FileInputStream 類從文件係統中的文件中提取輸入字節。 FileInputStream 用於讀取原始字節流,例如圖像數據。要讀取字符流,請考慮使用 FileReader。它應該用於讀取麵向字節的數據,例如讀取音頻、視頻、圖像等。處理輸入流的類的層次結構如下:
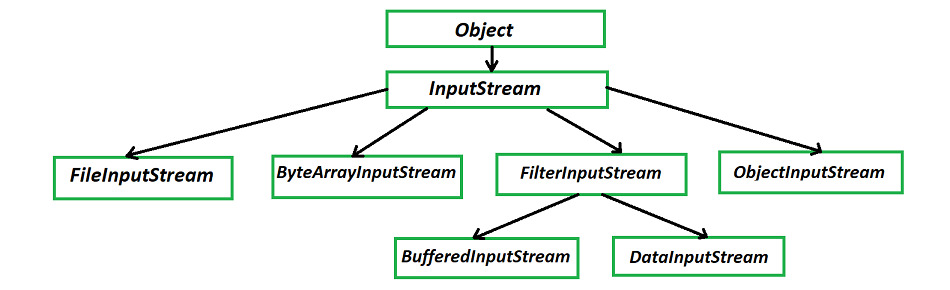
處理輸入流的類的層次結構
示例 1:
Java
// Java program to demonstrate Use of FileInputStream class
// Importing the desired class
import java.io.FileInputStream;
// Importing input output class from java.io package
import java.io.IOException;
// Main class
class FileInputStreamGFG {
// Method 1
// To read from the file
private void readFile() throws IOException
{
// Creating an object of FileInputStream
FileInputStream fileInputStream = null;
// Try block to check for exceptions
try {
// Now, creating a FileInputStream by
// opening a connection to an actual file
// The file named by the path name in the
// file system
// Here customly we have taken
// gfg.txt contains fileInputStream
= new FileInputStream("gfg.txt");
// data - "Java was called
// Oak at the beginning."
int i;
// Reads a byte of data from this input stream
// using read() method
// Till condition holds true
while ((i = fileInputStream.read()) != -1) {
// Print the stream
System.out.print((char)i);
}
}
// If there is any exception encountered
// then execute this block
finally {
// Stream is not there in file
if (fileInputStream != null) {
// Then close this file input stream and
// releases any system resources associated
// with the stream using close() method
fileInputStream.close();
}
}
}
// Method 2
// Main driver method
public static void main(String[] args)
throws IOException
{
// Constructor of this class
FileInputStreamGFG fileInputStreamGfg
= new FileInputStreamGFG();
// Calling the readFile() method
// in the main() method
fileInputStreamGfg.readFile();
}
}
輸出:
Java was called Oak at the beginning.
現在詳細討論 ObjectInputStream 的輸出流,用於反序列化先前使用 ObjectOutputStream 寫入的原始數據和對象。隻有支持 java.io.Externalizable 接口的對象才能從流中讀取。 Java ObjectInputStream 類使您能夠從 InputStream 讀取 Java 對象,而不僅僅是原始字節。您將 InputStream 包裝在 ObjectInputStream 中,以便可以從中讀取對象。當然,讀取的字節必須代表有效的序列化 Java 對象。否則,讀取對象將會失敗。通常我們會使用ObjectInputStream來讀取由Java ObjectOutputStream寫入(序列化)的數據對象。
示例 2:
Java
// Java program to demonstrate Use of ObjectInputStream
// class
// Importing required input output classes
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.Serializable;
// Before serialization and de-serialization of objects the
// class of the object must implement java.io.Serializable
// Class 1
// Helper class implementing Serializable interface
class Student implements Serializable {
// Private class member variables
private static final long serialVersionUID
= -1438960132000208485L;
private String name;
private int age;
// Constructor of this class
public Student(String name, int age)
{
// super keyword refers to parent class
super();
// this keyword refers to current object instance
this.name = name;
this.age = age;
}
// Getters and Setter for class
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public int getAge() { return age; }
public void setAge(int age) { this.age = age; }
// Override toString method
@Override public String toString()
{
// Simply return the name and age
return "Student [name=" + name + ", age=" + age
+ "]";
}
}
// Class 2
// Main class
public class ObjectInputStreamDemo {
// Main driver method
public static void main(String[] args)
throws FileNotFoundException, IOException,
ClassNotFoundException
{
// Creating an object of above class
// in the main() method
ObjectInputStreamDemo objectInputStreamDemo
= new ObjectInputStreamDemo();
// Readfile function call
objectInputStreamDemo.readStudentObject();
}
// Member method of main class
private void readStudentObject()
throws IOException, FileNotFoundException,
ClassNotFoundException
{
// Initially null is set to both streams
// read and write streams
FileInputStream fileInputStream = null;
ObjectInputStream objectInputStream = null;
// Try block to check for exceptions
try {
// Input stream directory
fileInputStream
= new FileInputStream("student.txt");
// Input stream object
objectInputStream
= new ObjectInputStream(fileInputStream);
// Creating an object of above class to
// read an object from the ObjectInputStream
Student student
= (Student)objectInputStream.readObject();
// Display message when input stream is
// completely read
System.out.println(
"Successfully read student object from the file.");
// Print an display commands
System.out.println(student);
System.out.println("Name = "
+ student.getName());
System.out.println("Age = "
+ student.getAge());
}
// When an exception is encountered execute the
// block
finally {
// If there is nothing to be read
if (objectInputStream != null) {
// Then close a ObjectInputStream will
// the InputStream instance from which
// the ObjectInputStream is reading
// isong the close() method
objectInputStream.close();
}
}
}
}
輸出:
Successfully read student object from the file. Student [name=John, age=25] Name = John Age = 25
FileInputStream 和 ObjectInputStream 之間的唯一區別是:
FileInputStream | ObjectInputStream |
---|---|
java.io.FileInputStream 中的 Java FileInputStream 類可以將文件內容作為字節流讀取,因此 FileInputStream 可用於序列化. | Java中的ObjectInputStream可用於將InputStream轉換為對象。這個將輸入流轉換為對象的過程稱為反序列化. |
Java FileInputStream 類從文件獲取輸入字節。 | 它還可用於通過使用 SocketStream 在主機之間傳遞對象。 |
它用於讀取麵向字節的數據。 | 主要用於反序列化使用ObjectOutputStream寫入的原始數據和對象。 |
FileInputStream 類包含 9 個方法。 | ObjectInputStrean 類包含 27 個方法。 |
相關用法
- Java FileInputStream和FileReader的區別用法及代碼示例
- Java FileInputStream available()用法及代碼示例
- Java FileInputStream close()用法及代碼示例
- Java FileInputStream finalize()用法及代碼示例
- Java FileInputStream getChannel()用法及代碼示例
- Java FileInputStream getFD()用法及代碼示例
- Java FileInputStream skip()用法及代碼示例
- Java FileInputStream read()用法及代碼示例
- Java FileDescriptor sync()用法及代碼示例
- Java FileDescriptor valid()用法及代碼示例
- Java FileOutputStream close()用法及代碼示例
- Java FileOutputStream finalize()用法及代碼示例
- Java FileOutputStream getChannel()用法及代碼示例
- Java FileOutputStream getFD()用法及代碼示例
- Java FilePermission equals()用法及代碼示例
- Java FilePermission getActions()用法及代碼示例
- Java FilePermission hashCode()用法及代碼示例
- Java FilePermission implies()用法及代碼示例
- Java FilePermission newPermissionCollection()用法及代碼示例
- Java File canExecute()用法及代碼示例
- Java File canRead()用法及代碼示例
- Java File canWrite()用法及代碼示例
- Java File createNewFile()用法及代碼示例
- Java File createTempFile()用法及代碼示例
- Java File delete()用法及代碼示例
注:本文由純淨天空篩選整理自vanshitatwr620大神的英文原創作品 Difference Between FileInputStream and ObjectInputStream in Java。非經特殊聲明,原始代碼版權歸原作者所有,本譯文未經允許或授權,請勿轉載或複製。