Python提供了计算数字幂的函数,因此可以简化计算数字幂的任务。它在日常编程中具有许多应用程序。
天真的计算能力的方法:
# Python code to demonstrate naive method
# to compute power
n = 1
for i in range(1,5):
n=3*n
print ("The value of 3**4 is:",end="")
print (n)
输出:
The value of 3**4 is:81
使用pow()
1. float pow(x,y):此函数计算x ** y。此函数首先将其参数转换为float,然后计算幂。
Declaration: float pow(x,y) 参数: x: Number whose power has to be calculated. y: Value raised to compute power. 返回值: Returns the value x**y in float.
# Python code to demonstrate pow()
# version 1
print ("The value of 3**4 is:",end="")
# Returns 81
print (pow(3,4))
输出:
The value of 3**4 is:81.0
2. float pow(x,y,mod):此函数计算(x ** y)%mod。此函数首先将其参数转换为float,然后计算幂。
Declaration: float pow(x,y,mod) 参数: x: Number whose power has to be calculated. y: Value raised to compute power. mod: Value with which modulus has to be computed. 返回值: Returns the value (x**y) % mod in float.
# Python code to demonstrate pow()
# version 2
print ("The value of (3**4) % 10 is:",end="")
# Returns 81%10
# Returns 1
print (pow(3,4,10))
输出:
The value of (3**4) % 10 is:1
pow()中的实现案例:
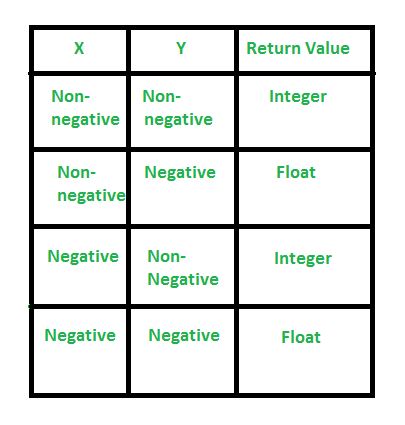
# Python code to discuss negative
# and non-negative cases
# positive x, positive y (x**y)
print("Positive x and positive y:",end="")
print(pow(4, 3))
print("Negative x and positive y:",end="")
# negative x, positive y (-x**y)
print(pow(-4, 3))
print("Positive x and negative y:",end="")
# positive x, negative y (x**-y)
print(pow(4, -3))
print("Negative x and negative y:",end="")
# negative x, negative y (-x**-y)
print(pow(-4, -3))
输出:
Positive x and positive y:64 Negative x and positive y:-64 Positive x and negative y:0.015625 Negative x and negative y:-0.015625
相关用法
注:本文由纯净天空筛选整理自 pow() in Python。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。