在本主题中,我们将讨论 C 编程语言中的 abs 函数。 abs()函数是stdlib.h头文件中预定义的函数,用于返回给定整数的绝对值。所以,如果我们想返回给定数字的绝对值,我们需要在C程序中实现stdlib.h头文件。 abs() 函数只返回正数。例如:假设我们有一个整数-5,我们想得到绝对数,我们使用abs()函数返回正数为5。此外,如果我们传递任何正数,它返回相同的数。
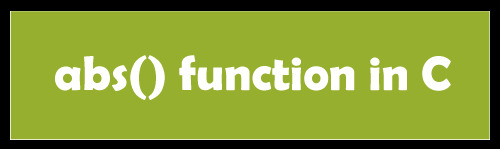
用法
int abs (int x);
在上面的语法中,x 是一个整数数据类型,它包含负数或正数并传入 abs() 函数以返回正值,因为该函数具有整数数据类型。
注意:abs() 函数总是返回一个正数,即使给定的数是负数或正数。
使用 abs() 函数获取数字绝对值的程序
让我们考虑使用 C 程序中的 abs() 函数打印绝对数的示例。
程序
#include <stdio.h>
#include <stdlib.h> // use stdlib.h header file to use abs() function.
int main()
{
int num, n; // declare the local variable
printf (" Enter a number to display the absolute value:");
scanf ("%d", #);
/* define the abs() function to convert the given number into the absolute value. */
n = abs (num);
printf ("\n The absolute value of %d is %d. ", num, n);
return 0;
}
输出
Enter a number to display the absolute value:-35 The absolute value of -35 is 35.
使用 abs() 函数打印给定整数的绝对值的程序
让我们创建一个程序来使用 C 中的 abs() 函数打印给定数字的绝对值。
绝对.c
#include <stdio.h>
#include <stdlib.h> // use stdlib.h header file to use abs() function.
#include <math.h>
int main()
{
printf (" The absolute value of 27 is %d ", abs (27));
printf (" \n The absolute value of -16 is %d ", abs (-16));
printf (" \n The absolute value of -125 is %d ", abs (-125));
printf (" \n The absolute value of 18 is %d ", abs (18));
printf (" \n The absolute value of -29 is %d ", abs (-29));
printf (" \n The absolute value of 0 is %d ", abs (0));
return 0;
}
输出
The absolute value of 27 is 27 The absolute value of -16 is 16 The absolute value of -125 is 125 The absolute value of 18 is 18 The absolute value of -29 is 29 The absolute value of 0 is 0
使用for循环打印两个整数之间的绝对值的程序
让我们考虑一个示例,在 C 程序中使用 for 循环打印两个整数之间的绝对值。
Abs2.c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
int main()
{
int i, num, last;
printf (" Enter the first number:\n ");
scanf (" %d", #);
printf ("\n Enter the last number from which you want to get the absolute number:");
scanf (" %d", &last;);
// use for loop to print the absolute number
for (i = num; i <= last; i++)
{
// abs() function convert a negative number to positive number
printf( "\n The absolute value of %d is %d. ", i, abs( i));
}
return 0;
}
输出
Enter the first negative number: -5 Enter the last number from which you want to get the absolute number: 5 The absolute value of -5 is 5. The absolute value of -4 is 4. The absolute value of -3 is 3. The absolute value of -2 is 2. The absolute value of -1 is 1. The absolute value of 0 is 0. The absolute value of 1 is 1. The absolute value of 2 is 2. The absolute value of 3 is 3. The absolute value of 4 is 4. The absolute value of 5 is 5.
不使用abs()函数获取绝对值的程序
让我们创建一个 C 程序来获取数字的绝对值,而不使用 abs() 函数。
绝对值
#include <stdio.h>
#include <stdlib.h> // use stdlib.h header file to use abs() function.
int getAbsolute (int num)
{
/* if the passed value (num) is less than 0 (zero),
the number multiplied by (-1) to return an absolute value. */
if (num < 0)
{
num = ( -1 ) * num; // given negative number multiplied by (-1)
printf (" The absolute value is:%d", num);
}
else
{
printf (" The absolute value is:%d", num);
}
return num;
}
int main()
{
int num;
printf (" Enter a number to display the absolute value:");
scanf ("%d", #);
// call the functon
getAbsolute(num);
return 0;
}
输出
Enter a number to display the absolute value:-8 The absolute value is:8
正如我们在上面的程序中看到的,我们从用户那里传递了一个整数。如果给定的数字是负数,它将乘以 (-1) 以返回正数。如果数字是正数,则返回相同的数字。
相关用法
- C语言 atan2()用法及代码示例
- C语言 asin()用法及代码示例
- C语言 atan()用法及代码示例
- C语言 acos()用法及代码示例
- C语言 assert()用法及代码示例
- C语言 arc()用法及代码示例
- C语言 asctime()、asctime_s()用法及代码示例
- C语言 fread()用法及代码示例
- C语言 feof()用法及代码示例
- C语言 imagesize()用法及代码示例
- C语言 getarcoords()用法及代码示例
- C语言 strcspn()用法及代码示例
- C语言 setlinestyle()用法及代码示例
- C语言 showbits()用法及代码示例
- C语言 sprintf()用法及代码示例
- C语言 outtextxy()用法及代码示例
- C语言 isgraph()用法及代码示例
- C语言 grapherrormsg()用法及代码示例
- C语言 moveto()用法及代码示例
- C语言 putchar()用法及代码示例
注:本文由纯净天空筛选整理自 abs() function in C。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。