Mongoose API 的 Mongoose Query API.prototype.read() 方法用于查询对象。它允许我们告诉MongoDB应该从哪个节点读取数据。使用此方法,我们可以设置五种不同首选项中的一种来让MongoDB读取数据。让我们通过一个例子来理解read()方法。
偏爱:
- primary: 它是默认的读取节点。如果主节点不可用,查询操作将产生错误。
- secondary: 它用于指定辅助节点首选项。如果辅助节点不可用,查询操作将产生错误。
- primaryPreferred:它用于指定 PrimaryPreferred 节点首选项。在此首选项中,如果数据可用,则从主节点读取数据,否则从辅助节点读取数据。
- secondaryPreferred:它用于指定 secondaryPreferred 节点首选项。在此偏好中,如果辅助节点可用,则从辅助节点读取数据,否则从主节点读取数据。
- nearest: 查询操作从所有最近的节点读取数据。但是,它将包括主节点和辅助节点。
用法:
query.read( <preference> );
参数:该方法接受两个参数,如下所述:
- preference:用于指定MongoDB读取数据的读取偏好。
- tags: 它用于以数组的形式指定查询操作的可选标签。
返回值:该方法返回查询对象。
设置 Node.js 应用程序:
步骤 1:使用以下命令创建 Node.js 应用程序:
npm init
步骤 2:创建 NodeJS 应用程序后,使用以下命令安装所需的模块:
npm install mongoose
项目结构: 项目结构将如下所示:
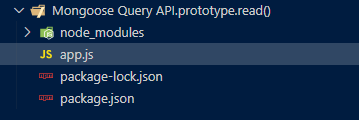
数据库结构:数据库结构如下所示,集合中存在以下文档。
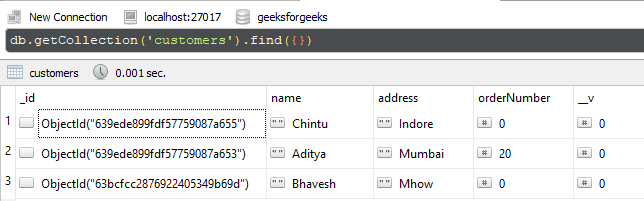
示例 1:在此示例中,我们将说明以下函数read()方法。我们将首选节点设置为“primary”节点。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
const URI = "mongodb://localhost:27017/geeksforgeeks";
let connectionObject = mongoose.createConnection(URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
let Customer = connectionObject.model(
"Customer",
new mongoose.Schema({
name: String,
address: String,
orderNumber: Number,
})
);
let query = Customer.find();
query.read("primary")
query.then((result) => {
console.log("Result -", result);
}).catch((err) => {
console.log(err);
});
运行程序的步骤:要运行应用程序,请从项目的根目录执行以下命令:
node app.js
输出:
Result - [ { _id: new ObjectId("639ede899fdf57759087a655"), name: 'Chintu', address: 'Indore', orderNumber: 0, __v: 0 }, { _id: new ObjectId("639ede899fdf57759087a653"), name: 'Aditya', address: 'Mumbai', orderNumber: 20, __v: 0 }, { _id: new ObjectId("63bcfcc2876922405349b69d"), name: 'Bhavesh', address: 'Mhow', orderNumber: 0, __v: 0 } ]
示例 2:在此示例中,我们将说明read()方法。我们将首选节点设置为“secondary”节点。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
const URI = "mongodb://localhost:27017/geeksforgeeks";
let connectionObject = mongoose.createConnection(URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
let Customer = connectionObject.model(
"Customer",
new mongoose.Schema({
name: String,
address: String,
orderNumber: Number,
})
);
let query = Customer.find({ name: "Chintu" });
query.read("secondary");
query.exec((error, result) => {
if (error) {
console.log("Error -", error);
} else {
console.log("Result -", result);
}
});
运行程序的步骤:要运行应用程序,请从项目的根目录执行以下命令:
node app.js
输出:
Result - [ { _id: new ObjectId("639ede899fdf57759087a655"), name: 'Chintu', address: 'Indore', orderNumber: 0, __v: 0 } ]
参考:https://mongoosejs.com/docs/api/query.html#query_Query-read
相关用法
- Mongoose Query.prototype.readConcern()用法及代码示例
- Mongoose Query.prototype.remove()用法及代码示例
- Mongoose Query.prototype.replaceOne()用法及代码示例
- Mongoose Query.prototype.regex()用法及代码示例
- Mongoose Query.prototype.all()用法及代码示例
- Mongoose Query.prototype.and()用法及代码示例
- Mongoose Query.prototype.batchSize()用法及代码示例
- Mongoose Query.prototype.box()用法及代码示例
- Mongoose Query.prototype.catch()用法及代码示例
- Mongoose Query.prototype.centerSphere()用法及代码示例
- Mongoose Query.prototype.circle()用法及代码示例
- Mongoose Query.prototype.collation()用法及代码示例
- Mongoose Query.prototype.cursor()用法及代码示例
- Mongoose Query.prototype.distinct()用法及代码示例
- Mongoose Query.prototype.exec()用法及代码示例
- Mongoose Query.prototype.explain()用法及代码示例
- Mongoose Query.prototype.geometry()用法及代码示例
- Mongoose Query.prototype.getOptions()用法及代码示例
- Mongoose Query.prototype.getPopulatedPaths()用法及代码示例
- Mongoose Query.prototype.getUpdate()用法及代码示例
- Mongoose Query.prototype.gt()用法及代码示例
- Mongoose Query.prototype.gte()用法及代码示例
- Mongoose Query.prototype.hint()用法及代码示例
- Mongoose Query.prototype.lean()用法及代码示例
- Mongoose Query.prototype.limit()用法及代码示例
注:本文由纯净天空筛选整理自kartikmukati大神的英文原创作品 Mongoose Query.prototype.read() API。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。