函数签名
st.altair_chart(altair_chart, use_container_width=False)
参数 | 说明 |
---|---|
altair_chart (altair.vegalite.v2.api.Chart) | 要显示的 Altair 图表对象。 |
use_container_width (bool) | 如果为 True,则将图表宽度设置为列宽。这优先于 Altair 的原生 |
示例
import pandas as pd
import numpy as np
import altair as alt
df = pd.DataFrame(
np.random.randn(200, 3),
columns=['a', 'b', 'c'])
c = alt.Chart(df).mark_circle().encode(
x='a', y='b', size='c', color='c', tooltip=['a', 'b', 'c'])
st.altair_chart(c, use_container_width=True)
Altair 海图示例可在 https://altair-viz.github.io/gallery/ 中找到。
注释图表
Altair 还允许您使用文本、图像和表情符号对图表进行注释。您可以通过创建 layered charts 来做到这一点,它可以让您将两个不同的图表叠加在一起。这个想法是使用第一个图表来显示数据,并使用第二个图表来显示注释。然后可以使用+
运算符将第二个图表覆盖在第一个图表之上,以创建分层图表。
让我们来看一个使用文本和表情符号注释时间序列图表的示例。
第 1 步:创建基本图表
在此示例中,我们创建了一个时间序列图表来跟踪股票价格的演变。该图表是交互式的,并包含多行工具提示。单击 这里 以了解有关 Altair 中多行工具提示的更多信息。
首先,我们导入所需的库并使用
包加载示例股票数据集:vega_datasets
import altair as alt
import pandas as pd
import streamlit as st
from vega_datasets import data
# We use @st.experimental_memo to keep the dataset in cache
@st.experimental_memo
def get_data():
source = data.stocks()
source = source[source.date.gt("2004-01-01")]
return source
source = get_data()
接下来,我们定义一个函数get_chart()
来创建带有多线工具提示的股票价格的交互式时间序列图表。 x 轴代表日期,y 轴代表股票价格。
然后我们调用get_chart()
,它将股票价格 DataFrame 作为输入并返回一个图表对象。这将是我们的基础图表,我们将在其上覆盖 Step 2 中的注释。
# Define the base time-series chart.
def get_chart(data):
hover = alt.selection_single(
fields=["date"],
nearest=True,
on="mouseover",
empty="none",
)
lines = (
alt.Chart(data, title="Evolution of stock prices")
.mark_line()
.encode(
x="date",
y="price",
color="symbol",
)
)
# Draw points on the line, and highlight based on selection
points = lines.transform_filter(hover).mark_circle(size=65)
# Draw a rule at the location of the selection
tooltips = (
alt.Chart(data)
.mark_rule()
.encode(
x="yearmonthdate(date)",
y="price",
opacity=alt.condition(hover, alt.value(0.3), alt.value(0)),
tooltip=[
alt.Tooltip("date", title="Date"),
alt.Tooltip("price", title="Price (USD)"),
],
)
.add_selection(hover)
)
return (lines + points + tooltips).interactive()
chart = get_chart(source)
第 2 步:注释图表
现在我们有了第一个显示数据的图表,我们可以用文本和表情符号对其进行注释。让我们将 ⬇ 表情符号覆盖在时间序列图表顶部的特定兴趣点。我们希望用户将鼠标悬停在 ⬇ 表情符号上以查看相关的注释文本。
为简单起见,让我们注释四个特定日期并将注释的高度设置为恒定值 10
。
提示
您可以通过使用 Streamlit 小部件的输出替换 hard-coded 值来改变注释的水平和垂直位置!单击这里 跳转到下面的实时示例,并通过使用 Streamlit 小部件来培养注释的理想水平和垂直位置的直觉。
为此,我们创建了一个 DataFrame annotations_df
,其中包含日期、注释文本和注释的高度:
# Add annotations
ANNOTATIONS = [
("Mar 01, 2008", "Pretty good day for GOOG"),
("Dec 01, 2007", "Something's going wrong for GOOG & AAPL"),
("Nov 01, 2008", "Market starts again thanks to..."),
("Dec 01, 2009", "Small crash for GOOG after..."),
]
annotations_df = pd.DataFrame(ANNOTATIONS, columns=["date", "event"])
annotations_df.date = pd.to_datetime(annotations_df.date)
annotations_df["y"] = 10
使用这个 DataFrame ,我们创建了一个散点图,其中 x 轴代表日期,y 轴代表注释的高度。特定日期和高度的数据点由 ⬇ 表情符号表示,使用 Altair 的 mark_text()
mark 。
当用户将鼠标悬停在 ⬇ 表情符号上时,注释文本会显示为工具提示。这是使用 Altair 的 encode()
方法将包含注释文本的 event
列映射到绘图的视觉属性 ⬇ 来实现的。
annotation_layer = (
alt.Chart(annotations_df)
.mark_text(size=20, text="⬇", dx=-8, dy=-10, align="left")
.encode(
x="date:T",
y=alt.Y("y:Q"),
tooltip=["event"],
)
.interactive()
)
最后,我们使用+
运算符将注释覆盖在基本图表的顶部,以创建最终的分层图表! ????
st.altair_chart(
(chart + annotation_layer).interactive(),
use_container_width=True
)
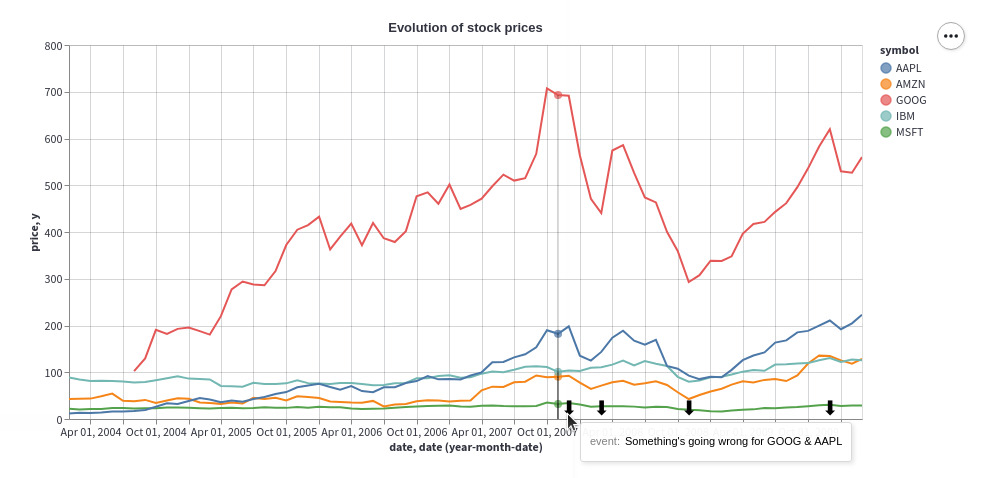
要使用图像而不是表情符号,请将包含 .mark_text()
的行替换为 .mark_image()
,并将下面的 image_url
替换为图像的 URL:
.mark_image(
width=12,
height=12,
url="image_url",
)
交互式示例
既然您已经学会了如何为图表添加注释,那就无极限了!我们扩展了上面的示例,让您可以交互式地粘贴您喜欢的表情符号并使用 Streamlit 小部件在图表上设置其位置。 ????
相关用法
- Python Streamlit st.area_chart用法及代码示例
- Python Streamlit st.audio用法及代码示例
- Python Streamlit st.experimental_singleton.clear用法及代码示例
- Python Streamlit st.bokeh_chart用法及代码示例
- Python Streamlit st.caption用法及代码示例
- Python Streamlit st.text_input用法及代码示例
- Python Streamlit st.title用法及代码示例
- Python Streamlit st.cache用法及代码示例
- Python Streamlit st.experimental_singleton用法及代码示例
- Python Streamlit st.empty用法及代码示例
- Python Streamlit st.error用法及代码示例
- Python Streamlit st.video用法及代码示例
- Python Streamlit st.vega_lite_chart用法及代码示例
- Python Streamlit st.slider用法及代码示例
- Python Streamlit st.header用法及代码示例
- Python Streamlit st.container用法及代码示例
- Python Streamlit st.form_submit_button用法及代码示例
- Python Streamlit st.form用法及代码示例
- Python Streamlit st.plotly_chart用法及代码示例
- Python Streamlit st.bar_chart用法及代码示例
- Python Streamlit st.code用法及代码示例
- Python Streamlit st.experimental_memo.clear用法及代码示例
- Python Streamlit st.warning用法及代码示例
- Python Streamlit st.image用法及代码示例
- Python Streamlit st.markdown用法及代码示例
注:本文由纯净天空筛选整理自streamlit.io大神的英文原创作品 st.altair_chart。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。