Mongoose 模式API.原型.static() 方法Mongoose API 的函數用於 Schema 對象。它允許我們使用模式對象為模型定義靜態類方法。我們可以訪問靜止的模式對象上的方法來定義靜態方法。讓我們了解一下static() 方法使用一個例子。
用法:
schemaObject.static( method, callback );
參數:該方法接受兩個參數,如下所述:
- method:它用於在模型的類級別指定方法名稱。
- callback:它用於指定將為該方法執行特定任務的函數。
返回值:該方法可以根據我們的函數定義返回任何值。
設置 Node.js Mongoose 模塊:
步驟 1:使用以下命令創建 Node.js 應用程序:
npm init
步驟 2:創建 NodeJS 應用程序後,使用以下命令安裝所需的模塊:
npm install mongoose
項目結構: 項目結構將如下所示:
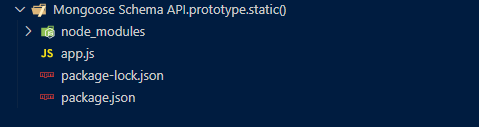
示例 1:下麵的示例說明了 Mongoose Schema 的函數static()方法。在此示例中,我們定義了名為的靜態方法按地址查找客戶,我們正在獲取其值為地址字段值為印多爾, 和我們使用 then 和 catch 塊將返回值作為承諾處理。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
const URI = "mongodb://localhost:27017/geeksforgeeks"
const connectionObject = mongoose.createConnection(URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
const customerSchema = new mongoose.Schema({
name: String,
address: String,
orderNumber: Number,
});
customerSchema.static('findCustomerByAddress',
function (address) {
return this.find({ address: address });
})
const Customer =
connectionObject.model('Customer', customerSchema);
Customer.findCustomerByAddress('Indore').then(result => {
console.log(result);
}).catch(error => console.log(error));
運行程序的步驟:要運行應用程序,請從項目的根目錄執行以下命令:
node app.js
輸出:
[ { _id: new ObjectId("639ede899fdf57759087a655"), name: 'Chintu', address: 'Indore', orderNumber: 6, __v: 0 } ]
示例 2:下麵的示例說明了 Mongoose Schema 的函數static()方法。在此示例中,我們定義了名為的靜態方法得到所有,我們從數據庫中獲取所有文檔,並使用匿名異步函數處理返回值。
文件名:app.js
Javascript
// Require mongoose module
const mongoose = require("mongoose");
// Set Up the Database connection
const URI = "mongodb://localhost:27017/geeksforgeeks"
const connectionObject = mongoose.createConnection(URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
const customerSchema = new mongoose.Schema({
name: String,
address: String,
orderNumber: Number,
});
customerSchema.static('getAll', function () {
return this.find({});
})
const Customer =
connectionObject.model('Customer', customerSchema);
(async () => {
const result = await Customer.getAll();
console.log(result);
})();
運行程序的步驟:要運行應用程序,請從項目的根目錄執行以下命令:
node app.js
輸出:
[ { _id: new ObjectId("639ede899fdf57759087a655"), name: 'Chintu', address: 'Indore', orderNumber: 6, __v: 0 }, { _id: new ObjectId("639ede899fdf57759087a653"), name: 'Aditya', address: 'Mumbai', orderNumber: 2, __v: 0 }, { _id: new ObjectId("639ede899fdf57759087a654"), name: 'Bhavesh', address: 'Delhi', orderNumber: 5, __v: 0 } ]
參考:https://mongoosejs.com/docs/api/schema.html#schema_Schema-static
相關用法
- Mongoose Schema.prototype.virtual()用法及代碼示例
- Mongoose Schema.prototype.plugin()用法及代碼示例
- Mongoose Schema.prototype.pre()用法及代碼示例
- Mongoose SchemaType.prototype.ref()用法及代碼示例
- Mongoose SchemaType.prototype.default()用法及代碼示例
- Mongoose SchemaType.prototype.immutable()用法及代碼示例
- Mongoose SchemaType.prototype.unique()用法及代碼示例
- Mongoose Schema Connection.prototype.asPromise()用法及代碼示例
- Mongoose SchemaType.prototype.validate()用法及代碼示例
- Mongoose Schema Connection.prototype.dropCollection()用法及代碼示例
- Mongoose SchemaType.prototype.get()用法及代碼示例
- Mongoose Schema Connection.prototype.set()用法及代碼示例
- Mongoose SchemaType.prototype.text()用法及代碼示例
- Mongoose SchemaType.prototype.set()用法及代碼示例
- Mongoose SchemaType.prototype.required()用法及代碼示例
- Mongoose SchemaType.prototype.select()用法及代碼示例
- Mongoose Schema Connection.prototype.dropDatabase()用法及代碼示例
- Mongoose SchemaType.prototype.transform()用法及代碼示例
- Mongoose SchemaType.prototype.index()用法及代碼示例
- Mongoose Schema Connection.prototype.close()用法及代碼示例
- Mongoose Schema Connection.prototype.useDb()用法及代碼示例
- Mongoose countDocuments()用法及代碼示例
- Mongoose deleteMany()用法及代碼示例
- Mongoose deleteOne()用法及代碼示例
- Mongoose estimatedDocumentCount()用法及代碼示例
注:本文由純淨天空篩選整理自sakshio0hoj大神的英文原創作品 Mongoose Schema.prototype.static() API。非經特殊聲明,原始代碼版權歸原作者所有,本譯文未經允許或授權,請勿轉載或複製。