在 Java 中,Runtime 类用于与每个具有 Runtime 类单个实例的 Java 应用程序进行交互,该实例允许应用程序与应用程序运行的环境进行交互。当前运行时间可以通过getRuntime()方法获取。
Methods of Java Runtime class
方法 | 执行的操作 |
---|---|
addShutdownHook(Thread hook) | 注册一个新的virtual-machine shutdown hook 线程。 |
availableProcessors() | 返回 JVM(Java 虚拟机)可用的处理器数量 |
exec(String command) | 在单独的进程中执行给定的命令 |
执行(字符串[]命令) | 在单独的进程中执行指定的命令和参数。 |
exec(字符串命令,字符串[] envp,文件目录) | 在具有指定环境和工作目录的单独进程中执行指定的字符串命令。 |
exec(字符串命令,字符串[] envp) | 在指定环境的单独进程中执行指定的字符串命令。 |
exec(String[] cmdarray, String[] envp, 文件目录) | 在具有指定环境和工作目录的单独进程中执行指定的命令和参数。 |
exec(String[] cmdarray, String[] envp) | 在指定环境的单独进程中执行指定的命令和参数。 |
exit(int status) | 通过启动关闭序列来终止当前正在运行的 Java 虚拟机。 |
freeMemory() | 返回 JVM(Java 虚拟机)中的可用内存量 |
gc() | 运行垃圾Collector。调用此方法表明 Java 虚拟机将努力回收未使用的对象,以使它们当前占用的内存可用于快速重用。 |
getRuntime() | 返回与当前 Java 应用程序关联的实例或运行时对象 |
halt(int status) | 强制终止当前正在运行的 Java 虚拟机。此方法永远不会正常返回。使用此方法时应极其谨慎。 |
load(String filename) | 将指定的文件名加载为动态库。文件名参数必须是完整的路径名。 |
loadLibrary(String libname) | 加载指定库名的动态库。包含代码的文件是从本地系统从通常获取库文件的位置加载的。 |
maxMemory() | 返回 Java 虚拟机将尝试使用的最大内存量。如果没有固有限制,则将返回值 Long.MAX_VALUE |
removeShutdownHook(Thread hook) | De-registers 一个 previously-registered 虚拟机关闭钩子。 |
runFinalization() | 运行任何挂起终结的对象的终结方法。这表明 JVM(Java 虚拟机)将工作范围扩大到运行那些已发现已被丢弃但尚未运行 Finalize 方法的对象的 Finalize 方法。 |
totalMemory() | 返回 JVM(Java 虚拟机)中的总内存量 |
traceInstructions(boolean a) | 启用或禁用指令跟踪。如果布尔参数为 true,那么它将建议 JVM 在执行虚拟机中的每条指令时发出调试信息。 |
traceMethodCalls(boolean a) | 启用或禁用方法调用跟踪。如果布尔参数为 true,那么它将建议 Java 虚拟机在调用虚拟机中的每个方法时发出调试信息。 |
示例 1:
Java
// Java Program to Illustrate Runtime class
// Via getRuntime(), freeMemory() and
// totalMemory() Method
// Importing required classes
import java.lang.*;
import java.util.*;
// Main class
public class GFG {
// Main driver method
public static void main(String[] args)
{
// Method 1: getRuntime()
// Getting the current runtime associated
// with this process
Runtime run = Runtime.getRuntime();
// Printing the current free memory for this runtime
// using freeMemory() method
System.out.println("" + run.freeMemory());
// Method 2: freeMemory()
// Printing the number of free bytes
System.out.println(
"" + Runtime.getRuntime().freeMemory());
// Method 3: totalMemory()
// Printing the number of total bytes
System.out.println(
"" + Runtime.getRuntime().totalMemory());
}
}
输出
132579840 132285936 134217728
示例 2:
Java
// Java Program to Illustrate Runtime class
// Via exec() Method
// Importing required classes
import java.util.*;
import java.lang.*;
// Main class
public class GFG {
// Main driver method
public static void main(String[] args)
{
// Try block to check for exceptions
try {
// Creating a process and execute google-chrome
Process process = Runtime.getRuntime().exec(
"google-chrome");
System.out.println(
"Google Chrome successfully started");
}
// Catch block to handle the exceptions
catch (Exception e) {
// Display the exception on the console
e.printStackTrace();
}
}
}
输出:
Google Chrome successfully started
Note: Replace with any software you want to start. Here we work on Linux and google-chrome is written like this way only. May differ in windows/mac.
示例 3:
Java
// Java Program to Illustrate Runtime class
// Via availableProcessors() Method, exit() Method
// Importing required classes
import java.util.*;
import java.lang.*;
// Main class
public class GFG {
// Main driver method
public static void main(String[] args) {
// Method 1: availableProcessors()
// Check the number of processors available
// and printing alongside
System.out.println("" + Runtime.getRuntime()
.availableProcessors());
// Method 2: exit()
// Making program to exit
Runtime.getRuntime().exit(0);
// Nothing will run now
System.out.println("Program Running Check");
// Note: here we will notice that there will be no
// output generated on console
}
}
输出
4
从上面的输出可以清楚地看出,exit()方法不允许下面的打印语句执行,因为“Program Running Check”没有打印在控制台上。如果我们注释掉availableProcessors()的工作,可以清楚地看到exit()方法输出如下:
示例4:
Java
// Java Program to illustrate Runtime Class
// Via loadLibrary(), runFinalization()
// gc(), maxMemory()
// Class
public class GFG {
// Main driver method
public static void main(String[] args)
{
// Method 1: loadLibrary()
// Loading a library that is home/saket/Desktop
// folder
Runtime.getRuntime().loadLibrary(
"/home/saket/Desktop/Library");
System.out.println("Library Loaded Successfully");
// Method 2: runFinalization()
// Run the finalization
Runtime.getRuntime().runFinalization();
// Print statement
System.out.println("Finalized");
// Method 3: gc()
// Run the garbage collector
Runtime.getRuntime().gc();
System.out.println("Running");
// Method 4: maxMemory()
// Printing the maximum memory
System.out.println(
"" + Runtime.getRuntime().maxMemory());
}
}
输出:
Library Loaded Successfully Finalized Running 2147483648
实施例5:
Java
// Java program to illustrate halt()
// method of Runtime class
public class GFG
{
public static void main(String[] args)
{
// halt this process
Runtime.getRuntime().halt(0);
// print a string, just to see if it process is halted
System.out.println("Process is still running.");
}
}
输出:
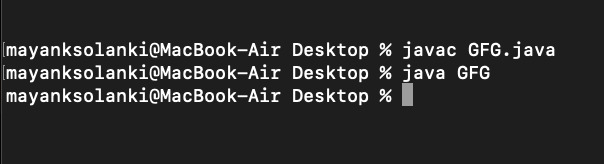
从上面的输出可以清楚地看出上面的程序已成功编译并运行。没有执行打印语句,因为我们使用了 halt() 方法来终止操作的进一步执行。
实施例6:
Java
// Java Program to Illustrate exec()
// Method of Runtime class
// Importing required classes
import java.io.*;
import java.util.*;
// Class
public class GFG {
// Main driver method
public static void main(String[] args) {
// Try block to check for exceptions
try {
// Declaring a string array
String[] cmd = new String[2];
// Initializing elements of string array
cmd[0] = "atom";
cmd[1] = "File.java";
// Creating a file with directory from local systems
File dir = new File("/Users/mayanksolanki/Desktop");
// Creating a process by creating Process class object
// and execute above array using exec() method
Process process = Runtime.getRuntime().exec(cmd, null);
// Display message on console for successful execution
System.out.println("File.java opening.");
}
// Catch block to handle exceptions
catch (Exception e) {
// Display exceptions with line number
// using printStackTrace() method
e.printStackTrace();
}
}
}
输出:
File.java opening.
相关用法
- Java Java.lang.Runtime.availableProcessors()用法及代码示例
- Java Java.lang.Runtime.exec()用法及代码示例
- Java Java.lang.Runtime.exit()用法及代码示例
- Java Java.lang.Runtime.freeMemory()用法及代码示例
- Java Java.lang.Runtime.gc()用法及代码示例
- Java Java.lang.Runtime.getRuntime()用法及代码示例
- Java Java.lang.Runtime.load()用法及代码示例
- Java Java.lang.Runtime.loadLibrary()用法及代码示例
- Java Java.lang.Runtime.maxMemory()用法及代码示例
- Java Java.lang.Runtime.removeShutdownHook()用法及代码示例
- Java Java.lang.Runtime.runFinalization()用法及代码示例
- Java Java.lang.Runtime.totalMemory()用法及代码示例
- Java Java.lang.Runtime.traceInstructions()用法及代码示例
- Java Java.lang.Runtime.traceMethodCalls()用法及代码示例
- Java Java.lang.Runtime.addShutdownHook(Thread hook)用法及代码示例
- Java Java.lang.Runtime.halt(int status)用法及代码示例
- Java Java.lang.Boolean.booleanValue()用法及代码示例
- Java Java.lang.Boolean.compareTo()用法及代码示例
- Java Java.lang.Boolean.equals()用法及代码示例
- Java Java.lang.Boolean.getBoolean()用法及代码示例
- Java Java.lang.Boolean.hashCode()用法及代码示例
- Java Java.lang.Boolean.parseBoolean()用法及代码示例
- Java Java.lang.Boolean.toString()用法及代码示例
- Java Java.lang.Boolean.valueOf()用法及代码示例
- Java Java.lang.Byte.byteValue()用法及代码示例
注:本文由纯净天空筛选整理自佚名大神的英文原创作品 Java.lang.Runtime class in Java。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。