Node.js 是一个开源的服务器端运行时环境。读取和写入是任何应用程序的两个主要重要函数。 Node.js 提供了广泛的内置函数来执行读写操作。这fs包中包含文件操作所需的函数。
filehandle.readLines() 是 NodeJs 中的内置函数。该函数从文件中逐行读取。
用法:
filehandle.readLines();
参数:该函数没有任何参数。
返回值:该函数返回readline.InterfaceConstructor
注意:在运行此程序之前,将此文本添加到 gfg.txt 文件中。
Hey GeekforGeeks A computer science portal for geeks
示例 1:在这个例子中,我们将看到文件句柄.readLines()函数。
JavaScript
const { open } = require('node:fs/promises');
(async () => {
const file = await open('./gfg.txt');
for await (const line of file.readLines()) {
if (line.includes('science')) {
console.log(line);
}
}
})();
输出:

示例 2:在本程序中,我们将通过不同的方式使用此方法。
JavaScript
const { open } = require('node:fs/promises');
(async () => {
const file = await open('./gfg.txt');
let scienceLines = 0;
let nonScienceLines = 0;
let searchVariable = "gfg";
for await (const line of file.readLines()) {
if (line.includes(searchVariable)) {
scienceLines++;
} else {
nonScienceLines++;
}
}
console.log(`Number of lines containing
${searchVariable}: ${scienceLines}`);
console.log(`Number of lines not containing
${searchVariable}: ${nonScienceLines}`);
})();
输出:
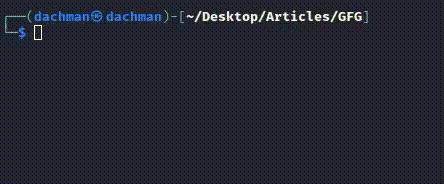
结论: filehandle.readLines() 函数用于在 Node.js 中逐行读取文件,返回一个异步迭代,允许对文件的行进行方便的迭代。通过利用 filehandle.readLines(),开发人员可以轻松地逐行处理大文件,从而实现高效的数据处理,而无需将整个文件一次性加载到内存中。当处理太大而无法完全放入内存的文件或以非阻塞方式异步处理文件时,它特别有用。
参考: https://nodejs.org/api/fs.html#filehandlereadlinesoptions
相关用法
- Node.js filehandle.readFile()用法及代码示例
- Node.js filehandle.read()用法及代码示例
- Node.js filehandle.close()用法及代码示例
- Node.js filehandle.truncate()用法及代码示例
- Node.js filehandle.appendFile()用法及代码示例
- Node.js fs.rename()用法及代码示例
- Node.js fs.statSync()用法及代码示例
- Node.js fs.truncate()用法及代码示例
- Node.js fs.mkdir()用法及代码示例
- Node.js fs.existsSync()用法及代码示例
- Node.js fs.exists()用法及代码示例
- Node.js fs.readFile()用法及代码示例
- Node.js forEach()用法及代码示例
- Node.js fs.renameSync()用法及代码示例
- Node.js fs.stat()用法及代码示例
- Node.js fs.rmdir()用法及代码示例
- Node.js fs.mkdirSync()用法及代码示例
- Node.js fs.open()用法及代码示例
- Node.js fs.realpath()用法及代码示例
- Node.js fs.mkdtempSync()用法及代码示例
- Node.js fs.createReadStream()用法及代码示例
- Node.js fs.write()用法及代码示例
- Node.js fs.openSync()用法及代码示例
- Node.js fs.rmdirSync()用法及代码示例
- Node.js fs.readdir()用法及代码示例
注:本文由纯净天空筛选整理自neeraj3304大神的英文原创作品 Node.js filehandle.readLines() Method。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。