在 LINQ 中,Cast 运算符用于将集合中存在的所有元素强制转换/转换为新集合的指定数据类型。如果我们尝试转换/转换集合中不同类型的元素(字符串/整数),则转换将失败,并抛出异常。
LINQ Cast() 转换运算符的语法
C# 代码
IEnumerable<string> result = obj.Cast<string>();
LINQ 强制转换运算符示例
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp1
{
class Program
{
static void Main(string[] args)
{
//create an object named 'obj' of ArrayList
ArrayList obj = new ArrayList();
//assign the values to the object 'obj'
obj.Add("USA");
obj.Add("Australia");
obj.Add("UK");
obj.Add("India");
//Here we are converting the ArrayList object to String type of object and store the result in 'result'
IEnumerable<string> result = obj.Cast<string>();
//Now with the help of foreach loop we will print the value of result
foreach (var item in result)
{
Console.WriteLine(item);
}
Console.ReadLine();
}
}
}
输出:
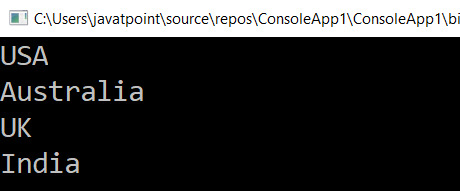
在上面的例子中,我们有一个 Arraylist,我们在其中添加了几个国家。这些国家是一个Object类型,通过Cast操作符,我们将ArrayList Object转换为String类型的对象。
相关用法
- LINQ Count()用法及代码示例
- LINQ AsEnumrable()用法及代码示例
- LINQ ToLookup()用法及代码示例
- LINQ ElementAtOrDefault()用法及代码示例
- LINQ Single()用法及代码示例
- LINQ sum()用法及代码示例
- LINQ ToArray()用法及代码示例
- LINQ Min()用法及代码示例
- LINQ ToDictionary()用法及代码示例
- LINQ LastOrDefault()用法及代码示例
- LINQ FirstOrDefault()用法及代码示例
- LINQ GroupBy()用法及代码示例
- LINQ ToList()用法及代码示例
- LINQ Max()用法及代码示例
- LINQ Aggregate()用法及代码示例
- LINQ DefaultfEmpty()用法及代码示例
- LINQ Last()用法及代码示例
- LINQ ElementAt()用法及代码示例
- LINQ OfType()用法及代码示例
- Lodash _.sampleSize()用法及代码示例
注:本文由纯净天空筛选整理自 LINQ Cast() Method。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。