std::shared_ptr是C++11中引入的智能指针之一。与简单指针不同,它有一个关联的控制块,用于跟踪托管对象的引用计数。此引用计数在指向同一对象的 shared_ptr 实例的所有副本之间共享,确保正确的内存管理和删除。
先决条件: C++ 中的指针,C++ 中的智能指针.
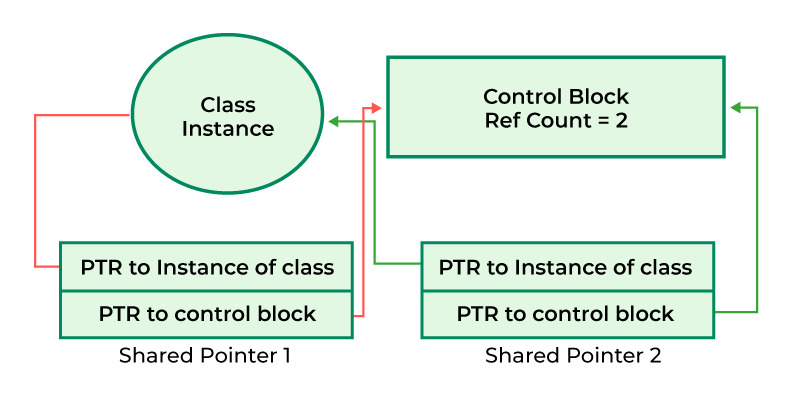
C++ 中的共享指针
std::shared_ptr 的语法
T 类型的 shared_ptr 可以声明为:
std::shared_ptr <T> ptr_name;
shared_ptr对象的初始化
我们可以使用以下方法初始化shared_ptr:
1. 使用新指针进行初始化
shared_ptr<T> ptr (new T());
shared_ptr<T> ptr = make_shared<T> (new T());
2.使用现有Pointer进行初始化
shared_ptr<T> ptr(already_existing_pointer);
shared_ptr<T> ptr = make_shared(already_existing_pointer);
shared_ptr的成员方法
以下是与shared_ptr相关的一些成员:
方法 | 说明 |
---|---|
reset() | 将 std::shared_ptr 重置为空,释放托管对象的所有权。 |
use_count() | 返回当前引用计数,指示有多少个 std::shared_ptr 实例共享所有权。 |
unique() | 检查是否只有一个 std::shared_ptr 拥有该对象(引用计数为 1)。 |
get() | 返回指向托管对象的原始指针。使用此方法时要小心。 |
交换(shr_ptr2) | 交换两个 std::shared_ptr 实例的内容(所有权)。 |
std::shared_ptr 的示例
示例 1:
C++
// C++ program to demonstrate shared_ptr
#include <iostream>
#include <memory>
using namespace std;
class A {
public:
void show() { cout << "A::show()" << endl; }
};
int main()
{
// creating a shared pointer and accessing the object
shared_ptr<A> p1(new A);
// printting the address of the managed object
cout << p1.get() << endl;
p1->show();
// creating a new shared pointer that shares ownership
shared_ptr<A> p2(p1);
p2->show();
// printing addresses of P1 and P2
cout << p1.get() << endl;
cout << p2.get() << endl;
// Returns the number of shared_ptr objects
// referring to the same managed object.
cout << p1.use_count() << endl;
cout << p2.use_count() << endl;
// Relinquishes ownership of p1 on the object
// and pointer becomes NULL
p1.reset();
cout << p1.get() << endl;
cout << p2.use_count() << endl;
cout << p2.get() << endl;
/*
These lines demonstrate that p1 no longer manages an
object (get() returns nullptr), but p2 still manages the
same object, so its reference count is 1.
*/
return 0;
}
输出
0x1365c20 A::show() A::show() 0x1365c20 0x1365c20 2 2 0 1 0x1365c20
示例 2:
C++
// C++ program to illustrate the use of make_shared
#include <iostream>
#include <memory>
using namespace std;
int main()
{
// Creating shared pointers using std::make_shared
shared_ptr<int> shr_ptr1 = make_shared<int>(42);
shared_ptr<int> shr_ptr2 = make_shared<int>(24);
// Accessing the values using the dereference operator
// (*)
cout << "Value 1: " << *shr_ptr1 << endl;
cout << "Value 2: " << *shr_ptr2 << endl;
// Using the assignment operator (=) to share ownership
shared_ptr<int> shr_ptr3 = shr_ptr1;
// Checking if shared pointer 1 and shared pointer 3
// point to the same object
if (shr_ptr1 == shr_ptr3) {
cout << "shared pointer 1 and shared pointer 3 "
"point to the same object."
<< endl;
}
// Swapping the contents of shared pointer 2 and shared
// pointer 3
shr_ptr2.swap(shr_ptr3);
// Checking the values after the swap
cout << "Value 2 (after swap): " << *shr_ptr2 << endl;
cout << "Value 3 (after swap): " << *shr_ptr3 << endl;
// Using logical operators to check if shared pointers
// are valid
if (shr_ptr1 && shr_ptr2) {
cout << "Both shared pointer 1 and shared pointer "
"2 are valid."
<< endl;
}
// Resetting a shared pointer
shr_ptr1.reset();
}
输出
Value 1: 42 Value 2: 24 shared pointer 1 and shared pointer 3 point to the same object. Value 2 (after swap): 42 Value 3 (after swap): 24 Both shared pointer 1 and shared pointer 2 are valid.
示例 3:使用 std::shared_ptr 实现链表
C++
#include <iostream>
#include <memory>
using namespace std;
// Define a singly linked list node
struct Node {
int data;
shared_ptr<Node> next;
Node(int val)
: data(val)
, next(NULL)
{
}
};
class LinkedList {
public:
LinkedList()
: head(NULL)
{
}
// Insert a new node at the end of the linked list
void insert(int val)
{
shared_ptr<Node> newNode = make_shared<Node>(val);
if (!head) {
head = newNode;
}
else {
shared_ptr<Node> current = head;
while (current->next) {
current = current->next;
}
current->next = newNode;
}
}
// Delete a node with a given value from the linked list
void del(int val)
{
if (!head) {
return;
}
if (head->data == val) {
head = head->next;
return;
}
shared_ptr<Node> current = head;
while (current->next
&& current->next->data != val) {
current = current->next;
}
if (current->next && current->next->data == val) {
current->next = current->next->next;
}
}
// Traverse and print the linked list
void Print()
{
shared_ptr<Node> current = head;
while (current) {
cout << current->data << " -> ";
current = current->next;
}
cout << "NULL" << endl;
}
private:
shared_ptr<Node> head;
};
int main()
{
LinkedList linkedList;
// Insert nodes into the linked list
linkedList.insert(1);
linkedList.insert(2);
linkedList.insert(3);
// Print the linked list
cout << "Linked List: ";
linkedList.Print();
// Delete a node and print the updated linked list
linkedList.del(2);
cout << "Linked List after deleting 2: ";
linkedList.Print();
return 0;
}
输出
Linked List: 1 -> 2 -> 3 -> NULL Linked List after deleting 2: 1 -> 3 -> NULL
相关用法
- C++ sin()用法及代码示例
- C++ sinh()用法及代码示例
- C++ scalbn()用法及代码示例
- C++ scalbln()用法及代码示例
- C++ sqrt()用法及代码示例
- C++ strtod()用法及代码示例
- C++ strtol()用法及代码示例
- C++ strtoll()用法及代码示例
- C++ strtoull()用法及代码示例
- C++ srand()用法及代码示例
- C++ strcpy()用法及代码示例
- C++ strncpy()用法及代码示例
- C++ strcat()用法及代码示例
- C++ strncat()用法及代码示例
- C++ strcmp()用法及代码示例
- C++ strncmp()用法及代码示例
- C++ strchr()用法及代码示例
- C++ strspn()用法及代码示例
- C++ strcspn()用法及代码示例
- C++ strpbrk()用法及代码示例
- C++ strrchr()用法及代码示例
- C++ strstr()用法及代码示例
- C++ strtok()用法及代码示例
- C++ strlen()用法及代码示例
- C++ strerror()用法及代码示例
注:本文由纯净天空筛选整理自yash_cse_21大神的英文原创作品 shared_ptr in C++。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。