將實現Python OpenCV - BFMatcher() 函數。
先決條件:OpenCV、matplotlib
BFMatcher() 函數是什麽?
BFMatcher()函數用於特征匹配,用於將一幅圖像中的特征與另一幅圖像進行匹配。 BFMatcher 指的是一個強力匹配器,它什麽都不是,而是用於將第一組中的一個特征的說明符與第二組中的每個其他特征進行匹配的距離計算。然後返回最近的。對於第一組中的每個說明符,該匹配器通過嘗試每個說明符來找到第二組中最接近的說明符。該說明符匹配器支持屏蔽說明符集的允許匹配。因此,為了實現該函數,我們的目標是找到一幅圖像的特征集與另一幅圖像的特征集最接近的說明符。
輸入圖像示例:
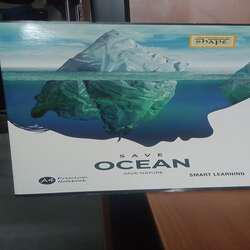
圖片1
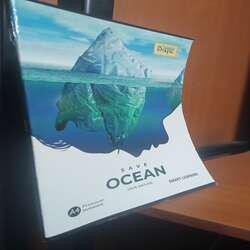
圖片2
安裝所需模塊:
要安裝必備模塊,請在命令提示符或終端中運行以下命令。
pip install opencv-python==3.4.2.16 pip install opencv-contrib-python==3.4.2.16 pip install matplotlib
代碼實現:
Python
# Importing required modules
import cv2
import matplotlib.pyplot as plt
# reading images
img1 = cv2.imread("image1.jpg")
img2 = cv2.imread("image2.jpg")
# function for feature matching
def BFMatching(img1, img2):
# Initiate SIFT detector
feat = cv2.ORB_create(5)
# find the keypoints and descriptors with SIFT
kpnt1, des1 = feat.detectAndCompute(img1, None)
kpnt2, des2 = feat.detectAndCompute(img2, None)
# BFMatcher with default parameters
bf = cv2.BFMatcher()
# finding matches from BFMatcher()
matches = bf.knnMatch(des1, des2, k=2)
# Apply ratio test
good = []
matched_image = cv2.drawMatchesKnn(img1,
kpnt1, img2, kpnt2, matches, None,
matchColor=(0, 255, 0), matchesMask=None,
singlePointColor=(255, 0, 0), flags=0)
# creating a criteria for the good matches
# and appending good matchings in good[]
for m, n in matches:
# print("m.distance is <",m.distance,">
# 1.001*n.distance is <",0.98*n.distance,">")
if m.distance < 0.98 * n.distance:
good.append([m])
# for jupyter notebook use this function
# to see output image
# plt.imshow(matched_image)
# if you are using python then run this-
cv2.imshow("matches", matched_image)
cv2.waitKey(0)
# uncomment the below section if you want to see
# the key points that are being used by the above program
print("key points of first image- ")
print(kpnt1)
print("\nkey points of second image-")
print(kpnt2)
print("\noverall features that matched by BFMatcher()-")
print(matches)
return("good features", good) # returning ggod features
BFMatching(img1, img2)
解釋:
- 在第 3 行和第 4 行中,我們將兩張圖像作為輸入。
- BFMatching方法:
- 我們創建了我們的篩檢測器“壯舉”我們將計算輸入圖像的關鍵點。在這裏,我們通過將參數 5 賦予 cv2.ORB_create() 方法,創建了用於從每個圖像中檢測 5 個關鍵點的檢測器。
- 然後我們初始化了我們的BFMatcher()具有默認參數的函數。
- df.knnMatch()方法將找到所有匹配並將它們存儲在 matches 數組中。
- 然後drawMathchesKnn()方法將繪製由創建的匹配BFMatcher()
- 接下來的循環是根據我們的興趣或標準選擇良好的匹配,在此示例中,我們假設如果圖像 1 的匹配特征的距離大於 0.98 X(圖像 1 的相應特征的距離),則該特征將是良好的。第二張圖片)並將它們存儲到數組 good[] 中。
您可以看到輸出。在下麵的輸出中,總體匹配為 5。符合我們邏輯的良好特征是否為 4。
輸出:
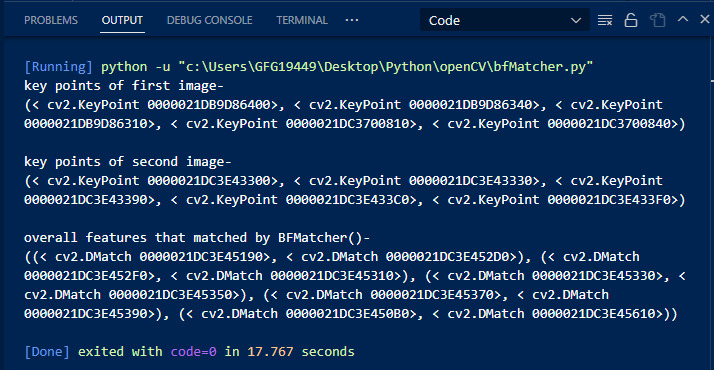
我們將看到程序繪製或顯示的輸出圖像:
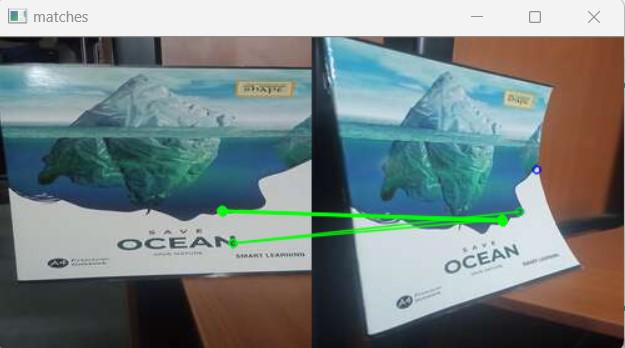
輸出
相關用法
- Python OpenCV cv2.circle()用法及代碼示例
- Python OpenCV cv2.blur()用法及代碼示例
- Python OpenCV cv2.ellipse()用法及代碼示例
- Python OpenCV cv2.cvtColor()用法及代碼示例
- Python OpenCV cv2.copyMakeBorder()用法及代碼示例
- Python OpenCV cv2.imread()用法及代碼示例
- Python OpenCV cv2.imshow()用法及代碼示例
- Python OpenCV cv2.imwrite()用法及代碼示例
- Python OpenCV cv2.putText()用法及代碼示例
- Python OpenCV cv2.rectangle()用法及代碼示例
- Python OpenCV cv2.arrowedLine()用法及代碼示例
- Python OpenCV cv2.erode()用法及代碼示例
- Python OpenCV cv2.line()用法及代碼示例
- Python OpenCV cv2.flip()用法及代碼示例
- Python OpenCV cv2.transpose()用法及代碼示例
- Python OpenCV cv2.rotate()用法及代碼示例
- Python OpenCV cv2.polylines()用法及代碼示例
- Python OpenCV Canny()用法及代碼示例
- Python OpenCV destroyAllWindows()用法及代碼示例
- Python OpenCV Filter2D()用法及代碼示例
- Python OpenCV getgaussiankernel()用法及代碼示例
- Python OpenCV getRotationMatrix2D()用法及代碼示例
- Python OpenCV getTrackbarPos()用法及代碼示例
- Python OpenCV haveImageReader()用法及代碼示例
- Python OpenCV imdecode()用法及代碼示例
注:本文由純淨天空篩選整理自anujmishraa大神的英文原創作品 Python OpenCV – BFMatcher() Function。非經特殊聲明,原始代碼版權歸原作者所有,本譯文未經允許或授權,請勿轉載或複製。