在本节中,我们将学习 C 编程语言中的 getchar() 函数。 getchar() 函数是一个非标准函数,其含义已在 stdin.h 头文件中定义,以接受来自用户的单个输入。换句话说,是 C 库函数从标准输入中获取单个字符(无符号字符)。但是,getchar() 函数与 getc() 函数类似,但与 C 编程语言的 getchar() 和 getc() 函数之间存在细微差别。 getchar() 从标准输入读取单个字符,而 getc() 从任何输入流读取单个字符。
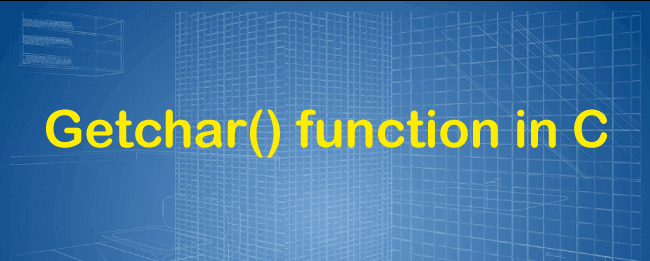
用法
int getchar (void);
它没有任何参数。但是,它将读取的字符作为 int 中的 unsigned char 返回,如果文件有错误,则返回文件末尾的 EOF。
现在我们编写几个getchar()函数程序来接受C中的单个字符并使用putchar()函数打印它们。
使用 getchar() 函数读取单个字符
让我们考虑一个使用 C 语言中的 getchar() 函数来获取单曲的程序。
程序.c
#include <stdio.h>
#include <conio.h>
void main()
{
char c;
printf ("\n Enter a character \n");
c = getchar(); // get a single character
printf(" You have passed ");
putchar(c); // print a single character using putchar
getch();
}
输出
Enter a character A You have passed A
正如我们在上面的程序中看到的那样,它在运行时使用 getchar() 函数从用户那里获取单个字符。得到字符后,通过putchar()函数打印字母。
使用 getchar() 函数从用户读取 n 个字符
让我们考虑使用 C 中的 getchar() 函数读取 n 个字符的程序。
获取字符
#include <stdio.h>
#include <conio.h>
#include <ctype.h>
int main()
{
char ch;
printf (" Enter a character ( If we want to exit press #) \n");
while (ch != '#') /* accept the number till the user does not enter the # to exit from the loop. */
{
ch = getchar();
printf (" \n We have entered the character:");
putchar (ch); // print a single character
printf ("\n");
}
return 0;
}
输出
Enter a character ( If we want to exit.. press #) A We have entered the character:A We have entered the character: B We have entered the character:B We have entered the character: C We have entered the character:C We have entered the character:
正如我们在上面的输出中看到的,while 循环不断地从用户那里接受一个字符,直到用户没有传递 # 字符。这里 getchar() 函数从标准输入中获取单个字符并将它们分配给 ch 变量。而 putchar() 函数打印读取的字符。
使用 scanf() 函数读取单个字符
让我们考虑一个使用 C 中的 scanf() 库函数读取字符的程序。
程序
#include <stdio.h>
#include <conio.h>
int main()
{
char ch;
printf ("\n Enter the character \n");
scanf ("%c", &ch); // get a single character, numeric or words
printf( " You have entered %c", ch); /* It print a single character or first letter of the words. */
return 0;
}
输出
Enter the character A You have entered A
我们可以看到,当我们执行上述程序时,它使用 scanf() 库函数而不是 getchar() 函数来获取单个字符或一组字符。但有一个小的区别; scanf() 函数可以从用户处获取单个或一组字符,而 getchar() 函数只能接受单个字符。
这里我们再次执行上面的程序,这一次,它显示了下面的结果。
Enter the character
Apple
You have entered A
使用 do-while 循环读取字符
让我们考虑使用 C 中的 do while 和 getchar() 函数读取字符的程序。
Dowhile1.c
#include <stdio.h>
#include <ctype.h>
int main()
{
int ch, i = 0;
char str[150];
printf (" Enter the characters from the keyboard (Press Enter button to stop).\n");
// use do while loop to define the condition
do
{
ch = getchar(); // takes character, number, etc from the user
str[i] = ch; // store the ch into str[i]
i++; // increment loop by 1
} while (ch != '\n'); // ch is not equal to '\n'
printf("Entered characters are %s ", str);
return 0;
}
输出
Enter the characters from the keyboard (Press Enter button to stop). Well b47gvb come Entered characters are Well b47gvb come
在上面的程序中,一个 do-while 循环不断地接受字符,直到用户通过 ENTER 按钮退出循环。
相关用法
- C语言 fread()用法及代码示例
- C语言 feof()用法及代码示例
- C语言 imagesize()用法及代码示例
- C语言 getarcoords()用法及代码示例
- C语言 strcspn()用法及代码示例
- C语言 setlinestyle()用法及代码示例
- C语言 showbits()用法及代码示例
- C语言 sprintf()用法及代码示例
- C语言 outtextxy()用法及代码示例
- C语言 isgraph()用法及代码示例
- C语言 grapherrormsg()用法及代码示例
- C语言 moveto()用法及代码示例
- C语言 putchar()用法及代码示例
- C语言 tmpnam()用法及代码示例
- C语言 putpixel()用法及代码示例
- C语言 fillellipse()用法及代码示例
- C语言 outtext()用法及代码示例
- C语言 atan2()用法及代码示例
- C语言 isprint()用法及代码示例
- C语言 getchar()用法及代码示例
注:本文由纯净天空筛选整理自 Getchar() function in C。非经特殊声明,原始代码版权归原作者所有,本译文未经允许或授权,请勿转载或复制。