Java中的List接口提供了一種存儲有序集合的方法。它是一個子接口集合。它是一個有序的對象集合,其中可以存儲重複的值。由於 List 保留插入順序,因此它允許位置訪問和插入元素。
表中的內容
Java中的列表接口
List接口位於java.util包中,並繼承了Collection接口。它是ListIterator接口的工廠。通過ListIterator,我們可以向前和向後迭代列表。 List接口的實現類有ArrayList、LinkedList、Stack和Vector。 ArrayList 和 LinkedList 在 Java 編程中廣泛使用。 Vector 類自 Java 5 起已被棄用。
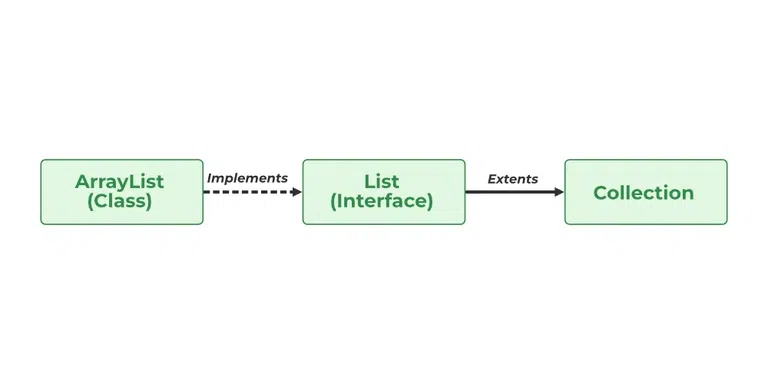
Java 集合框架中的列表和ArrayList
Java列表接口聲明
public interface List<E> extends Collection<E> ;
讓我們詳細說明在 List 類中創建對象或實例。自從List是一個接口,不能創建列表類型的對象。我們總是需要一個類來實現這個List為了創建一個對象。而且,推出後泛型在Java 1.5中,可以限製List中可以存儲的對象類型。就像用戶自定義‘classes’實現的其他幾個用戶自定義‘interfaces’一樣,List是‘interface’,由ArrayList類,預定義在java.util包。
Java列表的語法
這種類型的安全列表可以定義為:
List<Obj> list = new ArrayList<Obj> ();
Note: Obj is the type of the object to be stored in List
Java 列表示例
Java
// Java program to Demonstrate List Interface
// Importing all utility classes
import java.util.*;
// Main class
// ListDemo class
class GFG {
// Main driver method
public static void main(String[] args)
{
// Creating an object of List interface
// implemented by the ArrayList class
List<Integer> l1 = new ArrayList<Integer>();
// Adding elements to object of List interface
// Custom inputs
l1.add(0, 1);
l1.add(1, 2);
// Print the elements inside the object
System.out.println(l1);
// Now creating another object of the List
// interface implemented ArrayList class
// Declaring object of integer type
List<Integer> l2 = new ArrayList<Integer>();
// Again adding elements to object of List interface
// Custom inputs
l2.add(1);
l2.add(2);
l2.add(3);
// Will add list l2 from 1 index
l1.addAll(1, l2);
System.out.println(l1);
// Removes element from index 1
l1.remove(1);
// Printing the updated List 1
System.out.println(l1);
// Prints element at index 3 in list 1
// using get() method
System.out.println(l1.get(3));
// Replace 0th element with 5
// in List 1
l1.set(0, 5);
// Again printing the updated List 1
System.out.println(l1);
}
}
[1, 2] [1, 1, 2, 3, 2] [1, 2, 3, 2] 2 [5, 2, 3, 2]
現在讓我們使用列表接口執行各種操作,以更好地理解它。我們將討論下麵列出的以下操作,稍後將通過幹淨的 Java 代碼實現它們。
Java 列表接口中的操作
由於 List 是一個接口,因此它隻能與實現該接口的類一起使用。現在,我們來看看如何對 List 進行一些常用的操作。
- 操作1:使用 add() 方法向 List 類添加元素
- 操作2:使用set()方法更新List類中的元素
- 操作3:使用 indexOf()、lastIndexOf 方法搜索元素
- 操作4:使用remove()方法刪除元素
- 操作5:使用get()方法訪問List類中的元素
- 操作6:使用 contains() 方法檢查 List 類中是否存在元素
現在讓我們單獨討論這些操作,並在代碼中實現相同的操作,以便更好地掌握它。
1.使用以下方法將元素添加到 List 類add()方法
為了將元素添加到列表中,我們可以使用 List add()List get()方法。該方法被重載以根據不同的參數執行多個操作。
參數:它需要2個參數,即:
- add(Object):該方法用於在List的末尾添加一個元素。
- add(int index, Object):該方法用於在List中的特定索引處添加元素
例子:
Java
// Java Program to Add Elements to a List
// Importing all utility classes
import java.util.*;
// Main class
class GFG {
// Main driver method
public static void main(String args[])
{
// Creating an object of List interface,
// implemented by ArrayList class
List<String> al = new ArrayList<>();
// Adding elements to object of List interface
// Custom elements
al.add("Geeks");
al.add("Geeks");
al.add(1, "For");
// Print all the elements inside the
// List interface object
System.out.println(al);
}
}
[Geeks, For, Geeks]
2. 更新元素
添加元素後,如果我們想更改元素,可以使用 AbstractList set() 方法。由於 List 是有索引的,因此我們希望更改的元素是通過元素的索引來引用的。因此,該方法需要一個索引和需要在該索引處插入的更新元素。
例子:
Java
// Java Program to Update Elements in a List
// Importing utility classes
import java.util.*;
// Main class
class GFG {
// Main driver method
public static void main(String args[])
{
// Creating an object of List interface
List<String> al = new ArrayList<>();
// Adding elements to object of List class
al.add("Geeks");
al.add("Geeks");
al.add(1, "Geeks");
// Display theinitial elements in List
System.out.println("Initial ArrayList " + al);
// Setting (updating) element at 1st index
// using set() method
al.set(1, "For");
// Print and display the updated List
System.out.println("Updated ArrayList " + al);
}
}
Initial ArrayList [Geeks, Geeks, Geeks] Updated ArrayList [Geeks, For, Geeks]
3.搜索元素
在List接口中搜索元素是Java編程中的常見操作。 List 接口提供了多種搜索元素的方法,例如List indexOf(), List lastIndexOf()方法。
indexOf() 方法返回列表中指定元素第一次出現的索引,而 lastIndexOf() 方法返回指定元素最後一次出現的索引。
參數:
- indexOf(element): 返回列表中指定元素第一次出現的索引,如果未找到該元素則返回 -1
- lastIndexOf(element): 返回列表中指定元素最後一次出現的索引,如果未找到該元素則返回 -1
例子:
Java
import java.util.ArrayList;
import java.util.List;
public class ListExample {
public static void main(String[] args)
{
// create a list of integers
List<Integer> numbers = new ArrayList<>();
// add some integers to the list
numbers.add(1);
numbers.add(2);
numbers.add(3);
numbers.add(2);
// use indexOf() to find the first occurrence of an
// element in the list
int index = numbers.indexOf(2);
System.out.println(
"The first occurrence of 2 is at index "
+ index);
// use lastIndexOf() to find the last occurrence of
// an element in the list
int lastIndex = numbers.lastIndexOf(2);
System.out.println(
"The last occurrence of 2 is at index "
+ lastIndex);
}
}
The first occurrence of 2 is at index 1 The last occurrence of 2 is at index 3
4. 刪除元素
為了從列表中刪除一個元素,我們可以使用 List remove(Object obj)方法。該方法被重載以根據不同的參數執行多個操作。他們是:
參數:
- remove(Object):此方法用於簡單地從列表中刪除對象。如果存在多個此類對象,則刪除第一個出現的對象。
- remove(int index):由於列表已建立索引,因此此方法采用一個整數值,該整數值僅刪除列表中特定索引處存在的元素。刪除元素後,所有元素都會向左移動以填充空間,並更新對象的索引。
例子:
Java
// Java Program to Remove Elements from a List
// Importing List and ArrayList classes
// from java.util package
import java.util.ArrayList;
import java.util.List;
// Main class
class GFG {
// Main driver method
public static void main(String args[])
{
// Creating List class object
List<String> al = new ArrayList<>();
// Adding elements to the object
// Custom inputs
al.add("Geeks");
al.add("Geeks");
// Adding For at 1st indexes
al.add(1, "For");
// Print the initialArrayList
System.out.println("Initial ArrayList " + al);
// Now remove element from the above list
// present at 1st index
al.remove(1);
// Print the List after removal of element
System.out.println("After the Index Removal " + al);
// Now remove the current object from the updated
// List
al.remove("Geeks");
// Finally print the updated List now
System.out.println("After the Object Removal "
+ al);
}
}
Initial ArrayList [Geeks, For, Geeks] After the Index Removal [Geeks, Geeks] After the Object Removal [Geeks]
5. 訪問元素
為了訪問列表中的元素,我們可以使用List get()方法,返回指定索引處的元素
參數:
get(int index):此方法返回列表中指定索引處的元素。
例子:
Java
// Java Program to Access Elements of a List
// Importing all utility classes
import java.util.*;
// Main class
class GFG {
// Main driver method
public static void main(String args[])
{
// Creating an object of List interface,
// implemented by ArrayList class
List<String> al = new ArrayList<>();
// Adding elements to object of List interface
al.add("Geeks");
al.add("For");
al.add("Geeks");
// Accessing elements using get() method
String first = al.get(0);
String second = al.get(1);
String third = al.get(2);
// Printing all the elements inside the
// List interface object
System.out.println(first);
System.out.println(second);
System.out.println(third);
System.out.println(al);
}
}
Geeks For Geeks [Geeks, For, Geeks]
6. 檢查列表中是否存在元素
為了檢查列表中是否存在某個元素,我們可以使用List contains() 方法。如果指定的元素存在於列表中,則此方法返回 true,否則返回 false。
參數:
contains(Object):此方法采用單個參數,即要檢查的對象是否存在於列表中。
例子:
Java
// Java Program to Check if an Element is Present in a List
// Importing all utility classes
import java.util.*;
// Main class
class GFG {
// Main driver method
public static void main(String args[])
{
// Creating an object of List interface,
// implemented by ArrayList class
List<String> al = new ArrayList<>();
// Adding elements to object of List interface
al.add("Geeks");
al.add("For");
al.add("Geeks");
// Checking if element is present using contains()
// method
boolean isPresent = al.contains("Geeks");
// Printing the result
System.out.println("Is Geeks present in the list? "
+ isPresent);
}
}
Is Geeks present in the list? true
Java中List接口的複雜性
操作 |
時間複雜度 |
空間複雜度 |
---|---|---|
列表接口添加元素 |
O(1) |
O(1) |
從列表接口中刪除元素 |
O(N) |
O(N) |
替換列表接口中的元素 |
O(N) |
O(N) |
遍曆列表接口 |
O(N) |
O(N) |
Java 中列表接口的迭代
到目前為止,我們的輸入大小非常小,並且我們正在為每個實體手動執行操作。現在讓我們討論迭代列表以使它們適用於更大的樣本集的各種方法。
方法:有多種方法可以迭代列表。最著名的方法是使用基本的for循環結合一個List get()獲取特定索引處的元素循環高級.
例子:
Java
// Java program to Iterate the Elements
// in an ArrayList
// Importing java utility classes
import java.util.*;
// Main class
public class GFG {
// main driver method
public static void main(String args[])
{
// Creating an empty Arraylist of string type
List<String> al = new ArrayList<>();
// Adding elements to above object of ArrayList
al.add("Geeks");
al.add("Geeks");
// Adding element at specified position
// inside list object
al.add(1, "For");
// Using for loop for iteration
for (int i = 0; i < al.size(); i++) {
// Using get() method to
// access particular element
System.out.print(al.get(i) + " ");
}
// New line for better readability
System.out.println();
// Using for-each loop for iteration
for (String str : al)
// Printing all the elements
// which was inside object
System.out.print(str + " ");
}
}
Geeks For Geeks Geeks For Geeks
列表接口的方法
由於不同類型列表背後的主要概念是相同的,因此列表接口包含以下方法:
方法 |
說明 |
---|---|
List add(int index, E element) | 此方法與 Java 列表接口一起使用,以在列表中的特定索引處添加元素。當傳遞單個參數時,它隻是將元素添加到列表末尾。 |
List addAll() | 此方法與 Java 中的列表接口一起使用,將給定集合中的所有元素添加到列表中。當傳遞單個參數時,它會將給定集合的所有元素添加到列表末尾。 |
List size() | 此方法與 Java 列表接口一起使用以返回列表的大小。 |
List clear() | 該方法用於刪除列表中的所有元素。但是,所創建的列表的引用仍然被存儲。 |
List remove(int index) | 此方法從指定索引中刪除一個元素。它將後續元素(如果有)向左移動,並將其索引減 1。 |
List remove(Object obj) | 此方法與 Java 列表接口一起使用,以刪除列表中第一次出現的給定元素。 |
List get() | 此方法返回指定索引處的元素。 |
ArrayList set() | 此方法用新元素替換給定索引處的元素。該函數返回剛剛被新元素替換的元素。 |
List indexOf() | 此方法返回給定元素的第一次出現或-1如果該元素不存在於列表中。 |
List lastIndexOf() | 此方法返回給定元素的最後一次出現或-1如果該元素不存在於列表中。 |
List equals() | 此方法與 Java 列表接口一起使用,以比較給定元素與列表元素的相等性。 |
List hashCode() | 該方法與 Java 中的 List Interface 一起使用,返回給定列表的 hashcode 值。 |
List isEmpty() | 此方法與 Java 列表接口一起使用來檢查列表是否為空。如果列表為空,則返回 true,否則返回 false。 |
List contains() | 此方法與 Java 中的列表接口一起使用,以檢查列表是否包含給定元素。如果列表包含該元素,則返回 true。 |
List containsAll() | 此方法與 Java 列表接口一起使用來檢查列表是否包含所有元素的集合。 |
sort(Comparator comp) | 該方法與 Java 中的 List Interface 一起使用,根據給定的值對列表的元素進行排序Comparator Interface. |
Java 列表與集合
List接口和Set接口都繼承了Collection接口。然而,它們之間存在一些差異。
List |
放 |
---|---|
List 是一個有序序列。 | Set 是一個無序序列。 |
列表允許重複元素 | Set 不允許有重複的元素。 |
可以按元素的位置來訪問元素。 | 不允許對元素進行位置訪問。 |
可以存儲多個空元素。 | null 元素隻能存儲一次。 |
列表的實現有ArrayList、LinkedList、Vector、Stack | Set的實現有HashSet、LinkedHashSet。 |
類與 Java 列表接口的關聯
現在讓我們討論實現 List 接口的類,首先請參考下麵的圖示以更好地理解 List 接口。如下:
AbstractList,CopyOnWriteArrayList, 和AbstractSequentialList是實現 List 接口的類。在每個提到的類中都實現了單獨的函數。它們如下:
- 摘要列表:該類用於實現一個不可修改的列表,隻需擴展AbstractList類並僅實現get()和size()方法。
- 寫入數組列表時複製:該類實現了列表接口。它是一個增強版本ArrayList其中所有修改(添加、設置、刪除等)都是通過製作列表的新副本來實現的。
- 抽象順序列表:這個類實現了采集接口和AbstractCollection 類。該類用於實現一個不可修改的列表,隻需擴展AbstractList類並僅實現get()和size()方法。
我們將按照這種方式進行。
- ArrayList
- Vector
- Stack
- LinkedList
讓我們按順序討論它們並實現相同的方法,以了解使用 List 接口的類的工作原理。
1.ArrayList
ArrayList集合框架中實現的類為我們提供了Java中的動態數組。雖然它可能比標準數組慢,但對於需要對數組進行大量操作的程序很有幫助。讓我們看看如何使用此類創建列表對象。
例子:
Java
// Java program to demonstrate the
// creation of list object using the
// ArrayList class
import java.io.*;
import java.util.*;
class GFG {
public static void main(String[] args)
{
// Size of ArrayList
int n = 5;
// Declaring the List with initial size n
List<Integer> arrli = new ArrayList<Integer>(n);
// Appending the new elements
// at the end of the list
for (int i = 1; i <= n; i++)
arrli.add(i);
// Printing elements
System.out.println(arrli);
// Remove element at index 3
arrli.remove(3);
// Displaying the list after deletion
System.out.println(arrli);
// Printing elements one by one
for (int i = 0; i < arrli.size(); i++)
System.out.print(arrli.get(i) + " ");
}
}
[1, 2, 3, 4, 5] [1, 2, 3, 5] 1 2 3 5
2.矢量
Vector是在集合框架中實現的類,實現了可增長的對象數組。 Vector 實現了動態數組,這意味著它可以根據需要增長或縮小。與數組一樣,它包含可以使用整數索引訪問的組件。向量本質上屬於遺留類,但現在它與集合完全兼容。讓我們看看如何使用此類創建列表對象。
例子:
Java
// Java program to demonstrate the
// creation of list object using the
// Vector class
import java.io.*;
import java.util.*;
class GFG {
public static void main(String[] args)
{
// Size of the vector
int n = 5;
// Declaring the List with initial size n
List<Integer> v = new Vector<Integer>(n);
// Appending the new elements
// at the end of the list
for (int i = 1; i <= n; i++)
v.add(i);
// Printing elements
System.out.println(v);
// Remove element at index 3
v.remove(3);
// Displaying the list after deletion
System.out.println(v);
// Printing elements one by one
for (int i = 0; i < v.size(); i++)
System.out.print(v.get(i) + " ");
}
}
[1, 2, 3, 4, 5] [1, 2, 3, 5] 1 2 3 5
3. 堆棧
Stack是一個在集合框架中實現的類,它擴展了向量類模型並實現了棧數據結構。該類基於後進先出的基本原則。除了基本的入棧和出棧操作外,該類還提供了清空、搜索和查看三個函數。讓我們看看如何使用此類創建列表對象。
例子:
Java
// Java program to demonstrate the
// creation of list object using the
// Stack class
import java.io.*;
import java.util.*;
class GFG {
public static void main(String[] args)
{
// Size of the stack
int n = 5;
// Declaring the List
List<Integer> s = new Stack<Integer>();
// Appending the new elements
// at the end of the list
for (int i = 1; i <= n; i++)
s.add(i);
// Printing elements
System.out.println(s);
// Remove element at index 3
s.remove(3);
// Displaying the list after deletion
System.out.println(s);
// Printing elements one by one
for (int i = 0; i < s.size(); i++)
System.out.print(s.get(i) + " ");
}
}
[1, 2, 3, 4, 5] [1, 2, 3, 5] 1 2 3 5
4.LinkedList
LinkedList 是一個在集合框架中實現的類,它本質上實現了鏈表數據結構。它是一種線性數據結構,其中元素不存儲在連續位置,並且每個元素都是具有數據部分和地址部分的單獨對象。這些元素使用指針和地址鏈接。每個元素稱為一個節點。由於插入和刪除的動態性和簡便性,它們比數組更受青睞。讓我們看看如何使用此類創建列表對象。
例子:
Java
// Java program to demonstrate the
// creation of list object using the
// LinkedList class
import java.io.*;
import java.util.*;
class GFG {
public static void main(String[] args)
{
// Size of the LinkedList
int n = 5;
// Declaring the List with initial size n
List<Integer> ll = new LinkedList<Integer>();
// Appending the new elements
// at the end of the list
for (int i = 1; i <= n; i++)
ll.add(i);
// Printing elements
System.out.println(ll);
// Remove element at index 3
ll.remove(3);
// Displaying the list after deletion
System.out.println(ll);
// Printing elements one by one
for (int i = 0; i < ll.size(); i++)
System.out.print(ll.get(i) + " ");
}
}
[1, 2, 3, 4, 5] [1, 2, 3, 5] 1 2 3 5
相關用法
- Java List sort()用法及代碼示例
- Java List spliterator()用法及代碼示例
- Java List toArray()用法及代碼示例
- Java List add()用法及代碼示例
- Java List addAll()用法及代碼示例
- Java List clear()用法及代碼示例
- Java List contains()用法及代碼示例
- Java List containsAll()用法及代碼示例
- Java List equals()用法及代碼示例
- Java List get()用法及代碼示例
- Java List hashCode()用法及代碼示例
- Java List indexOf()用法及代碼示例
- Java List isEmpty()用法及代碼示例
- Java List lastIndexOf()用法及代碼示例
- Java List listIterator()用法及代碼示例
- Java List removeAll()用法及代碼示例
- Java List retainAll()用法及代碼示例
- Java List size()用法及代碼示例
- Java List sublist()用法及代碼示例
- Java List remove(Object obj)用法及代碼示例
- Java List add(E ele)用法及代碼示例
- Java List remove(int index)用法及代碼示例
- Java List add(int index, E element)用法及代碼示例
- Java ListIterator add()用法及代碼示例
- Java ListIterator hasNext()用法及代碼示例
注:本文由純淨天空篩選整理自佚名大神的英文原創作品 List Interface in Java with Examples。非經特殊聲明,原始代碼版權歸原作者所有,本譯文未經允許或授權,請勿轉載或複製。