Mongoose API 的 Aggregate sort() 方法用於執行聚合任務。它允許我們根據我們提供的字段對集合中的所有文檔進行排序。它還為我們提供了按升序或降序對輸入文檔進行排序的靈活性。
用法:
aggregate().sort( { fieldName : sortOrder } )
參數:該方法接受單個參數,如下所述:
- string/object:它用於指定集合中字段的名稱。我們可以提供字符串格式的字段名稱或作為對象以及排序順序。
返回值:該方法以數組的形式返回結果集。
設置 Node.js Mongoose 模塊:
步驟 1:使用以下命令創建 Node.js 應用程序:
npm init
步驟 2:創建 NodeJS 應用程序後,使用以下命令安裝所需的模塊:
npm install mongoose
項目結構: 項目結構將如下所示:

數據庫結構:數據庫結構如下所示,集合中存在以下文檔。
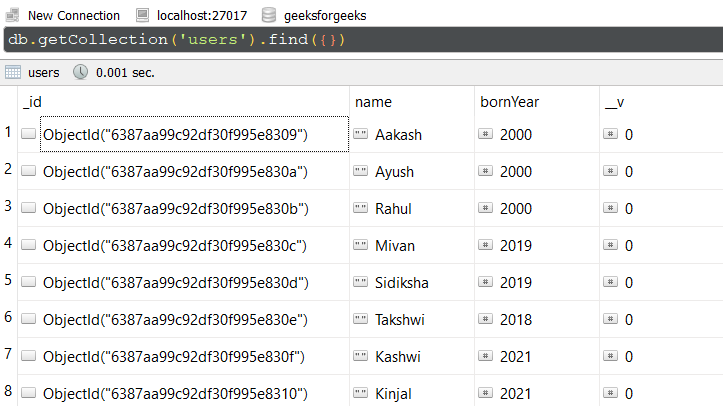
示例 1:在此示例中,我們使用 mongoose 建立了數據庫連接,並通過 userSchema 定義了模型,具有兩個字段 “name” 和 “bornYear”。最後,我們調用sort()方法 對結果集進行排序出生年份按升序排列。要按升序排序,我們必須提供 “1” 作為值出生年份字段並按降序排序值字段值應為“-1”。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
mongoose.connect("mongodb://localhost:27017/geeksforgeeks", {
useNewUrlParser: true,
useUnifiedTopology: true,
});
const userSchema = new mongoose.Schema({
name: String,
bornYear: Number
});
const User = mongoose.model('User', userSchema);
User.aggregate().sort({ 'bornYear': 1 })
.then(result => {
console.log(result)
})
運行程序的步驟:要運行應用程序,請從項目的根目錄執行以下命令:
node app.js
輸出:
[ { _id: new ObjectId("6387aa99c92df30f995e8309"), name: 'Aakash', bornYear: 2000, __v: 0 }, { _id: new ObjectId("6387aa99c92df30f995e830a"), name: 'Ayush', bornYear: 2000, __v: 0 }, { _id: new ObjectId("6387aa99c92df30f995e830b"), name: 'Rahul', bornYear: 2000, __v: 0 }, { _id: new ObjectId("6387aa99c92df30f995e830e"), name: 'Takshwi', bornYear: 2018, __v: 0 }, { _id: new ObjectId("6387aa99c92df30f995e830c"), name: 'Mivan', bornYear: 2019, __v: 0 }, { _id: new ObjectId("6387aa99c92df30f995e830d"), name: 'Sidiksha', bornYear: 2019, __v: 0 }, { _id: new ObjectId("6387aa99c92df30f995e830f"), name: 'Kashwi', bornYear: 2021, __v: 0 }, { _id: new ObjectId("6387aa99c92df30f995e8310"), name: 'Kinjal', bornYear: 2021, __v: 0 } ]
示例 2:在此示例中,我們通過傳遞對象數組參數來使用管道對象。首先我們進行排序出生年份字段按降序排列,然後進行排序名字字段按升序排列。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
mongoose.connect("mongodb://localhost:27017/geeksforgeeks", {
useNewUrlParser: true,
useUnifiedTopology: true,
});
const userSchema = new mongoose.Schema({
name: String,
bornYear: Number
});
const User = mongoose.model('User', userSchema);
User.aggregate([{ $sort: { bornYear: -1, name: 1 } }])
.exec((error, result) => {
if (error) {
console.log(error)
} else {
console.log(result)
}
})
運行程序的步驟:要運行應用程序,請從項目的根目錄執行以下命令:
node app.js
輸出:
[ { _id: new ObjectId("6387aa99c92df30f995e830f"), name: 'Kashwi', bornYear: 2021, __v: 0 }, { _id: new ObjectId("6387aa99c92df30f995e8310"), name: 'Kinjal', bornYear: 2021, __v: 0 }, { _id: new ObjectId("6387aa99c92df30f995e830c"), name: 'Mivan', bornYear: 2019, __v: 0 }, { _id: new ObjectId("6387aa99c92df30f995e830d"), name: 'Sidiksha', bornYear: 2019, __v: 0 }, { _id: new ObjectId("6387aa99c92df30f995e830e"), name: 'Takshwi', bornYear: 2018, __v: 0 }, { _id: new ObjectId("6387aa99c92df30f995e8309"), name: 'Aakash', bornYear: 2000, __v: 0 }, { _id: new ObjectId("6387aa99c92df30f995e830a"), name: 'Ayush', bornYear: 2000, __v: 0 }, { _id: new ObjectId("6387aa99c92df30f995e830b"), name: 'Rahul', bornYear: 2000, __v: 0 } ]
參考:https://mongoosejs.com/docs/api/aggregate.html#aggregate_Aggregate-sort
相關用法
- Mongoose Aggregate.prototype.sortByCount()用法及代碼示例
- Mongoose Aggregate.prototype.skip()用法及代碼示例
- Mongoose Aggregate.prototype.catch()用法及代碼示例
- Mongoose Aggregate.prototype.exec()用法及代碼示例
- Mongoose Aggregate.prototype.model()用法及代碼示例
- Mongoose Aggregate.prototype.limit()用法及代碼示例
- Mongoose Aggregate.prototype.then()用法及代碼示例
- Mongoose Aggregate.prototype.append()用法及代碼示例
- Mongoose Aggregate.prototype.project()用法及代碼示例
- Mongoose Aggregate.prototype.pipeline()用法及代碼示例
- Mongoose Aggregate.prototype.match()用法及代碼示例
- Mongoose Aggregate.prototype.cursor()用法及代碼示例
- Mongoose Aggregate.prototype.addFields()用法及代碼示例
- Mongoose Aggregate.prototype.lookup()用法及代碼示例
- Mongoose Aggregate prototype.unionWith()用法及代碼示例
- Mongoose Aggregate prototype.sample()用法及代碼示例
- Mongoose Aggregate prototype.unwind()用法及代碼示例
- Mongoose countDocuments()用法及代碼示例
- Mongoose deleteMany()用法及代碼示例
- Mongoose deleteOne()用法及代碼示例
- Mongoose estimatedDocumentCount()用法及代碼示例
- Mongoose exists()用法及代碼示例
- Mongoose find()用法及代碼示例
- Mongoose findById()用法及代碼示例
- Mongoose findByIdAndDelete()用法及代碼示例
注:本文由純淨天空篩選整理自sakshio0hoj大神的英文原創作品 Mongoose Aggregate.prototype.sort() API。非經特殊聲明,原始代碼版權歸原作者所有,本譯文未經允許或授權,請勿轉載或複製。