Cstrcat()函數將src指向的字符串追加到dest指向的字符串末尾。它將在目標字符串中附加源字符串的副本。加上一個終止空字符。字符串(src)的初始字符覆蓋字符串(dest)末尾的空字符。
它是c中字符串庫<string.h>和C++中<cstring>下預定義的字符串處理函數。
用法:
char *strcat(char *dest, const char *src);
Parameters: 該方法接受以下參數:
- dest: 這是指向目標數組的指針,該數組應包含 C 字符串,並且應足夠大以包含連接的結果字符串。
- src: 這是要附加的字符串。這不應與目的地重疊。
返回值:
- strcat() 函數返回目的地,指向目標字符串的指針。
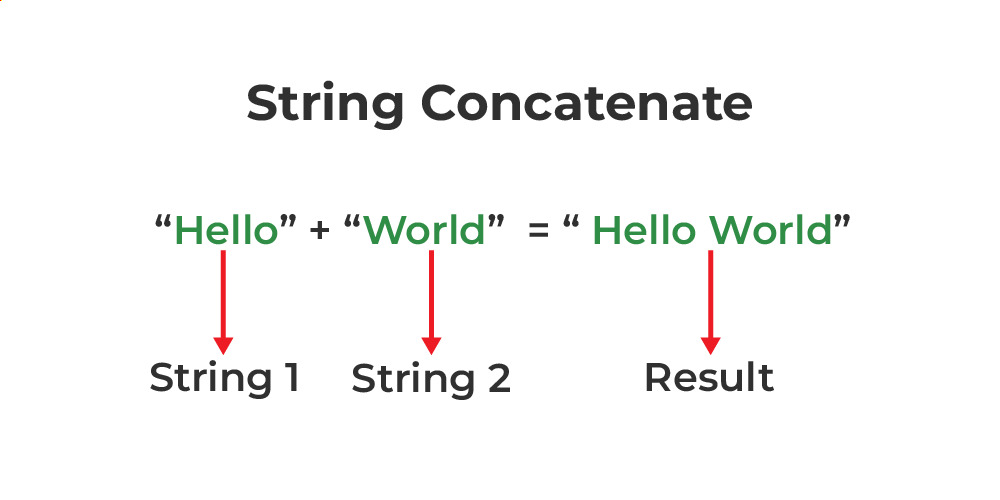
例子:
Input: src = "ForGeeks" dest = "Geeks" Output: "GeeksForGeeks" Input: src = "World" dest = "Hello " Output: "Hello World"
下麵是實現上述方法的 C/C++ 程序:
C
// C program to implement
// the above approach
#include <stdio.h>
#include <string.h>
// Driver code
int main()
{
// Define a temporary variable
char example[100];
// Copy the first string into
// the variable
strcpy(example, "Geeks");
// Concatenate this string
// to the end of the first one
strcat(example, "ForGeeks");
// Display the concatenated strings
printf("%s\n", example);
return 0;
}
輸出
GeeksForGeeks
如果出現以下情況,strcat() 的行為未定義:
- 目標數組對於 src 和 dest 的內容以及終止空字符來說不夠大
- 如果字符串重疊。
- 如果 dest 或 src 不是指向以 null 結尾的字節字符串的指針。
相關用法
- C語言 strcat()用法及代碼示例
- C語言 strchr()用法及代碼示例
- C語言 strcpy()用法及代碼示例
- C語言 strcmp()用法及代碼示例
- C語言 strcoll()用法及代碼示例
- C語言 strcmpi()用法及代碼示例
- C語言 strcspn()用法及代碼示例
- C語言 strtod()用法及代碼示例
- C語言 strtol()用法及代碼示例
- C語言 strtoul()用法及代碼示例
- C語言 strncat()用法及代碼示例
- C語言 strncmp()用法及代碼示例
- C語言 strncpy()用法及代碼示例
- C語言 strerror()用法及代碼示例
- C語言 strrchr()用法及代碼示例
- C語言 strstr()用法及代碼示例
- C語言 strtok()用法及代碼示例
- C語言 strxfrm()用法及代碼示例
- C語言 strftime()用法及代碼示例
- C語言 strlwr()用法及代碼示例
- C語言 strrev()用法及代碼示例
- C語言 strlen()用法及代碼示例
- C語言 strupr()用法及代碼示例
- C語言 strnset()用法及代碼示例
- C語言 strpbrk()用法及代碼示例
注:本文由純淨天空篩選整理自dhanshreekulkarni21大神的英文原創作品 strcat() in C。非經特殊聲明,原始代碼版權歸原作者所有,本譯文未經允許或授權,請勿轉載或複製。