Mongoose Aggregate 原型。Mongoose API 的lookup() 方法用于执行聚合任务。它允许我们在同一数据库中的集合之间执行左连接操作,以根据需求和条件过滤出文档。让我们通过一个例子来理解lookup()方法。
用法:
aggregate.lookup( <object> );
参数:此方法接受单个参数,如下所述:
- object:它用于指定方法执行的各种属性,例如:from、localField、foreignField。
返回值:该方法以数组的形式返回聚合结果集。
设置 Node.js Mongoose 模块:
步骤 1:使用以下命令创建 Node.js 应用程序:
npm init
步骤 2:创建 NodeJS 应用程序后,使用以下命令安装所需的模块:
npm install mongoose
项目结构: 项目结构将如下所示:
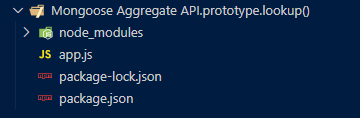
数据库结构:数据库结构如下所示,集合中存在以下文档。
- 学生作品集:
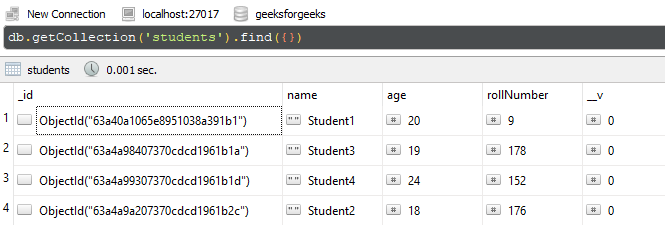
- 标记集合:
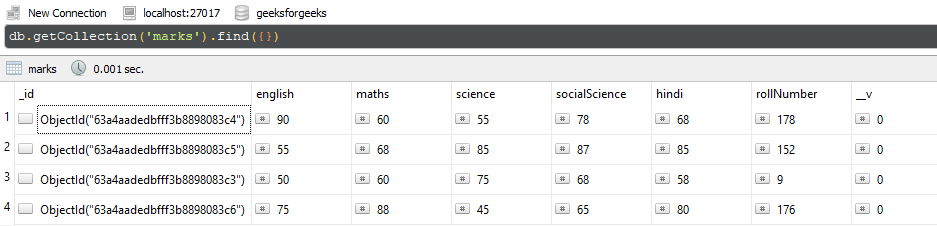
示例 1:在此示例中,我们使用 mongoose 建立了数据库连接,并通过 StudentSchema 和marksSchema 定义了模型。最后,我们通过以对象形式传递各种属性值来调用loopup()方法。我们得到了数组形式的预期结果。为了获得更好的图像,我们从结果数组中提取第一个对象。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
const URI = "mongodb://localhost:27017/geeksforgeeks"
const connectionObject = mongoose.createConnection(URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
const studentSchema = new mongoose.Schema({
name: { type: String },
age: { type: Number },
rollNumber: { type: Number },
});
const marksSchema = new mongoose.Schema({
english: Number,
maths: Number,
science: Number,
socialScience: Number,
hindi: Number,
rollNumber: Number
})
const Student = connectionObject.model('Student', studentSchema);
const Mark = connectionObject.model('Mark', marksSchema);
Student.aggregate().lookup({
from: 'marks',
localField: 'rollNumber', foreignField: 'rollNumber',
as: 'student_marks'
}).exec((error, result) => {
if (error) {
console.log('error - ', error);
} else {
console.log('result - ', result[0]);
}
})
运行程序的步骤:要运行应用程序,请从项目的根目录执行以下命令:
node app.js
输出:
result - { _id: new ObjectId("63a40a1065e8951038a391b1"), name: 'Student1', age: 20, rollNumber: 9, __v: 0, student_marks: [ { _id: new ObjectId("63a4aadedbfff3b8898083c3"), english: 50, maths: 60, science: 75, socialScience: 68, hindi: 58, rollNumber: 9, __v: 0 } ] }
示例 2:在此示例中,我们使用 mongoose 建立了数据库连接,并通过 StudentSchema 和marksSchema 定义了模型。最后,我们使用聚合管道并配置 $lookup 对象。我们得到了数组形式的预期结果。最后,我们使用 forEach 方法迭代数组并显示学生的姓名和学生的分数。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
const URI = "mongodb://localhost:27017/geeksforgeeks"
const connectionObject = mongoose.createConnection(URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
const studentSchema = new mongoose.Schema({
name: { type: String },
age: { type: Number },
rollNumber: { type: Number },
});
const marksSchema = new mongoose.Schema({
english: Number,
maths: Number,
science: Number,
socialScience: Number,
hindi: Number,
rollNumber: Number
})
const Student = connectionObject.model('Student', studentSchema);
const Mark = connectionObject.model('Mark', marksSchema);
(async () => {
const result = await Student.aggregate([{ $lookup:
{ from: 'marks', localField: 'rollNumber',
foreignField: 'rollNumber', as: 'student_marks' } }])
result.forEach(student => {
console.log(student.name)
console.log(student.student_marks);
})
})();
运行程序的步骤:要运行应用程序,请从项目的根目录执行以下命令:
node app.js
输出:
Student1 [ { _id: new ObjectId("63a4aadedbfff3b8898083c3"), english: 50, maths: 60, science: 75, socialScience: 68, hindi: 58, rollNumber: 9, __v: 0 } ] Student3 [ { _id: new ObjectId("63a4aadedbfff3b8898083c4"), english: 90, maths: 60, science: 55, socialScience: 78, hindi: 68, rollNumber: 178, __v: 0 } ] Student4 [ { _id: new ObjectId("63a4aadedbfff3b8898083c5"), english: 55, maths: 68, science: 85, socialScience: 87, hindi: 85, rollNumber: 152, __v: 0 } ] Student2 [ { _id: new ObjectId("63a4aadedbfff3b8898083c6"), english: 75, maths: 88, science: 45, socialScience: 65, hindi: 80, rollNumber: 176, __v: 0 } ]
参考: https://mongoosejs.com/docs/api/aggregate.html#aggregate_Aggregate-lookup
相关用法
- Mongoose Aggregate.prototype.limit()用法及代码示例
- Mongoose Aggregate.prototype.catch()用法及代码示例
- Mongoose Aggregate.prototype.exec()用法及代码示例
- Mongoose Aggregate.prototype.model()用法及代码示例
- Mongoose Aggregate.prototype.skip()用法及代码示例
- Mongoose Aggregate.prototype.then()用法及代码示例
- Mongoose Aggregate.prototype.append()用法及代码示例
- Mongoose Aggregate.prototype.sortByCount()用法及代码示例
- Mongoose Aggregate.prototype.project()用法及代码示例
- Mongoose Aggregate.prototype.pipeline()用法及代码示例
- Mongoose Aggregate.prototype.match()用法及代码示例
- Mongoose Aggregate.prototype.cursor()用法及代码示例
- Mongoose Aggregate.prototype.sort()用法及代码示例
- Mongoose Aggregate.prototype.addFields()用法及代码示例
- Mongoose Aggregate prototype.unionWith()用法及代码示例
- Mongoose Aggregate prototype.sample()用法及代码示例
- Mongoose Aggregate prototype.unwind()用法及代码示例
- Mongoose countDocuments()用法及代码示例
- Mongoose deleteMany()用法及代码示例
- Mongoose deleteOne()用法及代码示例
- Mongoose estimatedDocumentCount()用法及代码示例
- Mongoose exists()用法及代码示例
- Mongoose find()用法及代码示例
- Mongoose findById()用法及代码示例
- Mongoose findByIdAndDelete()用法及代码示例
注:本文由纯净天空筛选整理自sakshio0hoj大神的英文原创作品 Mongoose Aggregate.prototype.lookup() API。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。