Mongoose API 的 Aggregate API.prototype.unwind() 方法用於執行聚合任務。它允許我們分解 MongoDB 數據庫或架構中的複雜嵌套數組或文檔字段。它為字段中存在的每個元素返回一個文檔對象。它解構數組屬性,為數組中的每個元素輸出一個文檔對象。
用法:
aggregate.unwind(field)
參數:該方法接受如上所述和如下所述的單個參數:
- field:它是MongoDB 數據庫中的字段或屬性。
返回值:此方法為數組字段中的每個元素返回一個文檔對象。
設置 Node.js 應用程序:
步驟 1:使用以下命令創建 Node.js 應用程序:
npm init
步驟 2:創建 NodeJS 應用程序後,使用以下命令安裝所需的模塊:
npm install mongoose
項目結構: 項目結構將如下所示:

數據庫結構:數據庫結構如下所示,集合中存在以下文檔。
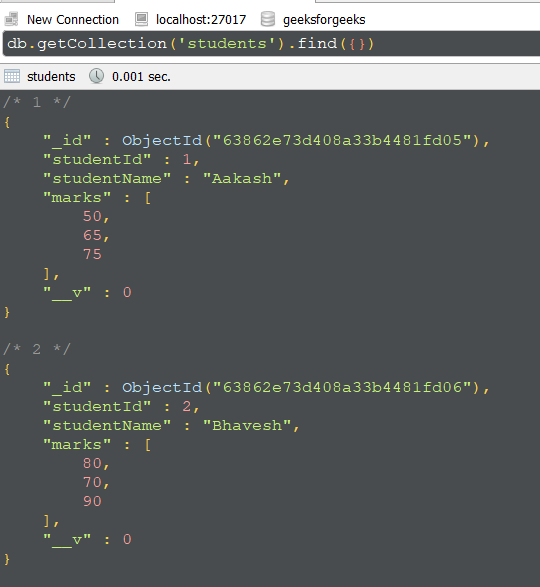
示例 1:在此示例中,我們使用 mongoose 建立了數據庫連接,並通過 StudentSchema 定義了模型,具有三個列或字段 “studentId”、“studentName” 和 “marks”。最後,我們使用unwind()方法 在分數字段來解構數組並獲取數組中每個元素的文檔作為輸出。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
mongoose.connect("mongodb://localhost:27017/geeksforgeeks", {
useNewUrlParser: true,
useUnifiedTopology: true,
});
const studentSchema = new mongoose.Schema({
studentId: Number,
studentName: String,
marks: []
});
const Student = mongoose.model('Student', studentSchema);
const aggregate = Student.aggregate();
aggregate.unwind("$marks").exec((err, result) => {
if (err) {
console.log(err)
} else {
console.log(result)
}
})
運行程序的步驟:要運行應用程序,請從項目的根目錄執行以下命令:
node app.js
輸出:
[ { _id: new ObjectId("63862e73d408a33b4481fd05"), studentId: 1, studentName: 'Aakash', marks: 50, __v: 0 }, { _id: new ObjectId("63862e73d408a33b4481fd05"), studentId: 1, studentName: 'Aakash', marks: 65, __v: 0 }, { _id: new ObjectId("63862e73d408a33b4481fd05"), studentId: 1, studentName: 'Aakash', marks: 75, __v: 0 }, { _id: new ObjectId("63862e73d408a33b4481fd06"), studentId: 2, studentName: 'Bhavesh', marks: 80, __v: 0 }, { _id: new ObjectId("63862e73d408a33b4481fd06"), studentId: 2, studentName: 'Bhavesh', marks: 70, __v: 0 }, { _id: new ObjectId("63862e73d408a33b4481fd06"), studentId: 2, studentName: 'Bhavesh', marks: 90, __v: 0 } ]
示例 2:在此示例中,我們使用 mongoose 建立了一個數據庫連接,並在 statesSchema 上定義了模型,具有三個列或字段 “stateId”、“stateName” 和 “city”。最後,我們使用unwind()city 字段上的方法來解構數組並獲取數組中每個元素的文檔作為輸出。在輸出中,我們可以看到我們正在獲取模式級別城市數組中存在的每個城市的文檔對象。
數據庫結構:數據庫結構如下所示,集合中存在以下文檔。

文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
mongoose.connect("mongodb://localhost:27017/geeksforgeeks", {
useNewUrlParser: true,
useUnifiedTopology: true,
});
const statesSchema = new mongoose.Schema({
stateId: Number,
stateName: String,
city: []
});
const State = mongoose.model('State', statesSchema);
const aggregate = State.aggregate();
aggregate.unwind("$city").then(result => {
console.log(result)
}).catch(err => {
console.log(err)
})
運行程序的步驟:要運行應用程序,請從項目的根目錄執行以下命令:
node app.js
輸出:
[ { _id: new ObjectId("63863213430c4520ce61c186"), stateId: 1, stateName: 'Maharashtra', city: 'Mumbai', __v: 0 }, { _id: new ObjectId("63863213430c4520ce61c186"), stateId: 1, stateName: 'Maharashtra', city: 'Pune', __v: 0 }, { _id: new ObjectId("63863213430c4520ce61c186"), stateId: 1, stateName: 'Maharashtra', city: 'Nagpur', __v: 0 }, { _id: new ObjectId("63863213430c4520ce61c187"), stateId: 2, stateName: 'Madhya Pradesh', city: 'Bhopal', __v: 0 }, { _id: new ObjectId("63863213430c4520ce61c187"), stateId: 2, stateName: 'Madhya Pradesh', city: 'Indore', __v: 0 } ]
參考:https://mongoosejs.com/docs/api/aggregate.html#aggregate_Aggregate-unwind
相關用法
- Mongoose Aggregate prototype.unionWith()用法及代碼示例
- Mongoose Aggregate prototype.sample()用法及代碼示例
- Mongoose Aggregate.prototype.catch()用法及代碼示例
- Mongoose Aggregate.prototype.exec()用法及代碼示例
- Mongoose Aggregate.prototype.model()用法及代碼示例
- Mongoose Aggregate.prototype.skip()用法及代碼示例
- Mongoose Aggregate.prototype.limit()用法及代碼示例
- Mongoose Aggregate.prototype.then()用法及代碼示例
- Mongoose Aggregate.prototype.append()用法及代碼示例
- Mongoose Aggregate.prototype.sortByCount()用法及代碼示例
- Mongoose Aggregate.prototype.project()用法及代碼示例
- Mongoose Aggregate.prototype.pipeline()用法及代碼示例
- Mongoose Aggregate.prototype.match()用法及代碼示例
- Mongoose Aggregate.prototype.cursor()用法及代碼示例
- Mongoose Aggregate.prototype.sort()用法及代碼示例
- Mongoose Aggregate.prototype.addFields()用法及代碼示例
- Mongoose Aggregate.prototype.lookup()用法及代碼示例
- Mongoose countDocuments()用法及代碼示例
- Mongoose deleteMany()用法及代碼示例
- Mongoose deleteOne()用法及代碼示例
- Mongoose estimatedDocumentCount()用法及代碼示例
- Mongoose exists()用法及代碼示例
- Mongoose find()用法及代碼示例
- Mongoose findById()用法及代碼示例
- Mongoose findByIdAndDelete()用法及代碼示例
注:本文由純淨天空篩選整理自kartikmukati大神的英文原創作品 Mongoose Aggregate prototype.unwind() API。非經特殊聲明,原始代碼版權歸原作者所有,本譯文未經允許或授權,請勿轉載或複製。