Java中的Console类的readPassword()方法有两种类型:
1. Java中Console类的readPassword()方法用于在禁用了回显的情况下从控制台读取密码或密码。
用法:
public char[] readPassword()
参数:此方法不接受任何参数。
返回值:此方法返回一个字符数组,其中包含从控制台读取的密码或口令。如果流结束,则返回null。
异常:如果发生I /O错误,则此方法将引发IOError。
注意:System.console()在在线IDE中返回null。
以下示例程序旨在说明IO包中Console类中的readPassword()方法:
程序1:
// Java program to illustrate
// Console readPassword() method
import java.io.*;
public class GFG {
public static void main(String[] args)
{
// Create the console object
Console cnsl
= System.console();
if (cnsl == null) {
System.out.println(
"No console available");
return;
}
// Read line
String str = cnsl.readLine(
"Enter username:");
// Print username
System.out.println(
"Username:" + str);
// Read password
// into character array
char[] ch = cnsl.readPassword(
"Enter password:");
// Print password
System.out.println(
"Password:" + ch);
}
}
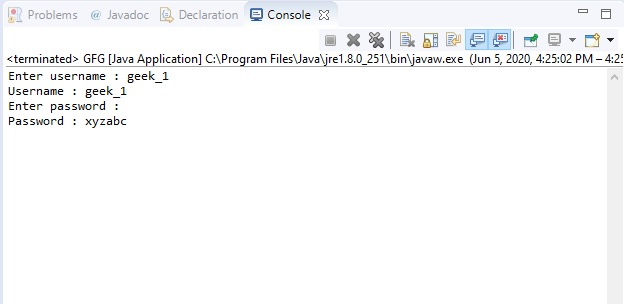
程序2:
// Java program to illustrate
// Console readPassword() method
import java.io.*;
public class GFG {
public static void main(String[] args)
{
// Create the console object
Console cnsl
= System.console();
if (cnsl == null) {
System.out.println(
"No console available");
return;
}
// Read line
String str = cnsl.readLine(
"Enter username:");
// Print username
System.out.println(
"Username:" + str);
// Read password
// into character array
char[] ch = cnsl.readPassword(
"Enter password:");
// Print password
System.out.println(
"Password:" + ch);
}
}
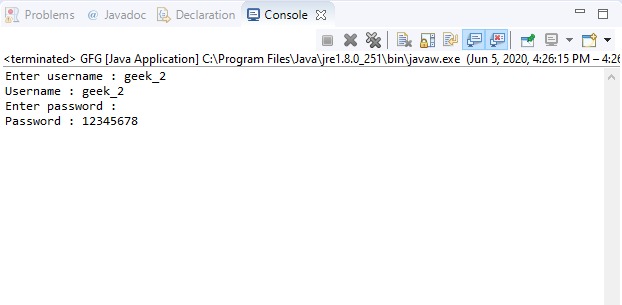
2. Java中Console类的readPassword(String,Object)方法用于通过提供格式化的提示从控制台读取密码或密码。它返回字符数组中的密码。
用法:
public char[] readPassword(String fmt,
Object... args)
参数:此方法接受两个参数:
- fmt-它表示字符串的格式。
- args-它表示由字符串格式的格式说明符引用的参数。
返回值:此方法返回包含从控制台读取的密码或密码短语的字符数组。如果流结束,则返回null。
异常:
- IllegalFormatException-如果字符串格式包含非法语法,或者格式说明符与给定参数不兼容,或者给定格式字符串或其他非法条件,则此方法将引发IllegalFormatException。
- IOError-如果发生I /O错误,则此方法将引发IOError。
以下示例程序旨在说明IO包中Console类中的readPassword(String,Object)方法:
程序1:
// Java program to illustrate
// Console readPassword(String, Object) method
import java.io.*;
public class GFG {
public static void main(String[] args)
{
// Create the console object
Console cnsl
= System.console();
if (cnsl == null) {
System.out.println(
"No console available");
return;
}
String fmt = "%2$5s %3$10s%n";
// Read line
String un = cnsl.readLine(
fmt, "Enter", "Username:");
// Print username
System.out.println(
"Username:" + un);
// Read password
// into character array
char[] pwd = cnsl.readPassword(
fmt, "Enter", "Password:");
// Print password
System.out.println(
"Password:" + pwd);
}
}
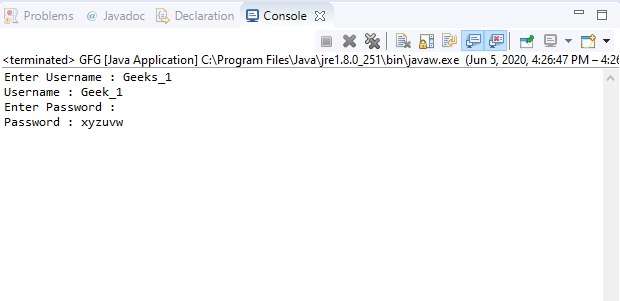
程序2:
// Java program to illustrate
// Console readPassword(String, Object) method
import java.io.*;
public class GFG {
public static void main(String[] args)
{
// Create the console object
Console cnsl
= System.console();
if (cnsl == null) {
System.out.println(
"No console available");
return;
}
String fmt = "%2$5s %3$10s%n";
// Read line
String un = cnsl.readLine(
fmt, "Enter", "Username:");
// Print username
System.out.println(
"Username:" + un);
// Read password
// into character array
char[] pwd = cnsl.readPassword(
fmt, "Enter", "Password:");
// Print password
System.out.println(
"Password:" + pwd);
}
}
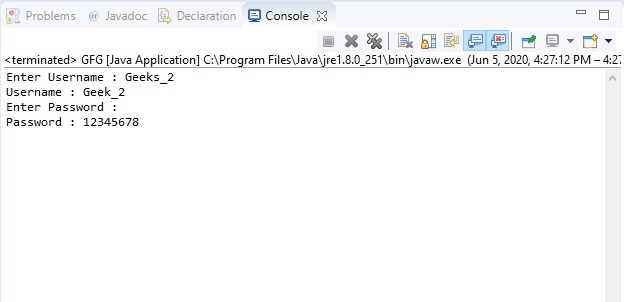
参考文献:
1. https://docs.oracle.com/javase/10/docs/api/java/io/Console.html#readPassword()
2. https://docs.oracle.com/javase/10/docs/api/java/io/Console.html#readPassword(java.lang.String, java.lang.Object…)
相关用法
- Java Console readLine()用法及代码示例
- Java Console writer()用法及代码示例
- Java Console reader()用法及代码示例
- Java Console flush()用法及代码示例
- Java Console format(String, Object)用法及代码示例
- Java Console printf(String, Object)用法及代码示例
- Java Java.io.Console用法及代码示例
- Java Java.util.Collections.disjoint()用法及代码示例
- Java Java.util.Collections.rotate()用法及代码示例
- Java Java lang.Long.numberOfLeadingZeros()用法及代码示例
- Java Java lang.Long.byteValue()用法及代码示例
- Java Java lang.Long.reverse()用法及代码示例
- Java Java lang.Long.builtcount()用法及代码示例
- Java Java lang.Long.highestOneBit()用法及代码示例
- Java Java lang.Long.numberOfTrailingZeros()用法及代码示例
- Java Java lang.Long.lowestOneBit()用法及代码示例
- Java Clock withZone()用法及代码示例
注:本文由纯净天空筛选整理自pp_pankaj大神的英文原创作品 Console readPassword() method in Java with Examples。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。