在本教程中,我们将借助示例了解 Java String format() 方法。
format()
方法根据传递的参数返回格式化字符串。
示例
class Main {
public static void main(String[] args) {
String str = "Java";
// format string
String formatStr = String.format("Language: %s", str);
System.out.println(formatStr);
}
}
// Output: Language: Java
format() 语法
用法:
String.format(String str, Object... args)
这里,
format()
是一个静态方法。我们使用类名String
调用format()
方法。str
是要格式化的字符串- 上面代码中的
...
表示您可以将多个对象传递给format()
。
参数:
format()
方法采用两个参数。
- format- 格式字符串
- args- 0 个或多个参数
返回:
- 返回一个格式化的字符串
示例 1:Java 字符串 format()
class Main {
public static void main(String[] args) {
String language = "Java";
int number = 30;
String result;
// format object as a string
result = String.format("Language: %s", language);
System.out.println(result); // Language: Java
// format number as a hexadecimal number
result = String.format("Hexadecimal Number: %x", number); // 1e
System.out.println(result); // Hexadecimal Number: 1e
}
}
在上面的程序中,注意代码
result = String.format("Language: %s", language);
这里,"Language: %s"
是一个格式化字符串.
格式字符串中的 %s
替换为 language
的内容。 %s
是格式说明符。
类似地,%x
在 String.format("Number: %x", number)
中被替换为 number
的十六进制值。
格式说明符
以下是常用的格式说明符:
说明符 | 说明 |
---|---|
%b , %B |
"true" 或 "false" 基于参数 |
%s , %S |
一个字符串 |
%c , %C |
一个 Unicode 字符 |
%d |
十进制整数(仅用于整数) |
%o |
八进制整数(仅用于整数) |
%x , %X |
十六进制整数(仅用于整数) |
%e , %E |
科学记数法(用于浮点数) |
%f |
十进制数(用于浮点数) |
示例 2:数字的字符串格式
class Main {
public static void main(String[] args) {
int n1 = 47;
float n2 = 35.864f;
double n3 = 44534345.76d;
// format as an octal number
System.out.println(String.format("n1 in octal: %o", n1)); // 57
// format as hexadecimal numbers
System.out.println(String.format("n1 in hexadecimal: %x", n1)); // 2f
System.out.println(String.format("n1 in hexadecimal: %X", n1)); // 2F
// format as strings
System.out.println(String.format("n1 as string: %s", n1)); // 47
System.out.println(String.format("n2 as string: %s", n2)); // 35.864
// format in scientific notation
System.out.println(String.format("n3 in scientific notation: %g", n3)); // 4.45343e+07
}
}
输出
n1 in octal: 57 n1 in hexadecimal: 2f n1 in hexadecimal: 2F n1 as string: 47 n2 as string: 35.864 n3 in scientific notation: 4.45343e+07
示例 3:具有多个格式说明符的字符串格式
您可以在格式字符串中使用多个格式说明符。
// using more than one format specifiers
// in a format string
class Main {
public static void main(String[] args) {
int n1 = 47;
String text = "Result";
System.out.println(String.format("%s\nhexadecimal: %x", text, n1));
}
}
输出
Result hexadecimal: 2f
在这里,%s
被替换为 text
的值。同样,%o
被替换为 n1
的十六进制值。
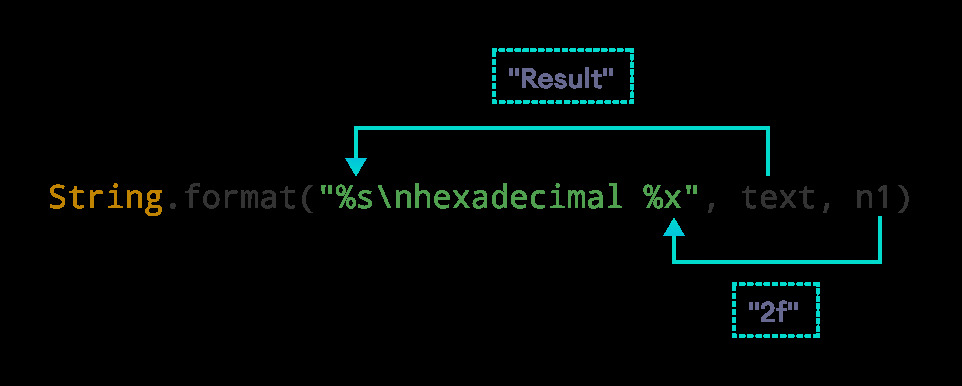
示例 4:十进制数的格式
class Main {
public static void main(String[] args) {
float n1 = -452.534f;
double n2 = -345.766d;
// format floating-point as it is
System.out.println(String.format("n1 = %f", n1)); // -452.533997
System.out.println(String.format("n2 = %f", n2)); // -345.766000
// show up to two decimal places
System.out.println(String.format("n1 = %.2f", n1)); // -452.53
System.out.println(String.format("n2 = %.2f", n2)); // -345.77
}
}
输出
n1 = -452.533997 n2 = -345.766000 n1 = -452.53 n2 = -345.77
注意:当我们格式化-452.534使用%f
,我们得到-452.533997.这不是因为format()
方法。 Java 不返回确切的浮点数的表示.
当使用%.2f
格式说明符时,format()
在小数点后给出两个数字。
示例 5:用空格和 0 填充数字
// using more than one format specifiers
// in a format string
class Main {
public static void main(String[] args) {
int n1 = 46, n2 = -46;
String result;
// padding number with spaces
// the length of the string will be 5
result = String.format("|%5d|", n1); // | 46|
System.out.println(result);
// padding number with numbers 0
// the length of the string will be 5
result = String.format("|%05d|", n1); // |00046|
System.out.println(result);
// using signs before numbers
result = String.format("%+d", n1); // +46
System.out.println(result);
result = String.format("%+d", n2); // -46
System.out.println(result);
// enclose negative number within parenthesis
// and removing the sign
result = String.format("%(d", n2); // (46)
System.out.println(result);
}
}
示例 6:在十六进制和八进制之前使用 0x 和 0
// using 0x before hexadecimal
// using 0 before octal
class Main {
public static void main(String[] args) {
int n = 46;
System.out.println(String.format("%#o", n)); // 056
System.out.println(String.format("%#x", n)); // 0x2e
}
}
带有语言环境的 Java 字符串 format()
如果您必须使用指定的 locale ,则 String format()
方法还有另一种语法。
String.format(Locale l,
String format,
Object... args)
示例 7:在 format() 中使用德语区域设置
// to use Locale
import java.util.Locale;
class Main {
public static void main(String[] args) {
int number = 8652145;
String result;
// using the current locale
result = String.format("Number: %,d", number);
System.out.println(result);
// using the GERMAN locale as the first argument
result = String.format(Locale.GERMAN, "Number in German: %,d", number);
System.out.println(result);
}
}
输出
Number: 8,652,145 Number in German: 8.652.145
注意:在德国,整数由.
代替,
.
相关用法
- Java String format()用法及代码示例
- Java String valueOf()用法及代码示例
- Java String split()用法及代码示例
- Java String strip()用法及代码示例
- Java String getChars()用法及代码示例
- Java String substring()用法及代码示例
- Java String replace()用法及代码示例
- Java String contains()用法及代码示例
- Java String regionMatches()用法及代码示例
- Java String copyValueOf()用法及代码示例
- Java String isEmpty()用法及代码示例
- Java String toString()用法及代码示例
- Java String endsWith()用法及代码示例
- Java String lines()用法及代码示例
- Java String repeat()用法及代码示例
- Java String hashCode()用法及代码示例
- Java String lastIndexOf()用法及代码示例
- Java String equals()用法及代码示例
- Java String replaceAll()用法及代码示例
- Java String startsWith()用法及代码示例
注:本文由纯净天空筛选整理自 Java String format()。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。