Mongoose API 的 Aggregate API.prototype.append() 方法用于执行聚合任务。它允许我们通过添加新操作来更新或修改当前管道。使用append()方法,我们可以将新的管道操作附加到当前的聚合管道。
用法:
aggregate(...).append( <Object/ArrayObject> )
参数:该方法接受单个参数,如下所述:
- operations:该方法将操作作为对象或对象数组形式的参数。
返回值:它以数组的形式返回聚合结果集。
设置 Node.js 应用程序:
步骤 1:使用以下命令创建 Node.js 应用程序:
npm init
步骤 2:创建 NodeJS 应用程序后,使用以下命令安装所需的模块:
npm install mongoose
项目结构: 项目结构将如下所示:
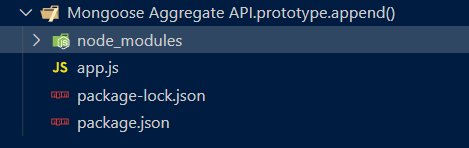
数据库结构:数据库结构如下所示,集合中存在以下文档。
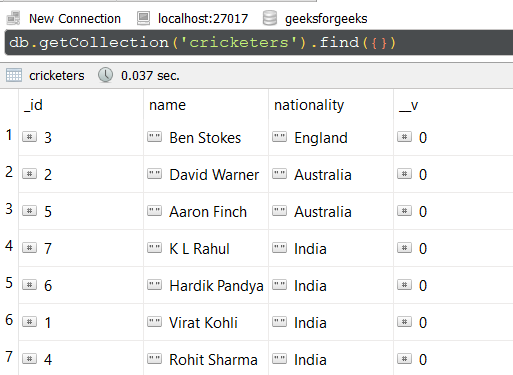
示例 1:在此示例中,我们使用 mongoose 建立了数据库连接,并通过 cricketerSchema 定义了模型,具有三列或字段 “_id”、“name” 和 “nationality”。首先,我们定义了初始管道操作项目聚合,然后我们打印当前管道状态。最后,我们使用附加了新的管道操作匹配聚合,最后使用 console.log 显示附加操作之前和之后的管道状态以及聚合结果。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
mongoose.connect(
"mongodb://localhost:27017/geeksforgeeks", {
useNewUrlParser: true,
useUnifiedTopology: true,
});
const cricketerSchema = new mongoose.Schema({
_id: Number,
name: String,
nationality: String
});
const Cricketer = mongoose.model(
'Cricketers', cricketerSchema);
const aggregate = Cricketer.aggregate();
aggregate.project({ name: 1, nationality: 1 })
const beforeAppend = aggregate.pipeline();
console.log("Before append - ", beforeAppend);
aggregate.append({ $match: { _id: 1 } })
.exec((error, success) => {
console.log("Result - ", success);
const afterAppend = aggregate.pipeline();
console.log("After append - ", afterAppend);
});
运行程序的步骤:要运行应用程序,请从项目的根目录执行以下命令:
node app.js
输出:
Before append - [ { '$project': { name: 1, nationality: 1 } } ] Result - [ { _id: 1, name: 'Virat Kohli', nationality: 'India' } ] After append - [ { '$project': { name: 1, nationality: 1 } }, { '$match': { _id: 1 } } ]
示例 2:在此示例中,不是将对象传递给append()方法中,我们传递包含管道操作的对象数组。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
mongoose.connect(
"mongodb://localhost:27017/geeksforgeeks", {
useNewUrlParser: true,
useUnifiedTopology: true,
});
const cricketerSchema = new mongoose.Schema({
_id: Number,
name: String,
nationality: String
});
const Cricketer = mongoose.model(
'Cricketers', cricketerSchema);
const aggregate = Cricketer.aggregate();
aggregate.project({ name: 1, nationality: 1 })
const beforeAppend = aggregate.pipeline();
console.log("Before append - ", beforeAppend);
const appendPipeline = [{ $match: { _id: 5 } }]
aggregate.append(appendPipeline).then((result) => {
console.log("Result - ", result);
const afterAppend = aggregate.pipeline();
console.log("After append - ", afterAppend);
});
运行程序的步骤:要运行应用程序,请从项目的根目录执行以下命令:
node app.js
输出:
Before append - [ { '$project': { name: 1, nationality: 1 } } ] Result - [ { _id: 5, name: 'Aaron Finch', nationality: 'Australia ' } ] After append - [ { '$project': { name: 1, nationality: 1 } }, { '$match': { _id: 5 } } ]
参考:https://mongoosejs.com/docs/api/aggregate.html#aggregate_Aggregate-append
相关用法
- Mongoose Aggregate.prototype.addFields()用法及代码示例
- Mongoose Aggregate.prototype.catch()用法及代码示例
- Mongoose Aggregate.prototype.exec()用法及代码示例
- Mongoose Aggregate.prototype.model()用法及代码示例
- Mongoose Aggregate.prototype.skip()用法及代码示例
- Mongoose Aggregate.prototype.limit()用法及代码示例
- Mongoose Aggregate.prototype.then()用法及代码示例
- Mongoose Aggregate.prototype.sortByCount()用法及代码示例
- Mongoose Aggregate.prototype.project()用法及代码示例
- Mongoose Aggregate.prototype.pipeline()用法及代码示例
- Mongoose Aggregate.prototype.match()用法及代码示例
- Mongoose Aggregate.prototype.cursor()用法及代码示例
- Mongoose Aggregate.prototype.sort()用法及代码示例
- Mongoose Aggregate.prototype.lookup()用法及代码示例
- Mongoose Aggregate prototype.unionWith()用法及代码示例
- Mongoose Aggregate prototype.sample()用法及代码示例
- Mongoose Aggregate prototype.unwind()用法及代码示例
- Mongoose countDocuments()用法及代码示例
- Mongoose deleteMany()用法及代码示例
- Mongoose deleteOne()用法及代码示例
- Mongoose estimatedDocumentCount()用法及代码示例
- Mongoose exists()用法及代码示例
- Mongoose find()用法及代码示例
- Mongoose findById()用法及代码示例
- Mongoose findByIdAndDelete()用法及代码示例
注:本文由纯净天空筛选整理自kartikmukati大神的英文原创作品 Mongoose Aggregate.prototype.append() API。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。