Python 中的 easyinput 模塊提供了一個簡單的輸入接口,類似於 C++ 中的 cin 流。它支持包括文件在內的多種數據類型,並提供不同數據類型輸入、多行輸入等函數。
安裝
要安裝此模塊,請在終端中鍵入以下命令。
pip install easyinput
使用的函數
- 讀取(*類型,數量=1,文件,as_list):從輸入流返回令牌。
參數:
- type:數據類型列表,每種類型都會返回相應的標記。
- amount:用於將給定類型重複特定次數。
- as_list :如果為 true,則令牌流作為列表返回,否則返回生成器。
- file :文件實體流。
- read_many(*類型,數量=1,文件=_StdIn):從輸入流讀取令牌,除非輸入其他類型的元素。
示例1:read()和read_many()的工作
Python3
from easyinput import read_many, read
a = read(int)
b = read(str)
print("The elements using read : ")
print(a, b)
print("Integer inputs using read many : ")
for num in read_many(int):
print(num)
# reading the string after integers
print(read())
輸出:
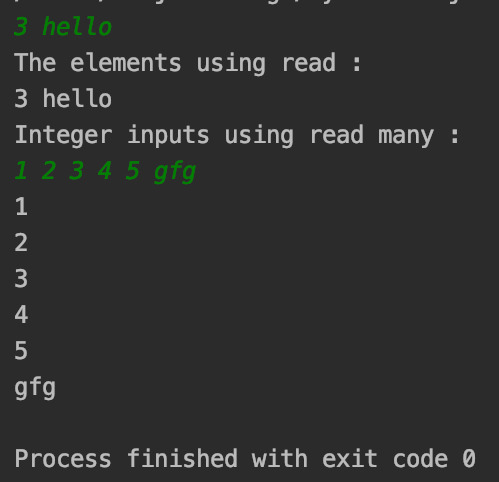
演示read()和read_many()
示例 2:使用amount() 和as_list()
通常,當使用 read() 中的多個參數時,read() 返回一個列表。如果我們需要使用生成器而不是列表,as_list() 可以設置為 false。它的優點是可以避免列表的迭代來訪問和填充不同的數據類型。
Python3
from easyinput import read
# input int, str, int chain 3 times
multi_input = read(int, str, amount=2)
# printing type and input
print(type(multi_input))
print(multi_input)
# putting as_list = False
print("Using as_list false : ")
multi_input = read(int, str, amount=2, as_list=False)
print(type(multi_input))
print(multi_input)
輸出:

使用amount()和as_list()
示例3:使用read_many()輸入文件
read() 和 read_many() 函數提供將文件作為輸入流獲取的函數,以從文件中獲取數據並在控製台上渲染,或使用文件參數渲染到任何文件。
代碼:
Python3
from easyinput import read_many
print("Getting integer inputs from files : ")
with open('gfg_file_input') as inp_file:
for ele in read_many(int, file=inp_file):
print(ele)
輸出:

輸出數量
示例 4:將自定義數據類型與 read() 一起使用
除了原始數據類型之外,read() 還可以接受接受字符串作為輸入的類實例,這些字符串可用於轉換為自定義類型以進行工作。下麵的示例獲取小寫單詞。
Python3
from easyinput import read
class ToLower:
def __init__(self, ele):
self.ele = ele.lower()
def print_ele(self):
print(self.ele)
# Gets object of ToLower class
ele = read(ToLower)
# printing the word
print("Lowercase string : ")
ele.print_ele()
輸出:

將輸入轉換為小寫
使用read_many_lines()
與 read_many() 類似,不同之處在於它一次讀取整行,而不是在空格處移動到換行符。讀取整行。
用法:
read_many_lines(rstrip=真,skip_empty=假)
參數:
rstrip :跳過輸入的所有尾隨換行符。默認值為 True。
skip_empty :默認為 false,當設置為 True 時,會跳過僅是空字符的行。
代碼:
Python3
from easyinput import read_many_lines
print("Reading lines using read many lines : ")
count = 1
for line in read_many_lines():
print(line)
count = count + 1
if count > 5:
break
print("Reading lines using read many lines by skipping empty : ")
count = 1
for sline in read_many_lines(skip_empty=True):
print(sline)
count = count + 1
if count > 5:
break
輸出:

read_many_lines 的示例
相關用法
- Python enumerate()用法及代碼示例
- Python eval()用法及代碼示例
- Python exec()用法及代碼示例
- Python enum.auto()用法及代碼示例
- Python enum.IntEnum用法及代碼示例
- Python enchant.request_dict()用法及代碼示例
- Python enchant.get_enchant_version()用法及代碼示例
- Python enchant.request_pwl_dict()用法及代碼示例
- Python enchant.DictWithPWL()用法及代碼示例
- Python enchant.Dict()用法及代碼示例
- Python enchant.dict_exists()用法及代碼示例
- Python enchant.list_languages()用法及代碼示例
- Python expandtabs()用法及代碼示例
- Python exponential轉float用法及代碼示例
- Python emoji轉text用法及代碼示例
- Python email.message.EmailMessage.add_header用法及代碼示例
- Python email.message.EmailMessage.walk用法及代碼示例
- Python email.message.Message.as_string用法及代碼示例
- Python email.message.Message.as_bytes用法及代碼示例
- Python email.message.Message.add_header用法及代碼示例
- Python email.message.Message.walk用法及代碼示例
- Python email.headerregistry.BaseHeader用法及代碼示例
- Python email.headerregistry.DateHeader用法及代碼示例
- Python email.header.decode_header用法及代碼示例
- Python enumerate用法及代碼示例
注:本文由純淨天空篩選整理自manjeet_04大神的英文原創作品 easyinput module in Python。非經特殊聲明,原始代碼版權歸原作者所有,本譯文未經允許或授權,請勿轉載或複製。