RescaleOp是java.awt.image包中的一個類,它實現了BufferedImageOp和RasterOp接口。該類通過將每個像素的樣本值乘以比例因子然後添加偏移量來對源圖像中的數據執行 pixel-by-pixel 重新縮放。縮放後的樣本值被裁剪為目標圖像中的最小/最大表示。該類用於圖片處理。
- 為了光柵,重新縮放在頻帶上進行。縮放常數組的數量可以是一組,在這種情況下,相同的常數應用於所有波段,或者它必須等於源柵格波段的數量。
- 為了BufferedImages,重新縮放對顏色進行操作。縮放常數組的數量可以是一組,在這種情況下,相同的常數被應用於所有顏色分量。
- 圖像帶有IndexColorModel無法重新縮放。
- 如果一個RenderingHints在構造函數中指定對象,當需要進行顏色轉換時,可以使用顏色渲染提示和抖動提示。
用法:
public class RescaleOp extends Object implements BufferedImageOp, RasterOp
構造函數:
- public RescaleOp(float[]scaleFactors,float[]offsets,RenderingHintshints):具有所需比例因子和偏移量的構造函數。提示可以為空。
- 公共RescaleOp(浮點比例因子,浮點偏移量,RenderingHints提示):具有單個比例因子和偏移量的構造函數。提示可以為空。
方法:
- BufferedImage createCompatibleDestImage?(BufferedImage src,ColorModel destCM):此方法創建具有正確大小和波段數量的歸零目標圖像。
- WritableRaster createCompatibleDestRaster?(柵格源):給定此源,此方法將創建具有正確大小和波段數的 zeroed-destination 柵格。
- BufferedImage 過濾器?(BufferedImage src,BufferedImage dst):此方法重新縮放源 BufferedImage。
- WritableRaster 過濾器?(光柵源,WritableRaster dst):此方法重新縮放源柵格中的像素數據。
- Rectangle2D getBounds2D?(BufferedImage src):此方法返回重新縮放的目標圖像的邊界框。
- Rectangle2D getBounds2D?(光柵源):此方法返回重新縮放的目標柵格的邊界框。
- int getNumFactors?():此方法返回此 RescaleOp 中使用的縮放因子和偏移量的數量。
- float[] getOffsets?(float[] 偏移量):此方法返回給定數組中的偏移量。
- Point2D getPoint2D?(Point2D srcPt,Point2D dstPt):此方法返回給定源中的點的目標點的位置。
- RenderingHints getRenderingHints?():此方法返回此操作的渲染提示。
- float[] getScaleFactors?(float[] scaleFactors):此方法返回給定數組中的比例因子。
示例 1:在給定的示例中,我們將使用單個比例因子和偏移量設置圖片的對比度。以下代碼會將亮度降低 25%,並使像素變暗 3.6 倍。
Java
import java.awt.image.*;
import java.net.*;
import java.awt.*;
import java.io.*;
import javax.imageio.*;
public class DemonRescaleop {
public static void main(String[] args) throws Exception
{
// picking the image from the url
URL url
= new URL("https:// media.geeksforgeeks.org"
+ "/wp-content/uploads/geeksforgeeks-9.png");
// Reading the image from url
Image image = ImageIO.read(url);
// Setting up the scaling and
// the offset parameters for processing
RescaleOp rop = new RescaleOp(.75f, 3.6f, null);
// applying the parameters on the image
// by using filter() method, it takes the
// Source and destination objects of buffered reader
// here our destination object is null
BufferedImage bi
= rop.filter((BufferedImage)image, null);
ImageIO.write(bi, "png",
new File("processed.png"));
}
}
輸入:

原始圖像
輸出:
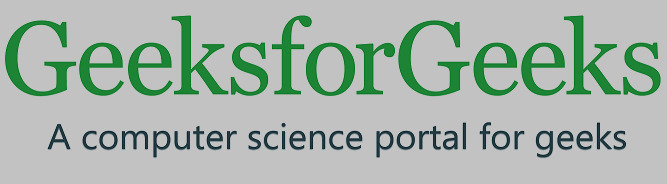
processed.png
使用的方法 filter(BufferedImage src, BufferedImage dst) 將源 BufferedImage 重新縮放到目標 BufferedImage 並返回相同的值。這裏我們沒有提到任何目標圖像並用 null 代替它。在這裏,我們為 BufferedImage 對象分配了重新縮放的結果。示例 2:在給定的示例中,我們將使用比例因子和偏移量數組設置圖片的對比度。每個數組的大小為 3,表示每個像素的紅色、綠色和藍色分量。在以下代碼中,整體亮度增加了 45%,並且所有像素顏色都向綠色移動。偏移量 150 會使每個像素的綠色分量增加 58.6% (150/256)。請記住,偏移量會添加到顏色值中,因此必須是 0 到 255 之間的值,這與比例因子相反,比例因子充當百分比。
Java
import java.awt.image.*;
import java.net.*;
import java.awt.*;
import java.io.*;
import javax.imageio.*;
public class DemonRescaleop {
public static void main(String[] args) throws Exception
{
// picking the image from the URL
URL url
= new URL("https:// media.geeksforgeeks.org"
+ "/wp-content/uploads/geeksforgeeks-9.png");
// Reading the image from url
Image image = ImageIO.read(url);
// Setting up the scaling and
// the offset parameters for processing
float[] factors = new float[] {
// RGB each value for 1 color
1.45f, 1.45f, 1.45f
};
float[] offsets = new float[] {
0.0f, 150.0f, 0.0f
};
RescaleOp rop
= new RescaleOp(factors, offsets, null);
// applying the parameters on the image
// by using filter() method, it takes the
// Source and destination objects of buffered reader
// here our destination object is null
BufferedImage bi
= rop.filter((BufferedImage)image, null);
ImageIO.write(bi, "png",
new File("processed.png"));
}
}
輸入:

原始圖像
輸出:
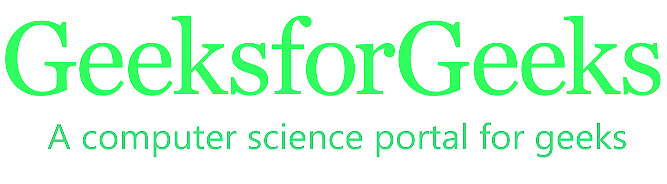
processed.png
使用的方法過濾器(BufferedImage src,BufferedImage dst)將源 BufferedImage 重新調整為目標 BufferedImage 並返回相同的值。這裏我們沒有提到任何目標圖像並用 null 代替它。在這裏,我們為 BufferedImage 對象分配了重新縮放的結果。參考: https://docs.oracle.com/javase/9/docs/api/java/awt/image/RescaleOp.html
相關用法
- Java Java.io.BufferedInputStream.available()用法及代碼示例
- Java Java.io.BufferedInputStream.close()用法及代碼示例
- Java Java.io.BufferedInputStream.read()用法及代碼示例
- Java Java.io.BufferedInputStream.reset()用法及代碼示例
- Java Java.io.BufferedInputStream.skip()用法及代碼示例
- Java Java.io.BufferedOutputStream.flush()用法及代碼示例
- Java Java.io.BufferedOutputStream.Write()用法及代碼示例
- Java Java.io.BufferedReader.Close()用法及代碼示例
- Java Java.io.BufferedReader.mark()用法及代碼示例
- Java Java.io.BufferedReader.markSupported()用法及代碼示例
- Java Java.io.BufferedReader.read()用法及代碼示例
- Java Java.io.BufferedReader.readline()用法及代碼示例
- Java Java.io.BufferedReader.ready()用法及代碼示例
- Java Java.io.BufferedReader.reset()用法及代碼示例
- Java Java.io.BufferedReader.skip()用法及代碼示例
- Java Java.io.BufferedWriter.close()用法及代碼示例
- Java Java.io.BufferedWriter.flush()用法及代碼示例
- Java Java.io.BufferedWriter.newLine()用法及代碼示例
- Java Java.io.BufferedWriter.write()用法及代碼示例
- Java Java.io.ByteArrayInputStream.available()用法及代碼示例
- Java Java.io.ByteArrayInputStream.close()用法及代碼示例
- Java Java.io.ByteArrayInputStream.mark()用法及代碼示例
- Java Java.io.ByteArrayInputStream.read()用法及代碼示例
- Java Java.io.ByteArrayInputStream.reset()用法及代碼示例
- Java Java.io.ByteArrayInputStream.skip()用法及代碼示例
注:本文由純淨天空篩選整理自piyush25pv大神的英文原創作品 Java.awt.image.RescaleOp Class in Java with Examples。非經特殊聲明,原始代碼版權歸原作者所有,本譯文未經允許或授權,請勿轉載或複製。