Java Short 类的 parseShort() 方法用于将字符串参数解析为有符号十进制短整数。
用法
static short parseShort(String x)throws NumberFormatException
参数
x:包含要解析的短表示的字符串。字符串中的所有字符都必须是十进制数字。
返回值
它返回由十进制参数表示的短值。
异常
如果字符串不包含可解析的 short,则抛出 NumberFormatException。
例子1
public class ShortParseShortExample1 {
public static void main(String[] args) {
//with Positive String Value
String x = "100";
// it returns signed decimal short value of string
short sValue = Short.parseShort(x);
// printing signed parseShort value
System.out.println("String "+x +" , parse value into parseShort =" + sValue);
}
}
输出:
String 100 , parse value into parseShort =100
例子2
public class ShortParseShortExample2 {
public static void main(String[] args) {
//with Negative String Value
String x = "-500";
// it returns signed decimal short value of string
short sValue = Short.parseShort(x);
// printing signed parseShort value
System.out.println("String "+x +" , parse value into parseShort =" + sValue);
}
}
输出:
String -500 , parse value into parseShort =-500
例子3
public class ShortParseShortExample3 {
public static void main(String[] args) {
String x = "Hello";
// in this case, it will throw exception because String doesn't contain parsable value.
short sValue = Short.parseShort(x);
// printing signed parseShort value
System.out.println("String "+x +" , parse value into parseShort =" + sValue);
}
}
输出:
NumberFormatException
短片:
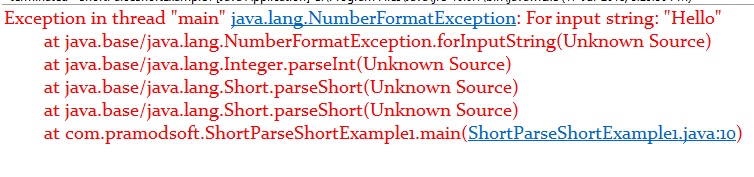
Java Short parseShort(String x, int radix) 方法
java Short 类的 parseShort(String x, int radix ) 方法用于将字符串参数解析为第二个参数指定的基数中的有符号short。
用法
static short parseShort(String x, int radix) throws NumberFormatException
参数
x:包含要解析的短的字符串。
radix:解析包含短值的字符串时要使用的基数。
返回值
它返回由指定基数中的字符串参数表示的 short。
异常
如果出现以下情况,它会抛出 NumberFormatException:
- 第一个参数为空或长度为零的字符串
- 字符串的任何字符都不是数字。
- 基数小于 Character.MIN_RADIX 或大于 Character.MAX_RADIX
- 字符串表示的值不是 short 类型的值
示例 4
public class ShortParseShortExample4 {
public static void main(String[] args) {
String x="100";
int radix=2;
// it parse string as a signed short in the radix
short shortValue = Short.parseShort(x,radix);
System.out.println(" String "+x+" parsed specified by radix "+radix + " , into signed decimal short = "+ shortValue);
}
}
输出:
String 100 parsed specified by radix 2 , into signed decimal short = 4
例 5
public class ShortParseShortExample5{
public static void main(String[] args) {
String x="abc"; //Non parsable value. throw exception
int radix=2;
// it parse string as a signed short in the radix
short shortValue = Short.parseShort(x,radix);
System.out.println(" String "+x+" parsed specified by radix "+radix + " , into signed decimal short = "+ shortValue);
}
}
输出:
NumberFormatException
短片:

例 6
字符串长度为零
public class ShortParseShortExample6 {
public static void main(String[] args) {
String x=" "; //String length is Zero . throw exception
int radix=2;
// it parse string as a signed short in the radix
short shortValue = Short.parseShort(x,radix);
System.out.println(" String "+x+" parsed specified by radix "+radix + " , into signed decimal short = "+ shortValue);
}
}
输出:
NumberFormatException
短片:

例 7
public class ShortParseShortExample7 {
public static void main(String[] args) {
// it parse string as a signed short in the radix
short shortValue = Short.parseShort("55", 6);
System.out.println(" String parsed specified by radix , into signed decimal short = "+ shortValue);
}
}
输出:
String parsed specified by radix into signed decimal short = 35
相关用法
- Java Short hashCode()用法及代码示例
- Java Short shortValue()用法及代码示例
- Java Short compare()用法及代码示例
- Java Short equals()用法及代码示例
- Java Short decode()用法及代码示例
- Java Short valueOf()用法及代码示例
- Java Short longValue()用法及代码示例
- Java Short doubleValue()用法及代码示例
- Java Short toString()用法及代码示例
- Java Short byteValue()用法及代码示例
- Java Short compareTo()用法及代码示例
- Java Short reverseBytes()用法及代码示例
- Java Short floatValue()用法及代码示例
- Java Short intValue()用法及代码示例
- Java Short compareUnsigned()用法及代码示例
- Java ShortBuffer rewind()用法及代码示例
- Java Guava Shorts.min()用法及代码示例
- Java Guava Shorts.max()用法及代码示例
- Java ShortBuffer hasArray()用法及代码示例
- Java ShortBuffer allocate()用法及代码示例
注:本文由纯净天空筛选整理自 Java Short parseShort() method。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。