length 屬性以 32 位無符號整數的形式返回數組中的元素數。我們也可以說 length 屬性返回一個表示數組元素數量的數字。返回值總是大於最高數組索引。
length 屬性還可用於設置數組中元素的數量。我們必須結合使用賦值運算符和 length 屬性來設置數組的長度。
JavaScript 中的 array.length 屬性與 jQuery 中的 array.size() 方法相同。在 JavaScript 中,使用 array.size() 方法是無效的,所以我們使用 array.length 屬性來計算數組的大小。
用法
以下語法用於返回數組的長度
array.length
以下語法用於設置數組的長度
array.length = number
為了更好地理解,讓我們看一些使用 array.length 屬性的插圖。
示例 1
這是一個了解如何使用 array.length 屬性計算數組長度的簡單示例。
<html>
<head>
<title> array.length </title>
</head>
<body>
<h3> Here, we are finding the length of an array. </h3>
<script>
var arr = new Array( 100, 200, 300, 400, 500, 600 );
document.write(" The elements of array are:" + arr);
document.write(" <br>The length of the array is:" + arr.length);
</script>
</body>
</html>
輸出
在輸出中,我們可以看到數組的長度為 6,大於數組最高索引的值。上例中指定數組的最高索引為 5。
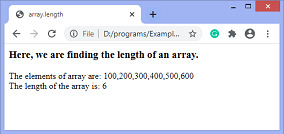
例2
在本例中,我們使用 array.length 屬性設置數組的長度。最初,數組包含兩個元素,所以一開始,長度是 2。然後我們將數組的長度增加到 9。
在輸出中,數組的值用逗號分隔。增加長度後,數組包含兩個已定義值和七個未定義值,以逗號分隔。然後我們插入五個數組元素並打印它們。現在,該數組包含七個已定義值和兩個未定義值。
<html>
<head>
<title> array.length </title>
</head>
<body>
<h3> Here, we are setting the length of an array. </h3>
<script>
var arr = [100, 200];
document.write(" Before setting the length, the array elements are:" + arr);
arr.length = 9;
document.write("<br><br> After setting the length, the array elements are:" + arr);
// It will print [ 1, 2, <7 undefined items> ]
arr[2] = 300;
arr[3] = 400;
arr[4] = 500;
arr[5] = 600;
document.write("<br><br> After inserting some array elements:" + arr);
</script>
</body>
</html>
輸出
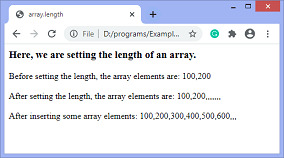
在下一個示例中,我們將測試具有非數字索引的數組的 length 屬性。
例3
在這個例子中,數組的索引是非數字的。這裏,數組包含五個具有非數字索引的元素。我們正在給定數組上應用 length 屬性以查看效果。現在讓我們看看 array.length 屬性如何處理數組的非數字索引。
<html>
<head>
<title> array.length </title>
</head>
<body>
<h3> There are five array elements but the index of the array is non numeric. </h3>
<script>
var arr = new Array();
arr['a'] = 100;
arr['b'] = 200;
arr['c'] = 300;
arr['d'] = 400;
arr['e'] = 500;
document.write("The length of array is:" + arr.length);
</script>
</body>
</html>
輸出
在輸出中,我們可以看到數組的長度顯示為0。執行上麵的代碼後輸出將是——
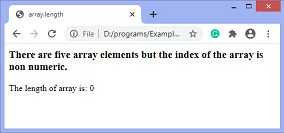
我們還可以使用 length 屬性來找出字符串中的單詞數。讓我們通過一個例子來理解它。
例4
在此示例中,我們使用 length 屬性來顯示字符串中存在的單詞數。在這裏,我們正在創建一個數組,並為數組元素使用 split() 函數。我們將字符串與空白 (" ") 字符分開。
如果我們直接在字符串上應用 length 屬性,那麽它會給我們字符串中的字符數。但在這個例子中,我們將了解如何計算字符串中的單詞數。
<html>
<head>
<title> array.length </title>
</head>
<body>
<script>
var str = "Welcome to the javaTpoint.com";
var arr = new Array();
arr = str.split(" ");
document.write(" The given string is:" + str);
document.write("<br><br> Number Of Words:"+ arr.length);
document.write("<br><br> Number of characters in the string:" + str.length);
</script>
</body>
</html>
輸出
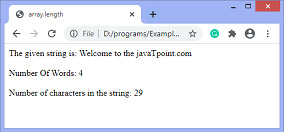
相關用法
- JavaScript Uint8Array.of()用法及代碼示例
- JavaScript BigInt.prototype.toString()用法及代碼示例
- JavaScript DataView.getInt16()用法及代碼示例
- JavaScript Symbol.keyFor()用法及代碼示例
- JavaScript handler.has()用法及代碼示例
- JavaScript JSON.stringify()用法及代碼示例
- JavaScript Symbol.split屬性用法及代碼示例
- JavaScript Function.displayName屬性用法及代碼示例
- JavaScript TypedArray reverse()用法及代碼示例
- JavaScript String slice()用法及代碼示例
- JavaScript 正則 \n用法及代碼示例
- JavaScript Math hypot()用法及代碼示例
- JavaScript Set add()用法及代碼示例
- JavaScript Array fill()用法及代碼示例
- JavaScript Math abs()用法及代碼示例
- JavaScript Date toISOString()用法及代碼示例
- JavaScript DataView.getInt8()用法及代碼示例
- JavaScript dataView.setBigInt64()用法及代碼示例
- JavaScript handler.get()用法及代碼示例
- JavaScript Math.tanh()用法及代碼示例
注:本文由純淨天空篩選整理自 JavaScript array.length property。非經特殊聲明,原始代碼版權歸原作者所有,本譯文未經允許或授權,請勿轉載或複製。