A Functions是一个语句块,它们通过接受一些输入并产生特定的输出来共同执行特定的任务。C++ 中的函数重写被称为在其派生类中以相同的签名(即返回类型和参数)重新定义基类函数。它属于以下类别运行时多态性。
Real-Life 函数重写示例
这个概念最好的Real-life例子是印度宪法。印度借鉴了政府机构的政治准则、结构、程序、权力和义务,规定了其他国家公民的基本权利、指导原则和义务,并自行实施;使其成为世界上最大的宪法。
另一个发展real-life 的例子可能是 RBI(印度储备银行)与其他国有银行(如 SBI、PNB、ICICI 等)之间的关系。其中 RBI 行使相同的监管职能,其他银行则按原样遵循。
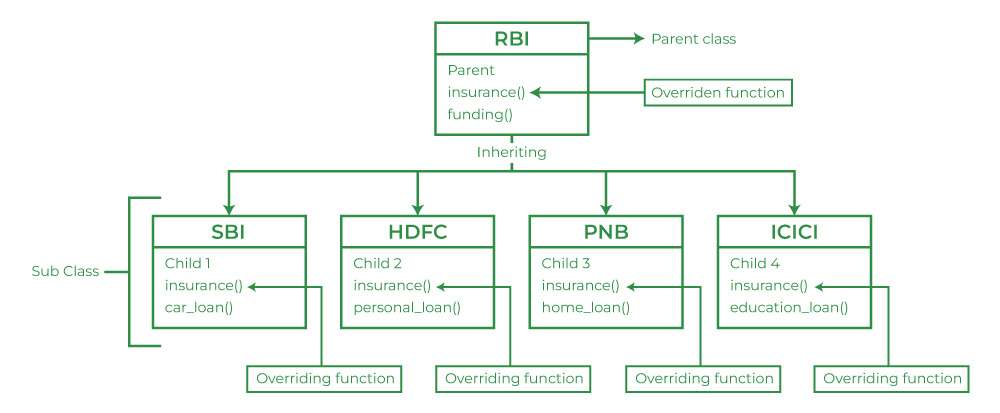
函数重写
用法:
class Parent{ access_modifier: // overridden function return_type name_of_the_function(){} }; } class child : public Parent { access_modifier: // overriding function return_type name_of_the_function(){} }; }
例子:
C++
// C++ program to demonstrate function overriding
#include <iostream>
using namespace std;
class Parent {
public:
void GeeksforGeeks_Print()
{
cout << "Base Function" << endl;
}
};
class Child : public Parent {
public:
void GeeksforGeeks_Print()
{
cout << "Derived Function" << endl;
}
};
int main()
{
Child Child_Derived;
Child_Derived.GeeksforGeeks_Print();
return 0;
}
输出
Derived Function
函数重写的变化
1.从派生类调用重写函数
C++
// C++ program to demonstrate function overriding
// by calling the overridden function
// of a member function from the child class
#include <iostream>
using namespace std;
class Parent {
public:
void GeeksforGeeks_Print()
{
cout << "Base Function" << endl;
}
};
class Child : public Parent {
public:
void GeeksforGeeks_Print()
{
cout << "Derived Function" << endl;
// call of overridden function
Parent::GeeksforGeeks_Print();
}
};
int main()
{
Child Child_Derived;
Child_Derived.GeeksforGeeks_Print();
return 0;
}
输出
Derived Function Base Function
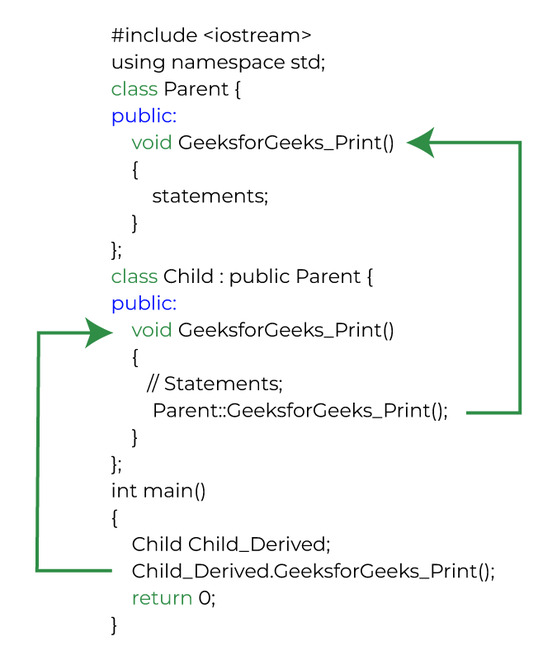
的输出从派生类调用重写函数
2. 使用指针调用重写函数
C++
// C++ program to access overridden function using pointer
// of Base type that points to an object of Derived class
#include <iostream>
using namespace std;
class Parent {
public:
void GeeksforGeeks()
{
cout << "Base Function" << endl;
}
};
class Child : public Parent {
public:
void GeeksforGeeks()
{
cout << "Derived Function" << endl;
}
};
int main()
{
Child Child_Derived;
// pointer of Parent type that points to derived1
Parent* ptr = &Child_Derived;
// call function of Base class using ptr
ptr->GeeksforGeeks();
return 0;
}
输出
Base Function
3. 重写函数对基类的访问
C++
// C++ program to access overridden function
// in main() using the scope resolution operator ::
#include <iostream>
using namespace std;
class Parent {
public:
void GeeksforGeeks()
{
cout << "Base Function" << endl;
}
};
class Child : public Parent {
public:
void GeeksforGeeks()
{
cout << "Derived Function" << endl;
}
};
int main()
{
Child Child_Derived;
Child_Derived.GeeksforGeeks();
// access GeeksforGeeks() function of the Base class
Child_Derived.Parent::GeeksforGeeks();
return 0;
}
输出
Derived Function Base Function
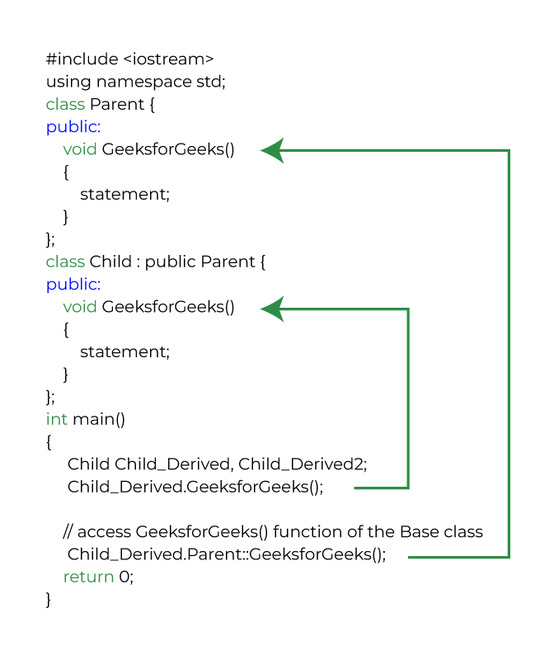
重写函数对基类的访问
4. 访问重写函数
C++
// C++ Program Demonstrating
// Accessing of Overridden Function
#include <iostream>
using namespace std;
// defining of the Parent class
class Parent
{
public:
// defining the overridden function
void GeeksforGeeks_Print()
{
cout << "I am the Parent class function" << endl;
}
};
// defining of the derived class
class Child : public Parent
{
public:
// defining of the overriding function
void GeeksforGeeks_Print()
{
cout << "I am the Child class function" << endl;
}
};
int main()
{
// create instances of the derived class
Child GFG1, GFG2;
// call the overriding function
GFG1.GeeksforGeeks_Print();
// call the overridden function of the Base class
GFG2.Parent::GeeksforGeeks_Print();
return 0;
}
输出
I am the Child class function I am the Parent class function
函数重载与函数重写
Function Overloading |
Function Overriding |
---|---|
它属于编译时多态性 | 它属于运行时多态性 |
一个函数可以被重载多次,因为它是在编译时解析的 | 函数不能被重写多次,因为它是在运行时解析的 |
无需继承即可执行 | 没有继承就无法执行 |
他们在同一个范围内 | 它们的范围不同。 |
要了解更多信息,您可以参考 函数重载 VS 函数重写.
相关用法
- C++ Function Overloading用法及代码示例
- C++ Function Pointer用法及代码示例
- C++ 函数用法及代码示例
- C++ Forward_list forward_list()用法及代码示例
- C++ Forward_list assign()用法及代码示例
- C++ Forward_list before_begin()用法及代码示例
- C++ Forward_list begin()用法及代码示例
- C++ Forward_list cbefore_begin()用法及代码示例
- C++ Forward_list cbegin()用法及代码示例
- C++ Forward_list cend()用法及代码示例
- C++ Forward_list clear()用法及代码示例
- C++ Forward_list emplace_after()用法及代码示例
- C++ Forward_list emplace_front()用法及代码示例
- C++ Forward_list empty()用法及代码示例
- C++ Forward_list end()用法及代码示例
- C++ Forward_list erase_after()用法及代码示例
- C++ Forward_list front()用法及代码示例
- C++ Forward_list get_allocator()用法及代码示例
- C++ Forward_list insert_after()用法及代码示例
- C++ Forward_list max_size()用法及代码示例
- C++ Forward_list merge()用法及代码示例
- C++ Forward_list pop_front()用法及代码示例
- C++ Forward_list push_front()用法及代码示例
- C++ Forward_list remove()用法及代码示例
- C++ Forward_list remove_if()用法及代码示例
注:本文由纯净天空筛选整理自harsh_shokeen大神的英文原创作品 Function Overriding in C++。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。