在 Java 中,Runtime 類用於與每個具有 Runtime 類單個實例的 Java 應用程序進行交互,該實例允許應用程序與應用程序運行的環境進行交互。當前運行時間可以通過getRuntime()方法獲取。
Methods of Java Runtime class
方法 | 執行的操作 |
---|---|
addShutdownHook(Thread hook) | 注冊一個新的virtual-machine shutdown hook 線程。 |
availableProcessors() | 返回 JVM(Java 虛擬機)可用的處理器數量 |
exec(String command) | 在單獨的進程中執行給定的命令 |
執行(字符串[]命令) | 在單獨的進程中執行指定的命令和參數。 |
exec(字符串命令,字符串[] envp,文件目錄) | 在具有指定環境和工作目錄的單獨進程中執行指定的字符串命令。 |
exec(字符串命令,字符串[] envp) | 在指定環境的單獨進程中執行指定的字符串命令。 |
exec(String[] cmdarray, String[] envp, 文件目錄) | 在具有指定環境和工作目錄的單獨進程中執行指定的命令和參數。 |
exec(String[] cmdarray, String[] envp) | 在指定環境的單獨進程中執行指定的命令和參數。 |
exit(int status) | 通過啟動關閉序列來終止當前正在運行的 Java 虛擬機。 |
freeMemory() | 返回 JVM(Java 虛擬機)中的可用內存量 |
gc() | 運行垃圾Collector。調用此方法表明 Java 虛擬機將努力回收未使用的對象,以使它們當前占用的內存可用於快速重用。 |
getRuntime() | 返回與當前 Java 應用程序關聯的實例或運行時對象 |
halt(int status) | 強製終止當前正在運行的 Java 虛擬機。此方法永遠不會正常返回。使用此方法時應極其謹慎。 |
load(String filename) | 將指定的文件名加載為動態庫。文件名參數必須是完整的路徑名。 |
loadLibrary(String libname) | 加載指定庫名的動態庫。包含代碼的文件是從本地係統從通常獲取庫文件的位置加載的。 |
maxMemory() | 返回 Java 虛擬機將嘗試使用的最大內存量。如果沒有固有限製,則將返回值 Long.MAX_VALUE |
removeShutdownHook(Thread hook) | De-registers 一個 previously-registered 虛擬機關閉鉤子。 |
runFinalization() | 運行任何掛起終結的對象的終結方法。這表明 JVM(Java 虛擬機)將工作範圍擴大到運行那些已發現已被丟棄但尚未運行 Finalize 方法的對象的 Finalize 方法。 |
totalMemory() | 返回 JVM(Java 虛擬機)中的總內存量 |
traceInstructions(boolean a) | 啟用或禁用指令跟蹤。如果布爾參數為 true,那麽它將建議 JVM 在執行虛擬機中的每條指令時發出調試信息。 |
traceMethodCalls(boolean a) | 啟用或禁用方法調用跟蹤。如果布爾參數為 true,那麽它將建議 Java 虛擬機在調用虛擬機中的每個方法時發出調試信息。 |
示例 1:
Java
// Java Program to Illustrate Runtime class
// Via getRuntime(), freeMemory() and
// totalMemory() Method
// Importing required classes
import java.lang.*;
import java.util.*;
// Main class
public class GFG {
// Main driver method
public static void main(String[] args)
{
// Method 1: getRuntime()
// Getting the current runtime associated
// with this process
Runtime run = Runtime.getRuntime();
// Printing the current free memory for this runtime
// using freeMemory() method
System.out.println("" + run.freeMemory());
// Method 2: freeMemory()
// Printing the number of free bytes
System.out.println(
"" + Runtime.getRuntime().freeMemory());
// Method 3: totalMemory()
// Printing the number of total bytes
System.out.println(
"" + Runtime.getRuntime().totalMemory());
}
}
輸出
132579840 132285936 134217728
示例 2:
Java
// Java Program to Illustrate Runtime class
// Via exec() Method
// Importing required classes
import java.util.*;
import java.lang.*;
// Main class
public class GFG {
// Main driver method
public static void main(String[] args)
{
// Try block to check for exceptions
try {
// Creating a process and execute google-chrome
Process process = Runtime.getRuntime().exec(
"google-chrome");
System.out.println(
"Google Chrome successfully started");
}
// Catch block to handle the exceptions
catch (Exception e) {
// Display the exception on the console
e.printStackTrace();
}
}
}
輸出:
Google Chrome successfully started
Note: Replace with any software you want to start. Here we work on Linux and google-chrome is written like this way only. May differ in windows/mac.
示例 3:
Java
// Java Program to Illustrate Runtime class
// Via availableProcessors() Method, exit() Method
// Importing required classes
import java.util.*;
import java.lang.*;
// Main class
public class GFG {
// Main driver method
public static void main(String[] args) {
// Method 1: availableProcessors()
// Check the number of processors available
// and printing alongside
System.out.println("" + Runtime.getRuntime()
.availableProcessors());
// Method 2: exit()
// Making program to exit
Runtime.getRuntime().exit(0);
// Nothing will run now
System.out.println("Program Running Check");
// Note: here we will notice that there will be no
// output generated on console
}
}
輸出
4
從上麵的輸出可以清楚地看出,exit()方法不允許下麵的打印語句執行,因為“Program Running Check”沒有打印在控製台上。如果我們注釋掉availableProcessors()的工作,可以清楚地看到exit()方法輸出如下:
示例4:
Java
// Java Program to illustrate Runtime Class
// Via loadLibrary(), runFinalization()
// gc(), maxMemory()
// Class
public class GFG {
// Main driver method
public static void main(String[] args)
{
// Method 1: loadLibrary()
// Loading a library that is home/saket/Desktop
// folder
Runtime.getRuntime().loadLibrary(
"/home/saket/Desktop/Library");
System.out.println("Library Loaded Successfully");
// Method 2: runFinalization()
// Run the finalization
Runtime.getRuntime().runFinalization();
// Print statement
System.out.println("Finalized");
// Method 3: gc()
// Run the garbage collector
Runtime.getRuntime().gc();
System.out.println("Running");
// Method 4: maxMemory()
// Printing the maximum memory
System.out.println(
"" + Runtime.getRuntime().maxMemory());
}
}
輸出:
Library Loaded Successfully Finalized Running 2147483648
實施例5:
Java
// Java program to illustrate halt()
// method of Runtime class
public class GFG
{
public static void main(String[] args)
{
// halt this process
Runtime.getRuntime().halt(0);
// print a string, just to see if it process is halted
System.out.println("Process is still running.");
}
}
輸出:
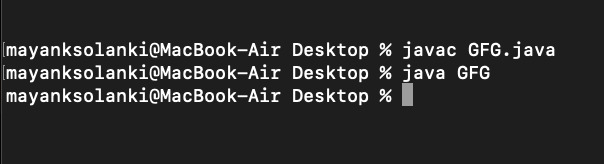
從上麵的輸出可以清楚地看出上麵的程序已成功編譯並運行。沒有執行打印語句,因為我們使用了 halt() 方法來終止操作的進一步執行。
實施例6:
Java
// Java Program to Illustrate exec()
// Method of Runtime class
// Importing required classes
import java.io.*;
import java.util.*;
// Class
public class GFG {
// Main driver method
public static void main(String[] args) {
// Try block to check for exceptions
try {
// Declaring a string array
String[] cmd = new String[2];
// Initializing elements of string array
cmd[0] = "atom";
cmd[1] = "File.java";
// Creating a file with directory from local systems
File dir = new File("/Users/mayanksolanki/Desktop");
// Creating a process by creating Process class object
// and execute above array using exec() method
Process process = Runtime.getRuntime().exec(cmd, null);
// Display message on console for successful execution
System.out.println("File.java opening.");
}
// Catch block to handle exceptions
catch (Exception e) {
// Display exceptions with line number
// using printStackTrace() method
e.printStackTrace();
}
}
}
輸出:
File.java opening.
相關用法
- Java Java.lang.Runtime.availableProcessors()用法及代碼示例
- Java Java.lang.Runtime.exec()用法及代碼示例
- Java Java.lang.Runtime.exit()用法及代碼示例
- Java Java.lang.Runtime.freeMemory()用法及代碼示例
- Java Java.lang.Runtime.gc()用法及代碼示例
- Java Java.lang.Runtime.getRuntime()用法及代碼示例
- Java Java.lang.Runtime.load()用法及代碼示例
- Java Java.lang.Runtime.loadLibrary()用法及代碼示例
- Java Java.lang.Runtime.maxMemory()用法及代碼示例
- Java Java.lang.Runtime.removeShutdownHook()用法及代碼示例
- Java Java.lang.Runtime.runFinalization()用法及代碼示例
- Java Java.lang.Runtime.totalMemory()用法及代碼示例
- Java Java.lang.Runtime.traceInstructions()用法及代碼示例
- Java Java.lang.Runtime.traceMethodCalls()用法及代碼示例
- Java Java.lang.Runtime.addShutdownHook(Thread hook)用法及代碼示例
- Java Java.lang.Runtime.halt(int status)用法及代碼示例
- Java Java.lang.Boolean.booleanValue()用法及代碼示例
- Java Java.lang.Boolean.compareTo()用法及代碼示例
- Java Java.lang.Boolean.equals()用法及代碼示例
- Java Java.lang.Boolean.getBoolean()用法及代碼示例
- Java Java.lang.Boolean.hashCode()用法及代碼示例
- Java Java.lang.Boolean.parseBoolean()用法及代碼示例
- Java Java.lang.Boolean.toString()用法及代碼示例
- Java Java.lang.Boolean.valueOf()用法及代碼示例
- Java Java.lang.Byte.byteValue()用法及代碼示例
注:本文由純淨天空篩選整理自佚名大神的英文原創作品 Java.lang.Runtime class in Java。非經特殊聲明,原始代碼版權歸原作者所有,本譯文未經允許或授權,請勿轉載或複製。